How to Remove Element of an Array in C#
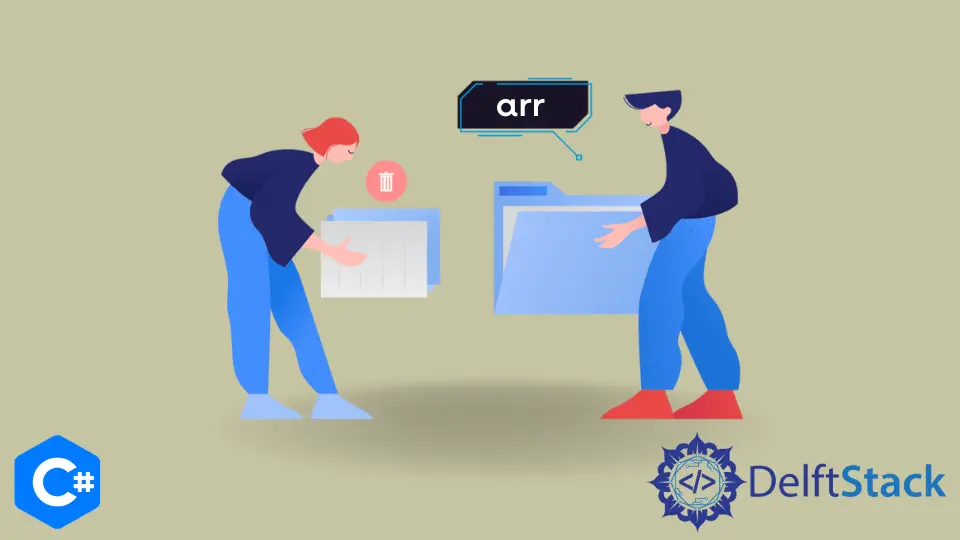
Removing an element from an array in C# can seem daunting, especially for beginners. However, with the right approach, it can be a straightforward task. Arrays are fixed in size, meaning once they’re created, you can’t directly remove an element like you would in a list. But don’t worry!
In this article, we’ll explore effective methods to remove elements from an array using the Where()
clause and array shifting techniques. By the end, you’ll have a solid understanding of how to manipulate arrays in C# and make your code cleaner and more efficient.
Using the Where()
Clause
One of the most elegant ways to remove an element from an array in C# is by using LINQ’s Where()
method. This method allows you to filter elements based on specified criteria, effectively excluding the element(s) you want to remove.
Here’s a simple example demonstrating how to use the Where()
clause:
using System;
using System.Linq;
class Program
{
static void Main()
{
int[] numbers = { 1, 2, 3, 4, 5 };
int elementToRemove = 3;
int[] result = numbers.Where(num => num != elementToRemove).ToArray();
Console.WriteLine(string.Join(", ", result));
}
}
Output:
1, 2, 4, 5
In this example, we start with an array of integers. We specify the element we want to remove (in this case, the number 3). The Where()
method creates a new array that includes only those numbers that do not match the specified element. Finally, we convert the result back to an array using ToArray()
and print the new array. This approach is clean and leverages LINQ for efficient data manipulation.
Shifting Elements in an Array
Another method to remove an element from an array involves manually shifting elements. This technique is particularly useful when you want to modify the original array rather than creating a new one.
Here’s how you can implement this approach:
using System;
class Program
{
static void Main()
{
int[] numbers = { 1, 2, 3, 4, 5 };
int elementToRemove = 3;
int index = Array.IndexOf(numbers, elementToRemove);
if (index >= 0)
{
for (int i = index; i < numbers.Length - 1; i++)
{
numbers[i] = numbers[i + 1];
}
Array.Resize(ref numbers, numbers.Length - 1);
}
Console.WriteLine(string.Join(", ", numbers));
}
}
Output:
1, 2, 4, 5
In this code snippet, we first find the index of the element we want to remove using Array.IndexOf()
. If the element exists, we shift all subsequent elements to the left, effectively overwriting the element we want to remove. Finally, we resize the array to remove the last duplicate entry. This method is efficient in terms of memory and allows for direct modification of the original array.
Conclusion
Removing an element from an array in C# can be accomplished through various methods, such as using the Where()
clause for a functional approach or manually shifting elements for a more hands-on technique. Understanding these methods will enhance your programming skills and allow you to manipulate arrays more effectively. Whether you’re working on small projects or larger applications, knowing how to manage arrays will undoubtedly come in handy.
FAQ
-
What is the difference between an array and a list in C#?
An array is a fixed-size collection, while a list can dynamically grow or shrink as elements are added or removed. -
Can I remove multiple elements from an array at once?
Yes, you can use theWhere()
clause with a condition that matches multiple elements to filter them out. -
Is it possible to remove an element from an array without creating a new array?
Yes, by shifting elements, you can remove an element from the original array, but this method may require resizing the array. -
What happens if I try to remove an element that doesn’t exist in the array?
The methods shown will simply leave the array unchanged if the specified element is not found. -
Are there performance considerations when removing elements from large arrays?
Yes, using LINQ methods likeWhere()
may involve more overhead compared to manual shifting, especially with large datasets.