从 C# 中的字符串中删除字符
-
使用 C# 中的
string.Replace()
函数从字符串中删除字符 -
使用 C# 中的
string.Join()
和string.Split()
函数从字符串中删除字符 -
使用 C# 中的
Regex.Replace()
函数从字符串中删除字符 - 在 C# 中使用 Linq 从字符串中删除字符
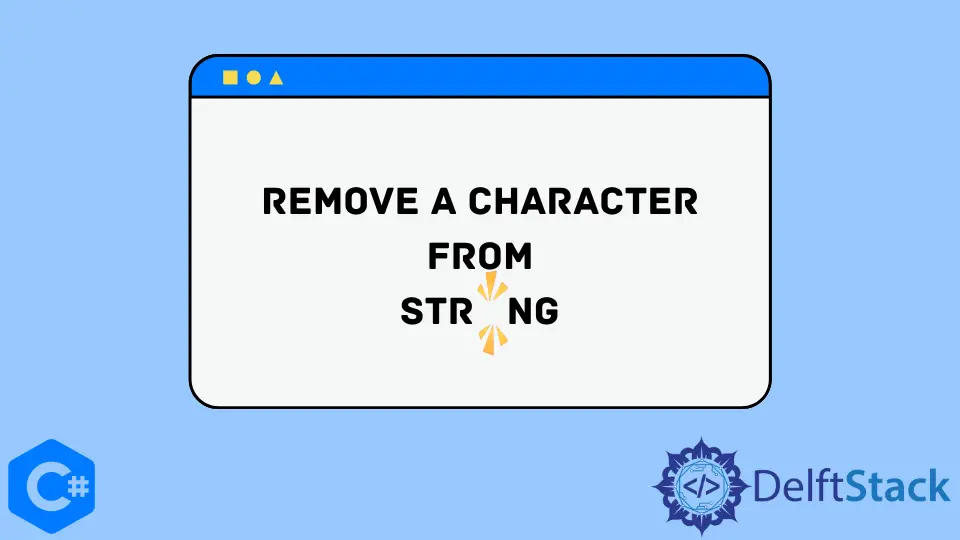
在本教程中,我们将讨论如何从 C# 中的字符串中删除字符。
使用 C# 中的 string.Replace()
函数从字符串中删除字符
string.Replace()
函数可以用来替换列表内的某个字符。我们可以通过将字符替换为 string.Empty
。如果要从字符串中删除多个字符,则必须使用循环来遍历每个字符。以下代码示例向我们展示了如何使用 C# 中的 string.Replace()
函数从字符串中删除多个字符。
using System;
namespace remore_characters_from_string {
class Program {
static void Main(string[] args) {
string str = "Something @to ,Write.;';";
string[] charsToRemove = new string[] { "@", ",", ".", ";", "'" };
foreach (var c in charsToRemove) {
str = str.Replace(c, string.Empty);
}
Console.WriteLine(str);
}
}
}
输出:
Something to Write
我们使用 C# 中的 string.Replace()
函数从字符串 str
中删除了字符 { "@", ",", ".", ";", "'" }
。我们创建了一个数组,其中包含要从 str
字符串中删除的所有字符。为了删除字符,我们遍历数组的每个元素,并使用 C# 中的 string.Replace()
函数将其替换为 string.Empty
。
使用 C# 中的 string.Join()
和 string.Split()
函数从字符串中删除字符
string.Join()
函数用于连接带有指定分隔符的参数中传递的字符串数组。string.Split()
函数用于将字符串拆分为多个具有指定分隔符的字符串。string.Join()
函数可与 string.Split()
函数一起使用,以从字符串中删除字符。这种方法比 string.Replace()
函数更好,因为我们不必使用循环来删除多个字符。以下代码示例向我们展示了如何使用 C# 中的 string.Join()
和 string.Split()
函数从字符串中删除多个字符。
using System;
namespace remore_characters_from_string {
static void Main(string[] args) {
string str = "Something @to ,Write.;';";
str = string.Join("", str.Split('@', ',', '.', ';', '\''));
Console.WriteLine(str);
}
}
}
输出:
Something to Write
我们使用 C# 中的 string.Replace()
函数从字符串 str
中删除了字符 { "@", ",", ".", ";", "'" }
。我们创建了一个数组,其中包含要从 str
字符串中删除的所有字符。为了删除字符,我们遍历数组的每个元素,并使用 C# 中的 string.Replace()
函数将其替换为 string.Empty
。
使用 C# 中的 Regex.Replace()
函数从字符串中删除字符
Regex
(也称为正则表达式)旨在增强功能 C# 中的文本操作。Regex.Replace()
函数用于替换以指定的顺序显示两个文本。通过在 regex 模式参数中指定每个字符,可以用 string.Empty
替换每个字符,从而可以从字符串中删除字符。下面的代码示例向我们展示了如何使用 C# 中的 Regex.Replace()
函数从字符串中删除多个字符。
using System;
using System.Text.RegularExpressions;
namespace remore_characters_from_string {
class Program {
static void Main(string[] args) {
string str = "Something @to ,Write.;';";
str = Regex.Replace(str, "[@,\\.\";'\\\\]", string.Empty);
Console.WriteLine(str);
}
}
}
输出:
Something to Write
我们使用 C# 中的 Regex.Replace()
函数从字符串 str
中删除了字符 { "@", ",", ".", ";", "'" }
。
在 C# 中使用 Linq 从字符串中删除字符
Linq 的语言集成查询在 C# 中提供了类似 SQL 的功能。我们可以使用 Linq 从字符串中删除字符。以下代码示例向我们展示了如何使用 C# 中的 Linq 从字符串中删除多个字符。
using System;
using System.Linq;
using System.Text.RegularExpressions;
namespace remore_characters_from_string {
class Program {
static void Main(string[] args) {
string str = "Something @to ,Write.;';";
str = new string((from c in str
where char.IsWhiteSpace(c) || char.IsLetterOrDigit(c)
select c
).ToArray());
Console.WriteLine(str);
}
}
}
输出:
Something to Write
我们使用 C# 中的 Linq 从字符串 str
中删除了所有非空格,字母或数字的内容。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn相关文章 - Csharp Char
- C# 将字符 char 转换为整型 int
- 在 C# 中将 Char 转换为 Int
- 在 C# 中获取字符串的第一个字符
- 在 C# 中计算字符串中一个字符的出现次数
- 在 C# 中获取字符的 ASCII 值