How to Convert a String to Boolean in C#
-
Use the
ToBoolean()
Method to Convert a String to Boolean inC#
-
Use the
TryParse()
Method to Convert a String to Boolean inC#
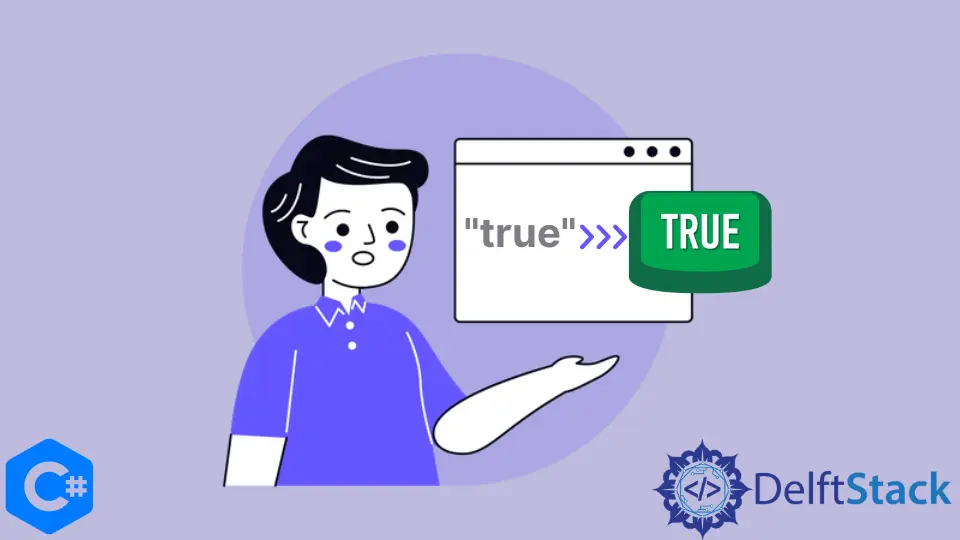
This article will introduce different methods to convert a string to Boolean
in C#, like the ToBoolean()
method and the TryParse()
method.
Use the ToBoolean()
Method to Convert a String to Boolean in C#
In C#, we can use the ToBoolean()
method to convert a string to a boolean value. This method has multiple overloads. The overload that we will use will have only one parameter. We will use the following overload in this case. The correct syntax to use this method is as follows.
Convert.ToBoolean(String stringName);
This overload of the method ToBoolean()
has one parameter only. The detail of its parameter is as follows.
Parameters | Description | |
---|---|---|
stringName |
mandatory | This is the string that we want to convert to the boolean value. |
This function returns a boolean value representing the value given in the string.
The program below shows how we can use the ToBoolean()
method to convert a string to Boolean
.
using System;
using System.Globalization;
class StringToBoolean {
static void Main(string[] args) {
string mystring = "true";
bool value = Convert.ToBoolean(mystring);
Console.WriteLine(value);
}
}
Output:
True
Use the TryParse()
Method to Convert a String to Boolean in C#
In C#, we can also use the TryParse()
method to convert a string to a boolean value. There are multiple overloads of this method. The overload that we will use will have two parameters. One of the parameters will be the out
variable. We will use the following overload in this case. The correct syntax to use this method is as follows.
Boolean.TryParse(String stringName, out variableName);
This overload of the method TryParse()
has two parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
stringName |
mandatory | This is the string that we want to convert to Boolean . |
variableName |
mandatory | It is the output variable in which we want to store our converted value. |
This function returns a boolean value representing the value given in the string.
The program below shows how we can use the TryParse()
method to convert a string to Boolean
.
using System;
using System.Globalization;
class StringToFloat {
static void Main(string[] args) {
string sample = "true";
Boolean myBool;
if (Boolean.TryParse(sample, out myBool)) {
Console.WriteLine(myBool);
}
}
}
Output:
True
Related Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#