How to Get Int Value From Enum in C#
-
Type Cast to Convert
enum
toint
-
static
Class to Convertenum
toint
-
Use
GetTypeCode()
to Convertenum
toint
- More Examples
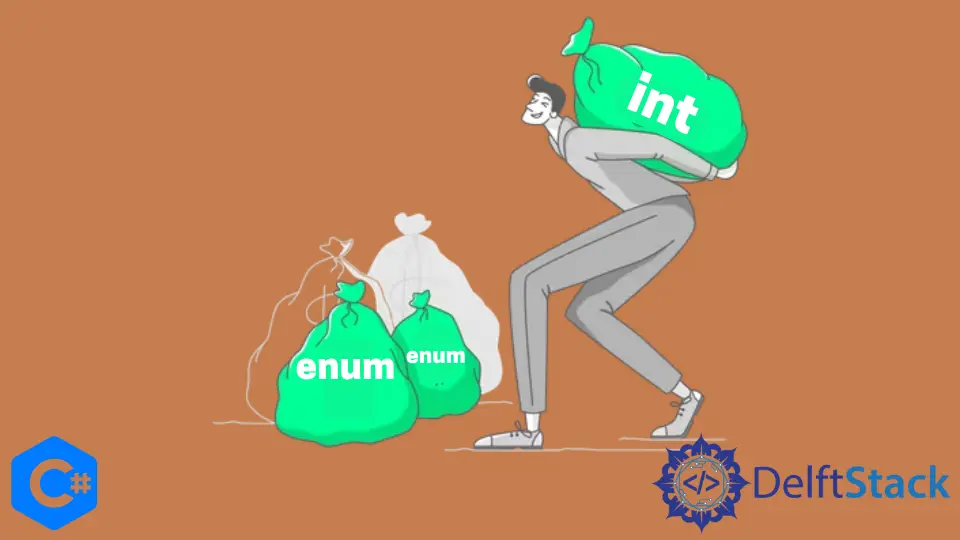
This tutorial explains how to get int
value from enum
in C# and lists down some of the common code examples to gather an overview of C#’s enumeration type.
Enum
(also known as Enumeration
) is a user-defined datatype or a value type that usually defines a set of constant named values of the underlying integral type. enum
keyword defines an enumeration type within the code. The basic example is given below:
enum Name { Joe, Rick, John, Matt }
The enumeration type of the predefined constant values is of data type int
by default. However, the underlying integral type can be explicitly set to any other integral numeric type like sbyte
, byte
, short
, ushort
, int
, uint
, long
and ulong
. The below table describes the size and range of each predefined integral type in C#.
C# keyword | Integer Size | Range |
---|---|---|
sbyte |
signed 8-bit |
[-128, 127] |
byte |
unsigned 8-bit |
[0, 255] |
short |
signed 16-bit |
[-32,768, 32,767] |
ushort |
unsigned 16-bit |
[0, 65,535] |
int |
signed 32-bit |
[-2,147,483,648, 2,147,483,647] |
uint |
unsigned 32-bit |
[0, 4,294,967,295] |
long |
signed 64-bit |
[-9,223,372,036,854,775,808, 9,223,372,036,854,775,807] |
ulong |
unsigned 64-bit |
[0, 18,446,744,073,709,551,615] |
Type Cast to Convert enum
to int
Getting int
value from enum
is not as generic as it seems to be as the challenge lies within the data types. See the following example.
public enum Days { Numbers = 20, DayType = 3 }
int abc = (int)Days.Numbers;
Console.WriteLine(abc);
Output:
20
There can be different underlying types for enum
which can be a bit tricky for the programmers. The above code stores int
value of Days
enum
to abc
. In this case, the Typecasting method is used to change the data type of an entity to another data type, ensuring correct usage of the variables. But using typecasting is always not preferred.
There are ways around which doesn’t have any dependency on Typecasting and can be pretty straightforward. These ways around are explained in detail with below code examples.
static
Class to Convert enum
to int
using System;
class Demo {
public static class Subjects {
public const int maths = 2;
public const int science = 3;
public const int english = 4;
public const int history = 5;
}
public static void Main() {
int xyz = Subjects.science;
Console.WriteLine(xyz);
}
}
Output:
3
The above code shows that Subjects.science
gets the right value of 3
without thinking about typecasting the correct integer value.
Use GetTypeCode()
to Convert enum
to int
using System;
class Demo {
public enum Subjects { maths, science, english, history }
public static void Main() {
Subjects Maths = Subjects.maths;
Object sub = Convert.GetTypeCode(Maths);
Console.WriteLine(sub);
}
}
Output:
Int32
If any programmer doesn’t want to use static const
, then they can use the above method of GetTypeCode()
. All of the above code successfully extract the integer from the enum type. The examples below will assist in getting more ideas.
More Examples
Example 1:
using System;
class Demo {
public enum Positions { Role = 2, Project = 3, Jobs = 4 }
public static void Main() {
int a = (int)Positions.Jobs;
Console.WriteLine(a);
}
}
Output:
4
Example 2:
using System;
class Demo {
public enum Galaxy : long { Sun = 1, S5HVS1 = 2, HD140283 = 2147977653 }
;
public static void Main() {
long solar = (long)Galaxy.HD140283;
Console.WriteLine(solar);
}
}
Output:
2147977653
Related Article - Csharp Integer
- How to Convert Int to String in C#
- How to Convert Int to Enum in C#
- Random Int in C#
- Random Number in a Range in C#
- How to Convert String to Int in C#
- Integer Division in C#