在 C# 中計算字串中一個字元的出現次數
- 使用 C# 中的 Linq 方法計算字串中字元的出現次數
-
使用 C# 中的
String.Split()
方法計算字串中字元的出現次數 -
使用 C# 中的
foreach
迴圈來計算字串中字元的出現次數
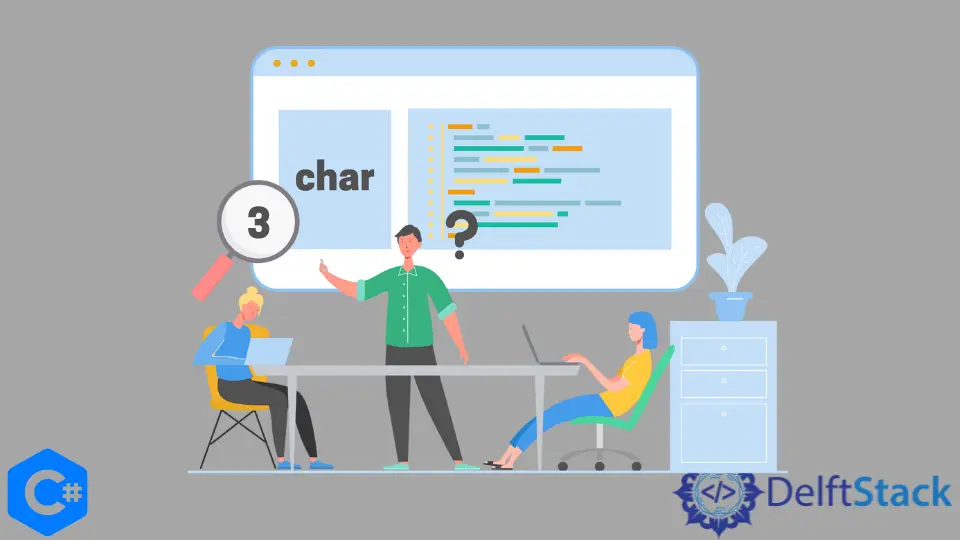
本教程將介紹在 C# 中獲取字串變數中字元出現次數的方法。
使用 C# 中的 Linq 方法計算字串中字元的出現次數
Linq
在 C# 中的資料結構上整合了 SQL 功能。下面的程式碼示例向我們展示瞭如何使用 C# 中的 Linq 方法獲取字串中某個字元的出現次數。
using System;
using System.Linq;
namespace count_occurrences_of_a_char_in_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = source.Count(f => f == 'o');
Console.WriteLine(count);
}
}
}
輸出:
2
在上面的程式碼中,我們使用 C# 中的 Linq 方法計算了字串變數 source
中字元 o
的出現次數。
使用 C# 中的 String.Split()
方法計算字串中字元的出現次數
String.Split()
方法基於 C# 中的分隔符將字串拆分為多個子字串。String.Split(x)
方法將比字串中 x
的出現次數多返回 1 個字串。我們可以計算 String.Split()
方法返回的字串數,並從中減去 1,以獲取主字串中字元出現的次數。下面的程式碼示例向我們展示瞭如何使用 C# 中的 String.Split()
方法來計算字串變數中字元出現的次數。
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = source.Split('o').Length - 1;
Console.WriteLine(count);
}
}
}
輸出:
2
在上面的程式碼中,我們使用 C# 中的 String.Split()
函式計算了字串變數 source
中字元 o
的出現次數。
使用 C# 中的 foreach
迴圈來計算字串中字元的出現次數
foreach
迴圈用於遍歷 C# 中的資料結構。我們可以使用 foreach
迴圈遍歷字串變數的每個字元,並使用 C# 中的 if
語句檢查該字元是否與所需字元匹配。下面的程式碼示例向我們展示瞭如何使用 C# 中的 foreach
迴圈來計算字串中字元的出現次數。
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = 0;
foreach (char c in source) {
if (c == 'o') {
count++;
}
}
Console.WriteLine(count);
}
}
}
輸出:
2
在上面的程式碼中,我們使用 C# 中的 foreach
迴圈計算了字串變數 source
中字元 o
的出現次數。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn相關文章 - Csharp String
- C# 將字串轉換為列舉型別
- C# 中將整形 Int 轉換為字串 String
- 在 C# 中的 Switch 語句中使用字串
- 如何在 C# 中把一個字串轉換為布林值
- 如何在 C# 中把一個字串轉換為浮點數
- 如何在 C# 中將字串轉換為位元組陣列