在 C# 中获取字符串的第一个字符
Muhammad Maisam Abbas
2024年2月16日
Csharp
Csharp String
Csharp Char
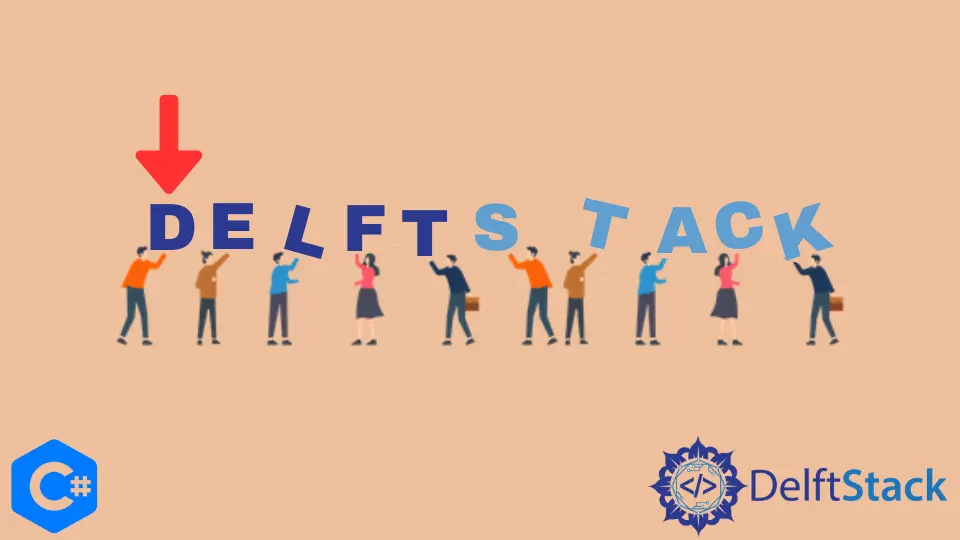
本教程将讨论在 C# 中获取字符串变量的第一个字符的方法。
在 C# 中使用 Linq 方法获取字符串的第一个字符
Linq 用于在 C# 中集成对数据结构的 SQL 查询。FirstOrDefault()
函数获取字符串的第一个字符。以下代码示例向我们展示了如何使用 C# 中的 Linq 方法获取字符串的第一个字符。
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string name = "DelftStack";
char first = name.FirstOrDefault();
Console.WriteLine(first);
}
}
}
输出:
D
在上面的代码中,我们使用 C# 中的 FirstOrDefault()
函数从字符串变量名称
中提取了第一个字符第一个
。
在 C# 中使用 String[]
方法获取字符串的第一个字符
String[x]
属性获取 C# 中字符串的 x
索引上的字符。下面的代码示例向我们展示了如何使用 C# 中的 String[]
属性获取字符串变量的第一个字符。
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string name = "DelftStack";
char first = name[0];
Console.WriteLine(first);
}
}
}
输出:
D
在上面的代码中,我们从字符串变量 name
中以 C# 中的 name[0]
提取了第一个字符 first
。
在 C# 中使用用户定义的方法获取字符串的第一个字符
通过遵循 C# 中用户定义的方法,我们还可以获得字符串的第一个字符。为此,我们必须首先使用 C# 中的 String.ToCharArray()
函数将字符串转换为字符数组。之后,我们可以在字符数组的 0
索引处获得该字符。以下代码示例向我们展示了如何在 C# 中实现此逻辑。
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string name = "DelftStack";
char[] charArray = name.ToCharArray();
char first = charArray[0];
Console.WriteLine(first);
}
}
}
输出:
D
此代码与上面讨论的其他两个示例具有相同的作用。但是不建议使用这种方法,因为它需要花费更多的时间和资源来执行,而且已经有一个内置的方法来实现。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn相关文章 - Csharp String
- C# 将字符串转换为枚举类型
- C# 中将整形 Int 转换为字符串 String
- 在 C# 中的 Switch 语句中使用字符串
- 如何在 C# 中把一个字符串转换为布尔值
- 如何在 C# 中把一个字符串转换为浮点数
- 如何在 C# 中将字符串转换为字节数组