How to Convert a String to a Byte Array in C#
- Why Convert a String to a Byte Array?
-
Using the
GetBytes()
Method - Alternative Method: Manual Conversion
- Conclusion
- FAQ
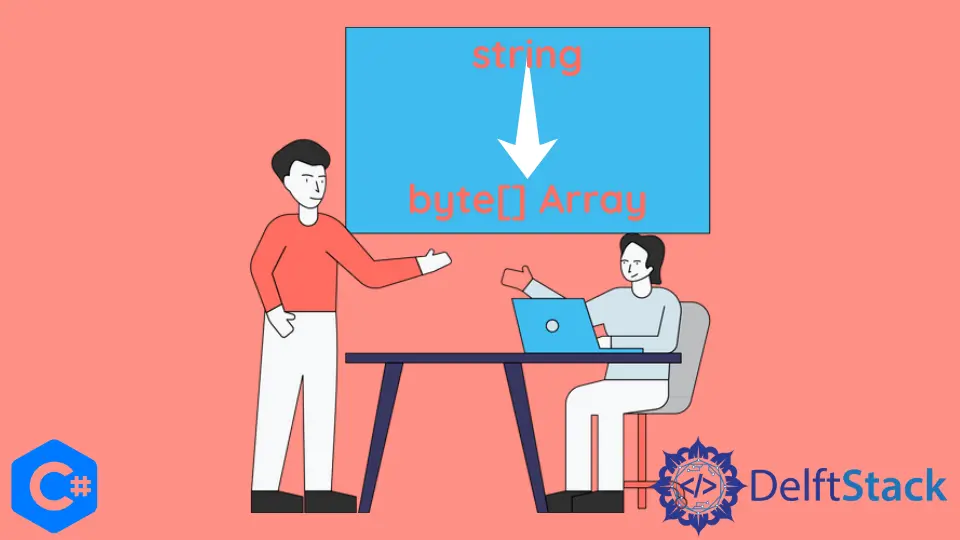
Converting a string to a byte array in C# is a fundamental task that developers often need to perform, whether for data transmission, encryption, or storage. Understanding how to manipulate strings and byte arrays is crucial for effective programming in C#.
In this article, we will explore the various methods to convert a string into a byte array, focusing on the widely-used GetBytes()
method from the System.Text.Encoding
namespace. By the end of this guide, you’ll have a clear understanding of how to implement these conversions in your own projects, along with practical examples to illustrate each method.
Why Convert a String to a Byte Array?
Before diving into the methods, it’s essential to understand why you might need to convert a string to a byte array. Strings in C# are representations of text, while byte arrays are a sequence of bytes that can represent data in various formats. When dealing with network communications, file operations, or encryption, you often need to convert strings into byte arrays. This conversion is crucial for ensuring that data is transmitted or stored in a format that other systems can interpret correctly.
Using the GetBytes()
Method
One of the most straightforward ways to convert a string to a byte array in C# is by using the GetBytes()
method provided by the System.Text.Encoding
class. This method is efficient and allows you to specify the encoding format, such as UTF-8 or ASCII, based on your requirements.
Here’s how you can use the GetBytes()
method:
using System;
using System.Text;
class Program
{
static void Main()
{
string text = "Hello, World!";
byte[] byteArray = Encoding.UTF8.GetBytes(text);
Console.WriteLine("Byte array: " + BitConverter.ToString(byteArray));
}
}
Output:
Byte array: 48-65-6C-6C-6F-2C-20-57-6F-72-6C-64-21
In this example, we first import the necessary namespaces, then define a string variable named text
. We use Encoding.UTF8.GetBytes(text)
to convert the string into a byte array. The BitConverter.ToString(byteArray)
method is used to print the byte array in a readable format. This method is versatile and can handle various string inputs, making it a go-to choice for many developers.
The GetBytes()
method is particularly useful because it allows you to specify the encoding format. UTF-8 is a popular choice as it supports a wide range of characters from different languages. If you need to work with ASCII or other encodings, you can easily switch the encoding type to suit your needs.
Alternative Method: Manual Conversion
While using GetBytes()
is efficient, you might also want to manually convert a string to a byte array. This method gives you more control over the conversion process, allowing for custom implementations if needed.
Here’s an example of how to manually convert a string to a byte array:
using System;
class Program
{
static void Main()
{
string text = "Hello, World!";
byte[] byteArray = new byte[text.Length];
for (int i = 0; i < text.Length; i++)
{
byteArray[i] = (byte)text[i];
}
Console.WriteLine("Byte array: " + BitConverter.ToString(byteArray));
}
}
Output:
Byte array: 48-65-6C-6C-6F-2C-20-57-6F-72-6C-64-21
In this example, we create a byte array of the same length as the string. We then iterate through each character of the string, converting it to its byte representation using a simple cast. This method can be beneficial when you need to customize the conversion logic or handle specific cases that the GetBytes()
method might not cover.
However, manual conversion may not handle character encodings as effectively as the GetBytes()
method. If your application requires support for different languages or special characters, it’s advisable to stick with the built-in methods provided by the .NET framework.
Conclusion
Converting a string to a byte array in C# is a straightforward process that can be accomplished using various methods, with the GetBytes()
method being the most efficient and commonly used. Whether you choose to utilize built-in methods or implement manual conversions, understanding these techniques is essential for effective data manipulation in your applications. As you continue to work with strings and byte arrays, these methods will serve as valuable tools in your programming toolkit.
FAQ
- What is the purpose of converting a string to a byte array?
Converting a string to a byte array is essential for data transmission, encryption, and file operations, ensuring that data is in a format that can be easily processed.
-
Is the
GetBytes()
method the only way to convert a string to a byte array in C#?
No, while GetBytes() is the most common method, you can also manually convert a string to a byte array using loops and casting. -
What encoding should I use when converting strings?
UTF-8 is a popular encoding for general use as it supports a wide range of characters. However, you can choose other encodings based on your specific needs. -
Can I convert a byte array back to a string?
Yes, you can use theGetString()
method from the Encoding class to convert a byte array back into a string. -
Are there performance differences between GetBytes() and manual conversion?
Generally, GetBytes() is optimized for performance and is recommended for standard use, while manual conversion may be slower and less efficient.
Related Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#