How to Convert String to Int in C#
-
C#
string
toint
Conversion -Int16.Parse()
/Int32.Parse()
/Int64.Parse()
Method -
C#
string
toint
Conversion -Int16.TryParse()
/Int32.TryParse()
/Int64.TryParse()
Method -
C#
String
toInt
Conversion -Convert.ToInt16()
/Convert.ToInt32()
/Convert.ToInt64()
Method
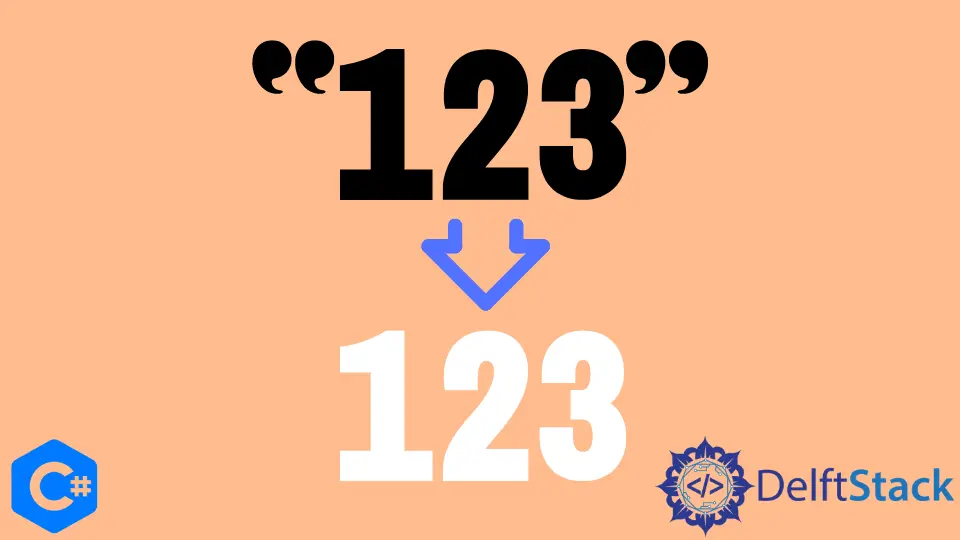
There are certain things you must consider when you convert a string to int. In this article, we will cover some of the easiest and recommended methods to achieve this.
C# string
to int
Conversion - Int16.Parse()
/ Int32.Parse()
/ Int64.Parse()
Method
Parse()
method can be used to convert a string representation of a number to equivalent 16/32/64 bit signed integer. For representational purposes, we will only cover 32-bit conversions from here on.
using System;
public class Instance {
public static void Main() {
string str_var = "123";
int num_var = Int32.Parse(str_var);
System.Console.WriteLine(num_var);
}
}
Output:
123
A slight disadvantage of using this method is that it throws a FormatException
in case the string is not a sequence of digits.
Although Parse()
ignores white spaces at the start and end of the string, apart from that, all characters must be of appropriate numerical value for successful conversion.
We recommend try...catch
statement to handle the exception, as depicted in the following codes.
using System;
public class Instance {
public static void Main() {
string str_var = "abc";
try {
int num_var = Int32.Parse(str_var);
System.Console.WriteLine(num_var);
} catch (FormatException) {
System.Console.WriteLine("Invalid String");
}
}
}
Output:
Invalid String
C# string
to int
Conversion - Int16.TryParse()
/ Int32.TryParse()
/ Int64.TryParse()
Method
Considered as a suitable alternative to Parse()
method, TryParse()
method doesn’t throw an exception in case of failure. Instead, it just returns False
if conversion fails, making implementation simpler.
using System;
public class Instance {
public static void Main() {
bool result;
string str_var = "111";
int num_var;
result = Int32.TryParse(str_var, out num_var);
if (result)
System.Console.WriteLine(num_var);
else
System.Console.WriteLine("Invalid String");
}
}
Output:
111
C# String
to Int
Conversion - Convert.ToInt16()
/ Convert.ToInt32()
/ Convert.ToInt64()
Method
Convert()
method takes string variable as the parameter and returns the equivalent 16/32/64 bit signed numeric value. Similar to Parse()
method, with this method you need a try...catch
statement for exceptions.
using System;
public class Instance {
public static void Main() {
string str_var = "123";
try {
int num_var = Convert.ToInt32(str_var);
System.Console.WriteLine(num_var);
} catch (FormatException) {
Console.WriteLine("Invalid String");
} catch (OverflowException) {
Console.WriteLine("The string cannot fit in 32 bit value");
}
}
}
Output:
123
Exceptions are expensive and are not considered a good practice generally to include in the program flow. But at times they might be useful to extract important information like Overflow condition in the Convert()
method.
All the above methods are equally effective and have certain trade-offs when it comes to implementation. We recommend choosing the optimal one on the basis of considerations we have laid out in this article.
Related Article - Csharp Integer
- How to Convert Int to String in C#
- How to Convert Int to Enum in C#
- Random Int in C#
- Random Number in a Range in C#
- Integer Division in C#