How to Get the Length of an Array in C#
Minahil Noor
Feb 16, 2024
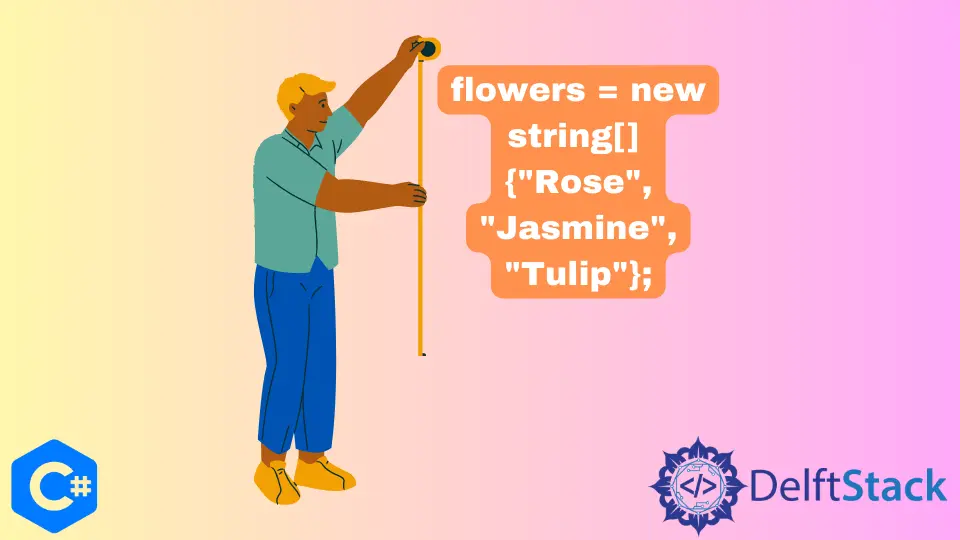
This article will introduce a method to find the length of an array in C#.
Use the Array.Length
Property to Find the Length of an Array in C#
In C#, we can use the Array.Length
property to find the length of an array. This property finds the total number of elements present in an array. The correct syntax to use this property is as follows.
ArrayName.Length;
This property returns the length of the array.
The program below shows how we can use the Array.Length
property to find an array’s length.
using System;
namespace FindLength {
class Length {
public static void Main() {
string[] flowers;
flowers = new string[] { "Rose", "Jasmine", "Lili", "Hibiscus", "Tulip", "Sun Flower" };
foreach (string flower in flowers) Console.Write(flower + "\n");
Console.Write("\nThe Number of Elements in the Array is: ");
Console.Write(flowers.Length);
}
}
}
Output:
Rose
Jasmine
Lili
Hibiscus
Tulip
Sun Flower
The Number of Elements in the Array is: 6