Random Number in a Range in C#
Minahil Noor
Feb 16, 2024
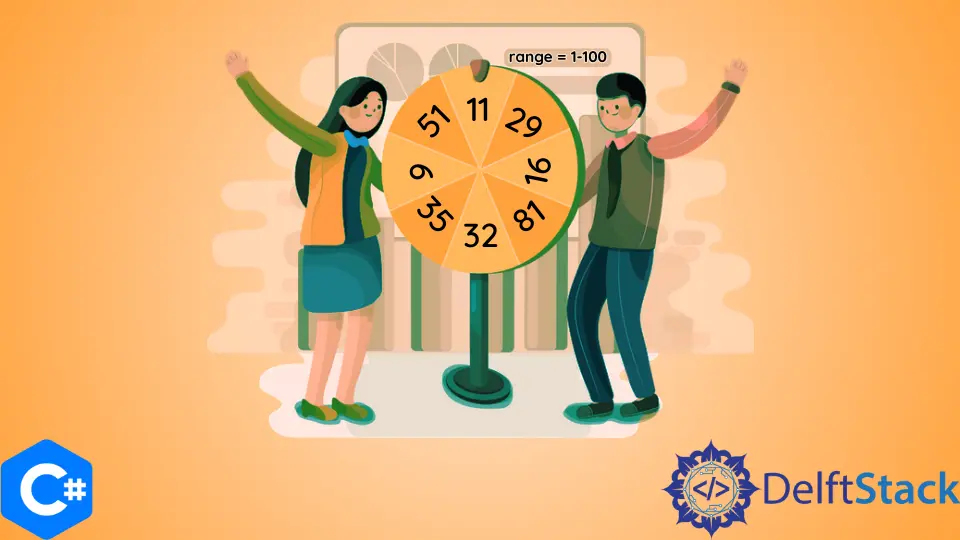
This article will introduce a method to generate a random integer number in a range in C#.
Use the Next()
Method to Generate a Random Integer Number in a Range in C#
We will use the Next()
method to generate a random integer number in a range in C#. This method generates a random integer in a specified range. It has three overloads. To use this method, we will have to create an object of the Random
class. The correct syntax to use this method is as follows.
Random myObject = new Random();
myObject.Next(min, max);
The detail of its parameters is as follows.
Parameters | Description | |
---|---|---|
min |
It is the minimum value or the lower bound of the range. | |
max |
It is the maximum value or upper bound of the range. |
This method returns a random integer in the specified range.
The program below shows how we can use the Next()
method to generate a random integer within a range.
using System;
public class Program {
public static void Main() {
Random myObject = new Random();
int ranNum = myObject.Next(100, 150);
Console.WriteLine("The Random Number is: " + ranNum);
}
}
Output:
The Random Number is: 145