在 C# 中将 Char 转换为 Int
Abdullahi Salawudeen
2023年10月12日
Csharp
Csharp Char
Csharp Int
-
C#
中的char
数据类型 -
在
C#
中使用Char.GetNumericValue()
方法将char
转换为int
-
在
C#
中使用Convert.ToInt32()
方法将char
转换为int
-
在
C#
中使用Int32.Parse()
方法将char
转换为int
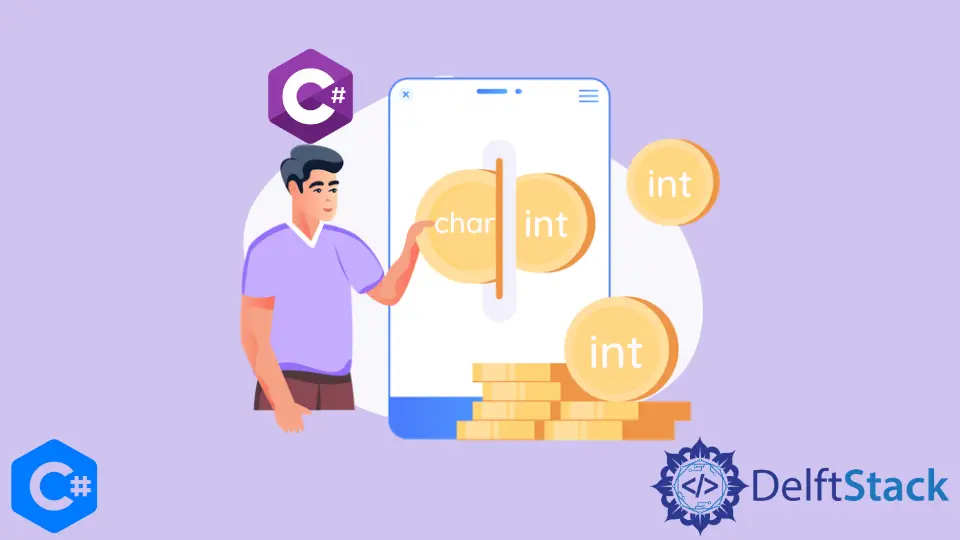
C# 是一种面向对象的编程语言。这意味着必须声明每个变量,同时指示变量将存储的值的类型。
C# 变量以不同的形式或类型存储,称为数据类型
。
C#
中的 char
数据类型
声明具有 Char
数据类型的变量的示例语法。
type variableName = value;
char grade = 'A';
char myCharacter = 'X';
char myLuckyNumber = '3';
单个字符存储在 char
数据类型中。字符必须用单引号括起来,例如 'A'
或 'X'
。
在 C#
中使用 Char.GetNumericValue()
方法将 char
转换为 int
Char.GetNumericValue()
方法将指定的数字 Unicode 字符转换为双精度浮点数。
如果该方法应用于数值用单引号括起来的 char
变量,则返回该数字,否则返回 -1
。
代码片段:
using System;
public class GetNumericValue {
public static void Main() {
int result = (int)Char.GetNumericValue('8');
Console.WriteLine(result); // Output: "8"
Console.WriteLine(Char.GetNumericValue('X')); // Output: "-1"
}
}
输出:
8-1
在 C#
中使用 Convert.ToInt32()
方法将 char
转换为 int
Convert.ToInt32()
函数将指定值转换为 32 位有符号整数。ToInt32()
方法有很多变体,具体取决于传递的参数的数据类型。
代码片段:
using System;
public class ConvertCharToInt {
public static void Main() {
char c = '3';
Console.WriteLine(Convert.ToInt32(c.ToString())); // Output: "3"
Console.WriteLine(Convert.ToInt32(c)); // Output: "51"
}
}
输出:
351
如果 char
数据类型作为参数传递给 Convert.Tolnt32()
方法,则返回 ASCII
等效项。
在 C#
中使用 Int32.Parse()
方法将 char
转换为 int
Int32.Parse()
方法将数字的 string
表示转换为其等效的 32 位有符号整数。此方法的缺点是必须将数值作为 string
参数传递。
char
数据类型必须首先转换为 string
数据类型,并且必须包含单引号 ' '
中的数值,否则代码将引发 溢出异常
。
代码片段:
using System;
public class GetNumericValue {
public static void Main() {
char c = '3';
char s = 'x';
string str = c.ToString();
string ex = s.ToString();
Console.WriteLine(Int32.Parse(str));
// Console.WriteLine(Int32.Parse(ex)); // Output: "ThrowEx"
}
}
输出:
3
如果 char
值不是单引号 ' '
中的数字,则会引发异常,如下所示。
代码片段:
using System;
public class GetNumericValue {
public static void Main() {
char s = 'x';
string ex = s.ToString();
Console.WriteLine(Int32.Parse(ex));
}
}
输出:
Unhandled Exception:
System.FormatException: Input string was not in a correct format.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe