Integer Division in C#
- Implement Integer Division in C#
- Enhancing Accuracy in Integer Division With Float Casting in C#
-
Integer Division and Remainder Calculation With
%
in C# - Conclusion
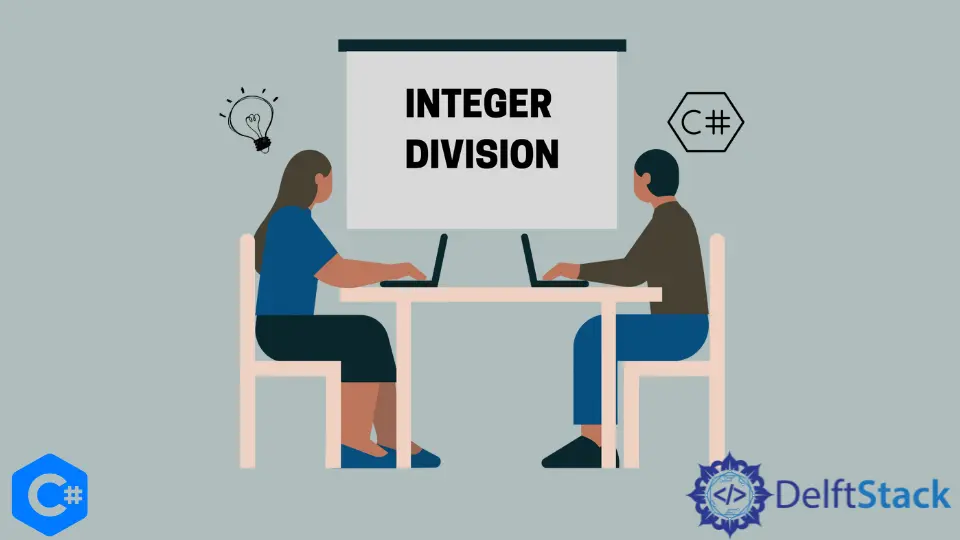
In the landscape of C# programming, understanding integer division is fundamental. When dividing one integer variable by another in C#, the result is another integer, even if the division is not exact.
This property is central to C# arithmetic, and this article explores its application using the /
operator, demonstrating the behavior through a concise code snippet. This tutorial will discuss the methods to perform integer division in C#.
Implement Integer Division in C#
Integer division is a fundamental property of C#. If we divide an integer variable with another integer variable, it returns another integer variable.
This property holds even when the numerator isn’t completely divisible by the denominator. This property of division in C# is demonstrated in the following code snippet.
using System;
class Program {
static void Main() {
// Assume we have two integers for division
int numerator = 20;
int denominator = 7;
// Applying the / operator for integer division
int result = numerator / denominator;
// Displaying the result to the console
Console.WriteLine($"Result of integer division: {result}");
}
}
Imagine we have a scenario where we need to divide two integers, numerator
and denominator
. In our example, numerator
is set to 20
, and denominator
is set to 7
.
Now, the crux of integer division lies in the line:
Here, the /
operator is employed for division. When applied to integers, it performs what’s known as integer division.
This means that any remainder resulting from the division is disregarded, and only the quotient, the whole number part, is retained. The outcome is stored in the variable result
.
To make this concept more tangible, let’s consider the values we’ve chosen: 20
as the numerator and 7
as the denominator. When we execute this division, the result will be 2
because 20
divided by 7
equals 2
with a remainder of 6
(20 = 2 * 7 + 6
).
Finally, we use Console.WriteLine
to present the result to the console.
Output:
Result of integer division: 2
Enhancing Accuracy in Integer Division With Float Casting in C#
There are scenarios where obtaining more accurate, floating-point results is imperative. In this discussion, we’ll explore a technique that involves casting either the numerator or the denominator to a float
to achieve this enhanced precision in integer division.
Consider a common situation where you have two integers, numerator
and denominator
, representing values that need to be divided. In many cases, the straightforward use of the /
operator results in integer division, discarding any remainder.
While this behavior is suitable for certain scenarios, there are instances where you require a more precise, floating-point output. Let’s dive into a practical example to elucidate this concept.
using System;
class Program {
static void Main() {
// Assume we have two integers for division
int numerator = 15;
int denominator = 4;
// Casting numerator to float for float output
float result1 = (float)numerator / denominator;
// Casting denominator to float for float output
float result2 = numerator / (float)denominator;
// Displaying the results to the console
Console.WriteLine($"Result 1 (casting numerator to float): {result1}");
Console.WriteLine($"Result 2 (casting denominator to float): {result2}");
}
}
Here, the essence lies in strategically casting either the numerator or the denominator to a float
before performing the division. This casting ensures that the division operation involves at least one floating-point operand, leading to a more precise floating-point output.
In the first case, we cast the numerator
to a float
. This guarantees that the division operation considers floating-point arithmetic, resulting in a more accurate output.
Similarly, in the second case, we cast the denominator
to a float
. Once again, this ensures that the division involves at least one floating-point operand, thus improving precision.
The results are then displayed to the console using Console.WriteLine
. By adopting this technique, you gain the flexibility to achieve precise floating-point outputs from integer division in C#.
Output:
Result 1 (casting numerator to float): 3.75
Result 2 (casting denominator to float): 3.75
Integer Division and Remainder Calculation With %
in C#
The %
operator, also known as the modulus operator, plays a key role in this context. It not only provides the quotient of the division but also yields the remainder, offering a versatile tool for various programming scenarios.
Let’s delve into a practical example to illustrate the application of the %
operator in integer division and remainder calculation.
using System;
class Program {
static void Main() {
// Assume we have two integers for division
int numerator = 17;
int denominator = 5;
// Performing integer division using / operator
int quotient = numerator / denominator;
// Calculating the remainder using % operator
int remainder = numerator % denominator;
// Displaying the results to the console
Console.WriteLine($"Quotient of integer division: {quotient}");
Console.WriteLine($"Remainder of integer division: {remainder}");
}
}
Consider a scenario where you have two integers, numerator
and denominator
, set to 17
and 5
, respectively.
We use the /
operator for integer division, resulting in the quotient. The result is the whole number part of the division, disregarding any remainder.
Now, to calculate the remainder, the %
operator comes into play. It calculates the remainder of the division and assigns it to the remainder
variable.
This remainder is essentially what is left after performing the integer division.
The results are then displayed to the console using Console.WriteLine
.
Quotient of integer division: 3
Remainder of integer division: 2
Conclusion
Mastering integer division in C# is essential for precise numeric operations. The /
operator, as showcased in the code snippet, efficiently handles integer division, providing a clear quotient while discarding any remainder.
This foundational concept sets the stage for further exploration of advanced techniques, such as float casting for enhanced precision and the use of the %
operator for both quotient and remainder in integer division. As you navigate through these methods, you empower yourself to wield C# arithmetic with finesse, creating robust and accurate applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn