How to Adding Values to a C# Array
-
C# Add Values to Array Using
for
Loop -
C# Add Values to Array Using
List
Data Structure andList<T>.Add(T)
Method
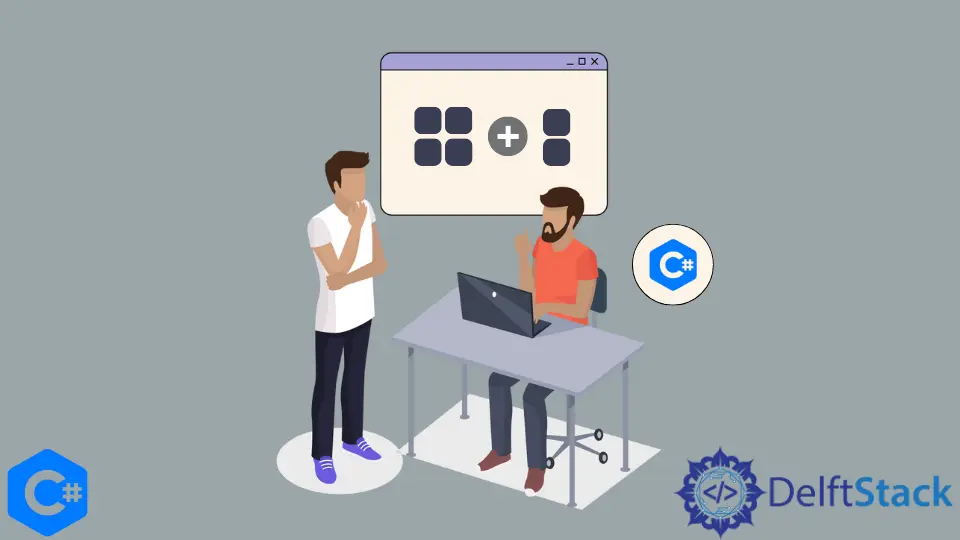
Array is an efficient data structure used to store a collection of variables of the same data type. Data in an array is structured and organized contiguously.
In this article, we will closely follow arrays in C# and look into ways to add values to a declared array.
Let’s first declare an integer array.
int[] arr_sample;
The above is the declaration of the array specifying its data type and name. To fill values to this array, we need to create an object of this array.
int[] arr_sample = new int[5];
As you will notice when we create an object, we also specify the size of the array. C# allows you to skip the dimensions in case you have specified the initializer list. But it’s a good practice to always include the size in the definition.
Let’s look at some of the methods you can use to fill this declared array.
C# Add Values to Array Using for
Loop
Each element has a unique index when it comes to arrays in general. So it’s easy to add values using a simple loop and incremental indexing.
using System;
public class Sample {
public static void Main() {
int[] arr_sample = new int[5];
for (int index = 0; index < 5; index++) {
arr_sample[index] = index;
System.Console.WriteLine(arr_sample[index]);
}
}
}
Output:
0
1
2
3
4
This method is simple but you need to be careful not to exceed the bounds of the array while indexing. Otherwise, that will lead to a Run-time
exception.
C# Add Values to Array Using List
Data Structure and List<T>.Add(T)
Method
You can use a list data structure for this purpose too, as an intermediary data structure. This method is particularly convenient when you are not sure about the size of the array.
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
List<int> intermediate_list = new List<int>();
int index;
for (index = 0; index < 5; index++) intermediate_list.Add(index);
int[] arr_sample = intermediate_list.ToArray();
for (index = 0; index < 5; index++) System.Console.WriteLine(arr_sample[index]);
}
}
Output:
0
1
2
3
4
This method doesn’t require you to fixate on the size of the array in compile time. You can actually go on filling the list according to your convenience and decide on the size of the array dynamically.
Making a choice out of listed methods in this article is easy. If you want a dynamically sized array, go for the list
method. And if you have already decided on the dimensions of the array, looping and filling the array is more convenient.