How to Sort an Array in Descending Order in C#
-
Use the
Array.Sort()
andArray.Reverse()
Method to Sort an Array in Descending Order inC#
-
Use the
OrderByDescending()
Method to Sort an Array in Descending Order inC#
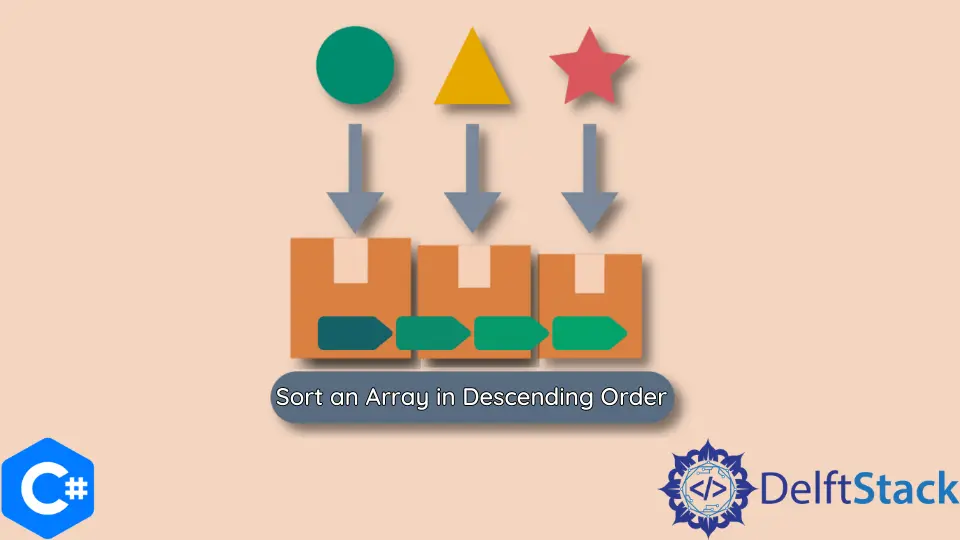
This article will introduce different methods to sort an array in descending order in C#.
Use the Array.Sort()
and Array.Reverse()
Method to Sort an Array in Descending Order in C#
We will use the two methods Array.sort()
and Array.Reverse()
collectively to sort an array in descending order. The Array.Sort()
method sorts the array in ascending order. We will reverse the array using Array.Reverse()
method to sort our array in descending order. There are multiple overloads of these methods. The correct syntax to use these methods is as follows.
Array.Sort(Array array);
This overload of the method Sort()
has one parameter only. The detail of its parameter is as follows
Parameters | Description | |
---|---|---|
array |
mandatory | This is the array that we want to sort. |
This function sorts the array in ascending order.
Array.Reverse(Array array);
This overload of the method Reverse()
has one parameter only. The detail of its parameter is as follows.
Parameters | Description | |
---|---|---|
array |
mandatory | This is the array that we want to reverse. |
This function reverses the given array.
The program below shows how we can use the Sort()
and Reverse()
methods to sort an array in descending order.
using System;
class Sort {
public static void Main() {
int[] arr = new int[] { 2, 10, 5, 8, 4, 11 };
Console.WriteLine("Array Before Sorting:\n");
foreach (int value in arr) {
Console.Write(value + " ");
}
Console.WriteLine("\n");
Array.Sort(arr);
Array.Reverse(arr);
Console.WriteLine("Array After Sorting:\n");
foreach (int value in arr) {
Console.Write(value + " ");
}
}
}