How to Get the First Character of a String in C#
-
Get the First Character of a String Variable in C# Using LINQ’s
First()
-
Get the First Character of a String Variable in C# Using LINQ’s
FirstOrDefault()
-
Get the First Character of a String With the
String[x]
Property in C# -
Get the First Character of a String With a User-Defined Method in
C#
-
Get the First Character of a String Variable in C# Using the
ElementAt()
Method - Conclusion
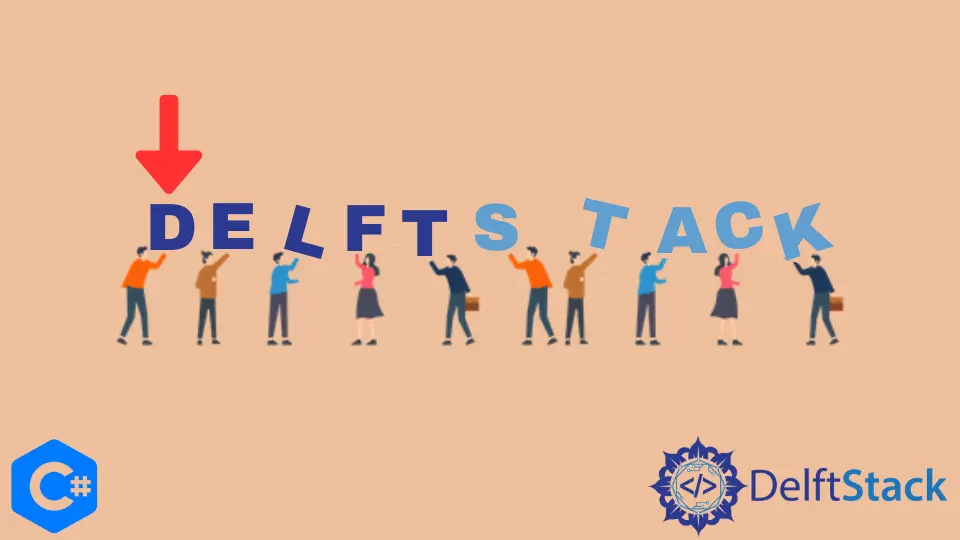
In C#, extracting the first character from a string can be achieved through various methods, each offering its own set of advantages and considerations.
In this article, we will explore multiple approaches, ranging from the convenient LINQ methods like First()
and FirstOrDefault()
to the direct use of the String[x]
property and user-defined methods. Additionally, we delve into the application of the ElementAt()
method in LINQ for direct access to characters at specific indices within a string.
Get the First Character of a String Variable in C# Using LINQ’s First()
In C#, LINQ (Language Integrated Query) provides a powerful and expressive way to perform queries on various data sources, including strings. While LINQ is commonly associated with querying collections, it can also be leveraged to extract specific elements from strings.
LINQ provides a convenient method called First()
that returns the first element of a sequence. Since strings in C# can be treated as sequences of characters, this method can be applied to extract the initial character.
Below is an example demonstrating how to utilize LINQ’s First()
function for this purpose:
using System;
using System.Linq;
class Program
{
static void Main()
{
string inputString = "DelftStack";
char firstChar = inputString.First();
Console.WriteLine($"The first character is: {firstChar}");
}
}
Output:
The first character is: D
In the provided code snippet, LINQ’s First()
function is applied directly to the string variable text
. This function retrieves the first character of the string.
The string is treated as a sequence of characters, and First()
efficiently extracts the initial character.
It’s important to note that if the string is empty, calling First()
will result in an InvalidOperationException
. Therefore, it’s advisable to ensure the string is not empty before using this method.
Get the First Character of a String Variable in C# Using LINQ’s FirstOrDefault()
Another function in LINQ, FirstOrDefault()
, is designed to return the first element of a sequence or a default value if the sequence is empty. While commonly used with collections, it can also be applied to strings, treating them as sequences of characters.
Let’s see a practical example demonstrating how to leverage LINQ’s FirstOrDefault()
to extract the first character of a string:
using System;
using System.Linq;
namespace ExtractFirstCharWithLINQ {
class Program {
static void Main(string[] args) {
string text = "DelftStack";
char firstChar = text.FirstOrDefault();
Console.WriteLine($"The first character is: {firstChar}");
}
}
}
Output:
The first character is: D
In the provided code snippet, we have the line:
char firstChar = text.FirstOrDefault();
LINQ’s FirstOrDefault()
function is applied directly to the string variable text
. This function retrieves the first character of the string or a default value if the string is empty.
The FirstOrDefault()
function returns a char
value, and there’s an implicit conversion from char?
(nullable char) to char
when assigned to firstChar
. This conversion is safe because we are confident the string is not empty.
In cases where the string is empty, FirstOrDefault()
will return the default value for char
, which is the null character ('\0'
). It’s essential to consider this behavior when using this method.
Get the First Character of a String With the String[x]
Property in C#
The String[x]
property allows developers to access individual characters within a string by specifying the index x
. In C#, strings are zero-based indexed, meaning the first character is at index 0
, the second character is at index 1
, and so on.
Let’s dive into a practical example to illustrate how the String[]
property can be employed to extract the first character of a string variable:
using System;
namespace ExtractFirstChar {
class Program {
static void Main(string[] args) {
string name = "DelftStack";
char firstChar = name[0];
Console.WriteLine($"The first character is: {firstChar}");
}
}
}
Output:
The first character is: D
In the provided code snippet above:
char firstChar = name[0];
The use of name[0]
signifies accessing the character at the first index of the string variable name
. Since indexing is zero-based in C#, this operation retrieves the first character.
The String[]
property allows for direct access to individual characters in the string. This directness simplifies the code, providing an efficient means to extract characters at specific positions.
Using String[]
for extracting the first character keeps the code concise and readable. This approach is particularly beneficial when only the initial character is required, eliminating the need for additional methods or complex operations.
Get the First Character of a String With a User-Defined Method in C#
In C#, there are various approaches to obtaining the first character of a string variable. One such method involves a user-defined approach, which includes converting the string to an array of characters using the String.ToCharArray()
function and then accessing the character at the 0
index of the resulting array.
While this method achieves the desired result, it’s important to note that it may not be the most efficient option due to the additional conversion step. Let’s explore this user-defined method through a practical example:
using System;
namespace GetUserDefinedFirstChar {
class Program {
static void Main(string[] args) {
string name = "DelftStack";
char firstChar = GetFirstCharacter(name);
Console.WriteLine($"The first character is: {firstChar}");
}
static char GetFirstCharacter(string input) {
char[] charArray = input.ToCharArray();
char firstChar = charArray[0];
return firstChar;
}
}
}
Output:
The first character is: D
In the above code snippet, you can see the line below:
char firstChar = GetFirstCharacter(name);
Here, the GetFirstCharacter
method is created to encapsulate the logic of extracting the first character of a string. Within the user-defined method, the string is converted to an array of characters using the ToCharArray()
function.
The character at the 0
index of the character array is then accessed, representing the first character of the original string. Finally, the extracted character is returned from the user-defined method.
While this user-defined method achieves the desired outcome, it’s essential to consider that this approach involves an additional step of converting the string to a character array, potentially introducing unnecessary overhead. In scenarios where performance is critical, alternative methods may be more efficient.
C# provides built-in methods, such as using the String[x]
property or LINQ’s FirstOrDefault()
, which offer a more direct and concise way to extract the first character without the need for additional conversions.
Get the First Character of a String Variable in C# Using the ElementAt()
Method
The ElementAt
method in C# is also a part of the LINQ (Language Integrated Query) extension methods provided by the System.Linq
namespace. It enables direct access to the element at a specified index within a sequence.
While ElementAt
is commonly used with collections, we can also apply it to strings, treating them as sequences of characters.
Let’s have an example demonstrating how to use the ElementAt()
method to get the first character of a string:
using System;
using System.Linq;
namespace ExtractFirstCharWithElementAt {
class Program {
static void Main(string[] args) {
string text = "CSharp DelftStack";
char firstChar = text.ElementAt(0);
Console.WriteLine($"The first character is: {firstChar}");
}
}
}
Output:
The first character is: C
In the provided code snippet, the ElementAt
method is applied directly to the string variable text
. This allows for direct access to the character at the specified index (0
in this case).
As with most indexing in C#, the index passed to ElementAt
is zero-based. Therefore, ElementAt(0)
retrieves the first character of the string.
However, be cautious because if the index provided to ElementAt
is out of range (less than 0
or greater than or equal to the length of the string), an ArgumentOutOfRangeException
is thrown. It’s essential to ensure the index is within a valid range.
Conclusion
We have explored various methods to extract the first character of a string variable in C#. We began by introducing LINQ’s First()
and FirstOrDefault()
functions, showcasing their application in treating strings as sequences of characters.
The String[x]
property was then presented as a direct and concise means to access the first character. We also delved into a user-defined method involving the conversion of the string to a character array.
Additionally, we discussed the usage of the ElementAt()
method from the LINQ extension methods, emphasizing its applicability to retrieve the first character directly from a string.
Each method demonstrated in this article has its merits and considerations. The choice of which method to use depends on factors such as code readability, performance requirements, and the presence of edge cases (e.g., empty strings).
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#