在 C# 中计算字符串中一个字符的出现次数
- 使用 C# 中的 Linq 方法计算字符串中字符的出现次数
-
使用 C# 中的
String.Split()
方法计算字符串中字符的出现次数 -
使用 C# 中的
foreach
循环来计算字符串中字符的出现次数
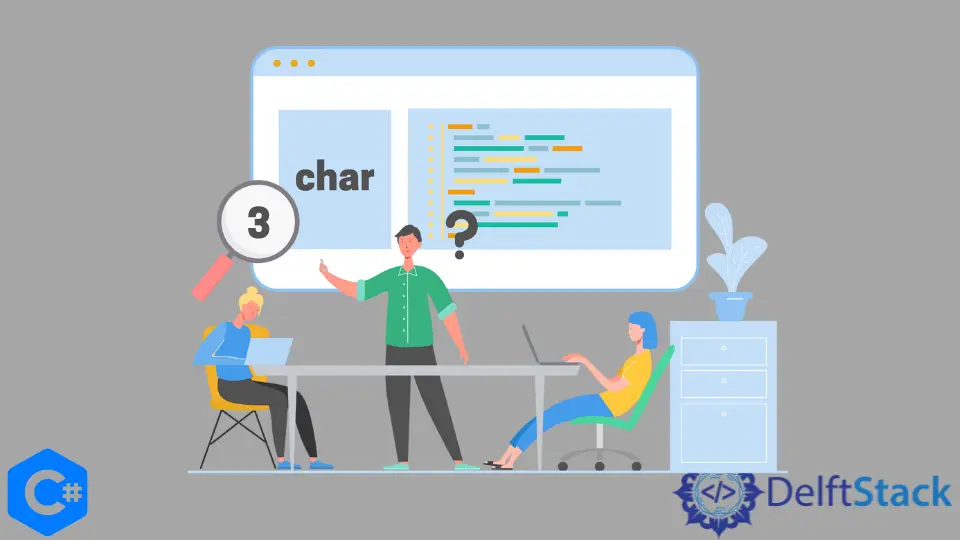
本教程将介绍在 C# 中获取字符串变量中字符出现次数的方法。
使用 C# 中的 Linq 方法计算字符串中字符的出现次数
Linq
在 C# 中的数据结构上集成了 SQL 功能。下面的代码示例向我们展示了如何使用 C# 中的 Linq 方法获取字符串中某个字符的出现次数。
using System;
using System.Linq;
namespace count_occurrences_of_a_char_in_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = source.Count(f => f == 'o');
Console.WriteLine(count);
}
}
}
输出:
2
在上面的代码中,我们使用 C# 中的 Linq 方法计算了字符串变量 source
中字符 o
的出现次数。
使用 C# 中的 String.Split()
方法计算字符串中字符的出现次数
String.Split()
方法基于 C# 中的分隔符将字符串拆分为多个子字符串。String.Split(x)
方法将比字符串中 x
的出现次数多返回 1 个字符串。我们可以计算 String.Split()
方法返回的字符串数,并从中减去 1,以获取主字符串中字符出现的次数。下面的代码示例向我们展示了如何使用 C# 中的 String.Split()
方法来计算字符串变量中字符出现的次数。
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = source.Split('o').Length - 1;
Console.WriteLine(count);
}
}
}
输出:
2
在上面的代码中,我们使用 C# 中的 String.Split()
函数计算了字符串变量 source
中字符 o
的出现次数。
使用 C# 中的 foreach
循环来计算字符串中字符的出现次数
foreach
循环用于遍历 C# 中的数据结构。我们可以使用 foreach
循环遍历字符串变量的每个字符,并使用 C# 中的 if
语句检查该字符是否与所需字符匹配。下面的代码示例向我们展示了如何使用 C# 中的 foreach
循环来计算字符串中字符的出现次数。
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = 0;
foreach (char c in source) {
if (c == 'o') {
count++;
}
}
Console.WriteLine(count);
}
}
}
输出:
2
在上面的代码中,我们使用 C# 中的 foreach
循环计算了字符串变量 source
中字符 o
的出现次数。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn相关文章 - Csharp String
- C# 将字符串转换为枚举类型
- C# 中将整形 Int 转换为字符串 String
- 在 C# 中的 Switch 语句中使用字符串
- 如何在 C# 中把一个字符串转换为布尔值
- 如何在 C# 中把一个字符串转换为浮点数
- 如何在 C# 中将字符串转换为字节数组