How to Use Strings in Switch Statement in C#
- Understanding the Basics of Switch Statements
- Using Strings in Switch Statements
- Case Sensitivity in String Comparisons
- Using Switch Expressions for Enhanced Readability
- Conclusion
- FAQ
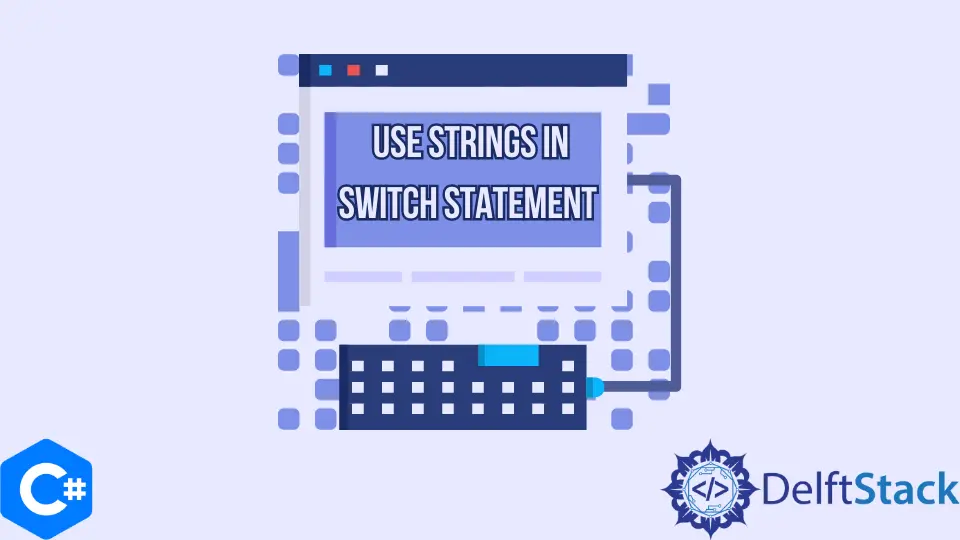
In C#, the switch statement is a powerful control structure that allows you to execute different code blocks based on the value of a variable. While traditionally used with integers or enums, C# 7.0 and later versions introduced the ability to use strings in switch statements. This enhancement opens up a world of possibilities for more readable and maintainable code.
In this article, we will explore how to effectively use strings within a switch statement in C#, providing practical examples and insights. Whether you’re a beginner or looking to sharpen your C# skills, this guide will help you understand how to implement string-based switch statements in your applications.
Understanding the Basics of Switch Statements
Before diving into string usage, it’s essential to understand how switch statements work in C#. A switch statement evaluates a variable against a list of values (called cases) and executes the corresponding block of code for the matching case. If no match is found, the default case (if provided) is executed.
Here’s a basic structure of a switch statement:
switch (variable)
{
case value1:
// Code block for value1
break;
case value2:
// Code block for value2
break;
default:
// Code block if no cases match
break;
}
This structure works seamlessly with strings, allowing you to execute different code blocks based on string values.
Using Strings in Switch Statements
Using strings in a switch statement is straightforward. You simply declare your switch variable as a string and define cases with string literals. This feature enhances code clarity and eliminates the need for multiple if-else statements. Here’s a simple example:
string day = "Monday";
switch (day)
{
case "Monday":
Console.WriteLine("Start of the work week.");
break;
case "Friday":
Console.WriteLine("Almost the weekend!");
break;
case "Saturday":
case "Sunday":
Console.WriteLine("It's the weekend!");
break;
default:
Console.WriteLine("Not a valid day.");
break;
}
Output:
Start of the work week.
In this example, the variable day
is evaluated in the switch statement. If day
matches “Monday,” the corresponding message is printed. The use of strings makes the code more intuitive and easier to manage. You can also group cases, as seen with Saturday and Sunday, which share the same output.
Case Sensitivity in String Comparisons
By default, string comparisons in switch statements are case-sensitive. This means that “monday” and “Monday” are treated as different strings. To handle case insensitivity, you can convert the string to a common case (either upper or lower) before the switch statement. Here’s how you can do it:
string day = "monday";
switch (day.ToLower())
{
case "monday":
Console.WriteLine("Start of the work week.");
break;
case "friday":
Console.WriteLine("Almost the weekend!");
break;
case "saturday":
case "sunday":
Console.WriteLine("It's the weekend!");
break;
default:
Console.WriteLine("Not a valid day.");
break;
}
Output:
Start of the work week.
In this modified example, day.ToLower()
ensures that any variation of “Monday” will be matched correctly, regardless of how the user inputs it. This approach enhances user experience by making your application more forgiving to different input formats.
Using Switch Expressions for Enhanced Readability
C# 8.0 introduced switch expressions, which provide a more concise syntax for switch statements. This modern approach allows you to write cleaner and more readable code. Here’s how you can utilize switch expressions with strings:
string day = "Friday";
string message = day switch
{
"Monday" => "Start of the work week.",
"Friday" => "Almost the weekend!",
"Saturday" or "Sunday" => "It's the weekend!",
_ => "Not a valid day."
};
Console.WriteLine(message);
Output:
Almost the weekend!
In this example, the switch expression evaluates the day
variable and assigns the corresponding message to the message
variable. The use of the or
operator allows for grouping cases elegantly. The underscore _
serves as the default case, making the code both compact and expressive.
Conclusion
Using strings in switch statements in C# is a valuable skill that enhances code readability and maintainability. Whether you’re working with traditional switch statements or adopting the newer switch expressions, understanding how to implement string comparisons effectively can make your applications more user-friendly. As you practice these techniques, you’ll find that they can significantly streamline your coding process and improve your overall programming experience.
FAQ
-
Can I use other data types in a switch statement?
Yes, you can use various data types, including integers, enums, and strings in switch statements. -
Are switch statements case-sensitive in C#?
By default, switch statements are case-sensitive. You can handle this by converting strings to a common case.
-
What is a switch expression in C#?
A switch expression is a more concise syntax introduced in C# 8.0 that allows for cleaner and more readable switch statements. -
Can I use multiple cases for a single output in a switch statement?
Yes, you can group multiple cases together for a single output by using thecase value1:
andcase value2:
syntax. -
Is it better to use switch statements or if-else statements in C#?
It depends on the situation. Switch statements can be more readable and organized when dealing with multiple conditions, especially with strings.
#. Learn the basics, explore case sensitivity, and discover switch expressions for cleaner code. Enhance your C# skills with practical examples and tips for effective string handling in switch statements.
Related Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#