How to Convert String to Enum in C#
-
C#
string
toenum
Conversion - UsingEnum.Parse()
andEnum.TryParse()
Methods -
C# Program to Convert
string
Into anenum
With Exception Handling UsingEnum.Parse()
Method -
C# Program to Convert
string
Into anenum
UsingEnum.TryParse()
-
C# Program to Check String Value Existence Using
Enum.IsDefined()
Method - Conclusion
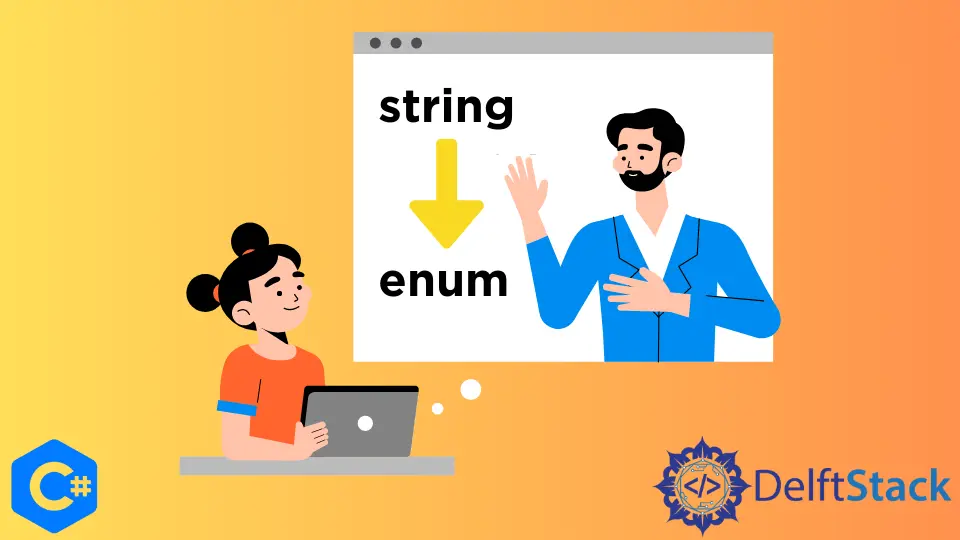
In C# we use Enum.Parse()
and Enum.TryParse()
methods to convert a string into an enum. Here we have given examples for both, but we recommend you to use Enum.TryParse()
method when converting a string into an enum.
C# string
to enum
Conversion - Using Enum.Parse()
and Enum.TryParse()
Methods
In order to convert a string into an enum
, we can use Enum.Parse()
method. You will have to carefully include System
at the top of your file as Enum.Parse()
is a static method.
Enum type, the string value and an indicator(optional) are the parameters of this method. The correct syntax to use Enum.Parse()
and Enum.TryParse()
is following:
EnumName VariableName = (EnumName)Enum.Parse(typeof(EnumName), StringValue);
EnumName VariableName = (EnumName)Enum.Parse(typeof(EnumName), StringValue,
indicator); // indicator can be true or false
// To implement Enum.TryParse()
EnumName VariableName;
Enum.TryParse(StringValue, out VariableName);
Now we are going to implement this in a c# program.
C# Program to Convert string
Into an enum
Using Enum.Parse()
using System;
class Conversion {
enum Flowers { None, Daisy = 1, Lili = 2, Rose = 3 }
static void Main() {
string stringvalue = "Rose";
Flowers Flower = (Flowers)Enum.Parse(typeof(Flowers), stringvalue);
// To check if we have converted successfully
if (Flower == Flowers.Rose) {
Console.WriteLine("The Flower is Rose");
}
}
}
Output:
The Flower is Rose
Here we have an enum named as Flowers
containing multiple constants with their respective values. We have called Enum.Parse()
method to convert the string value Rose
into an enum. The typeof(Flowers)
will return the enum type. In the end, we have checked if our conversion was successful or not.
Sometimes, an exception may occur if the string that we are trying to convert is not available in the enum. To avoid this we will use try-catch
block.
C# Program to Convert string
Into an enum
With Exception Handling Using Enum.Parse()
Method
using System;
class Conversion {
enum Flowers { None, Daisy = 1, Lili = 2, Rose = 3 }
static void Main() {
stringvalue = "Jasmine";
try {
Flowers Flower = (Flowers)Enum.Parse(typeof(Flowers), stringvalue);
} catch (Exception ex) {
Console.WriteLine("Not Possible");
// Set value to none
Flower = Flowers.none;
}
}
}
Output:
Not Possible
C# Program to Convert string
Into an enum
Using Enum.TryParse()
using System;
enum Flowers { None, Daisy = 1, Lili = 2, Rose = 3 }
class Conversion {
static void Main() {
string stringvalue = "Rose";
// Using Enum.TryParse()
Flowers Flower;
if (Enum.TryParse(stringvalue, out Flower)) {
Console.WriteLine(Flower == Flowers.Rose);
}
}
}
Output:
True
We can also pass the value of constant in enum as a parameter in Enum.TryParse()
method. Following program implements this:
using System;
enum Flowers { None, Daisy = 1, Lili = 2, Rose = 3 }
class Conversion {
static void Main() {
Flowers Flower;
if (Enum.TryParse("3", out Flower)) {
Console.WriteLine(Flower);
}
}
}
Output:
Rose
You can also check either the string value that you want to convert is defined in enum or not using Enum.IsDefined()
method.
C# Program to Check String Value Existence Using Enum.IsDefined()
Method
using System;
enum Flowers { None, Daisy = 1, Lili = 2, Rose = 3 }
class Conversion {
static void Main() {
Console.WriteLine(Enum.IsDefined(typeof(Flowers), "Rose"));
}
}
Output:
True
Conclusion
Enum.Parse()
and Enum.TryParse()
are the methods that are used to convert a string into an enum. But it is the best practice to use Enum.TryParse()
.
Related Article - Csharp String
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#