向 C# 数组中添加数值
Puneet Dobhal
2023年10月12日
Csharp
Csharp Array
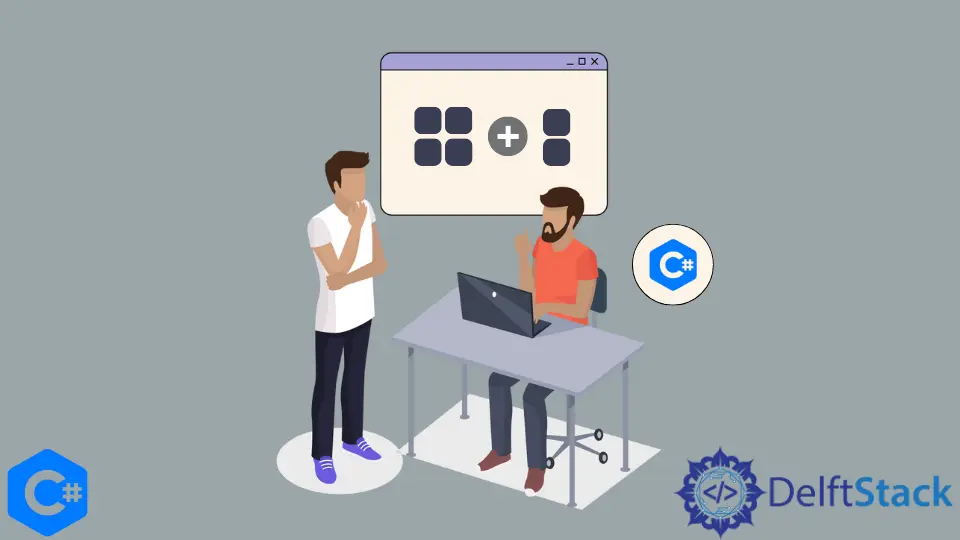
数组是一种有效的数据结构,用于存储相同数据类型的变量的集合。数组中的数据是连续构造和组织的。在本文中,我们将关注 C# 中的数组,并研究将数值添加到已声明的数组中的方法。
首先声明一个整数数组。
int[] arr_sample;
上面是数组的声明,指定了它的数据类型和名称。要向该数组添加数值,我们需要创建此数组的对象。
int[] arr_sample = new int[5];
你在创建对象时注意到,我们还指定了数组的大小。如果已指定初始化列表,则 C# 允许你跳过尺寸。但是,最好在定义中始终包含数组大小。
让我们看一些可用于填充此数组的方法。
C# 使用 for
循环将值添加到数组
通常,每个元素在数组中都有一个唯一的索引。因此,容易使用简单的循环和增量索引添加数值。
using System;
public class Sample {
public static void Main() {
int[] arr_sample = new int[5];
for (int index = 0; index < 5; index++) {
arr_sample[index] = index;
System.Console.WriteLine(arr_sample[index]);
}
}
}
输出:
0
1
2
3
4
此方法很简单,但在使用索引时请注意不要超出数组的范围。否则,将导致 Run-time
异常。
C# 使用 List
数据结构和 List<T>.Add(T)
方法向数组添加值
你也可以将列表数据结构用作中间数据结构。当你不确定数组的大小时,此方法特别方便。
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
List<int> intermediate_list = new List<int>();
int index;
for (index = 0; index < 5; index++) intermediate_list.Add(index);
int[] arr_sample = intermediate_list.ToArray();
for (index = 0; index < 5; index++) System.Console.WriteLine(arr_sample[index]);
}
}
输出:
0
1
2
3
4
此方法不需要你在编译时就固定数组的大小。实际上,你可以根据自己的方便继续填写列表,并动态决定数组的大小。
从本文列出的方法中进行选择最优方案是很容易的。如果你想要一个动态大小的数组,请使用 list
方法。而且,如果你已经确定了数组的尺寸,则循环和填充数组会更加方便。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe