C# 读取 CSV 文件并将其值存储到数组中
Minahil Noor
2023年10月12日
- C# 使用 StreamReader 类读取 CSV 文件并将其值存储到数组中
-
C# 使用
Microsoft.VisualBasic.FileIO
库的TextFieldParser
读取 CSV 文件并将其值存储到数组中
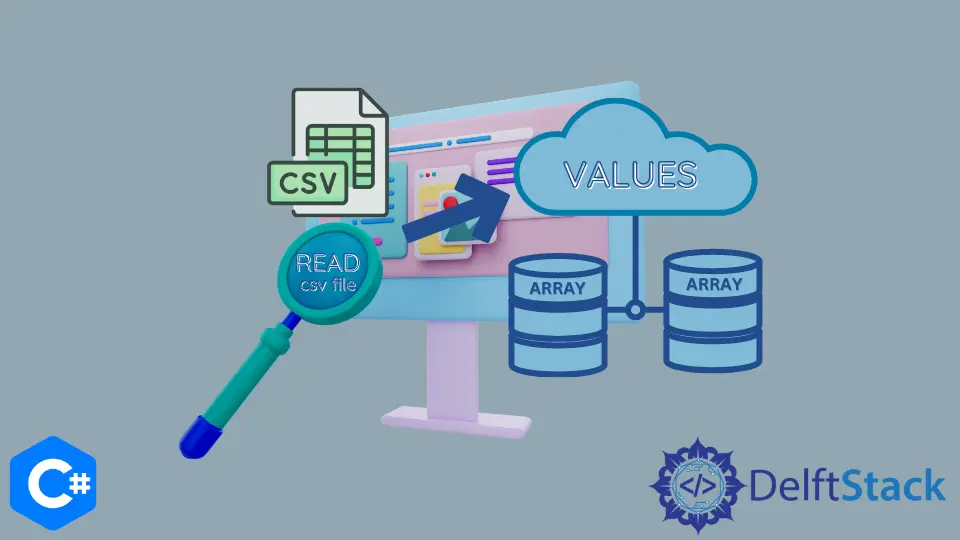
CSV 文件是 Comma Separated Values
,用于以有组织的方式存储数据。它通常以表格形式存储数据。大多数企业组织将其数据存储在 CSV 文件中。
在 C# 中,我们可以对 CSV 文件执行各种操作。我们可以读取,写入和更改 CSV
文件。本文重点介绍各种读取 CSV 文件并将其数据存储到数组中的方法。
C# 使用 StreamReader 类读取 CSV 文件并将其值存储到数组中
在 C# 中,StreamReader
类用于处理文件。它会打开,读取并帮助你对不同类型的文件执行其他功能。使用此类时,我们还可以对 CSV 文件执行不同的操作。
OpenRead()方法用于打开 CSV 文件,而 ReadLine()方法用于读取其内容。
使用 OpenRead()和 ReadLine()方法的正确语法如下:
// OpenRead() Syntax
File.OpenRead(@"FilePath");
// ReadLine() Syntax
StreamReaderObject.ReadLine();
示例代码:
using System.IO;
using System.Collections.Generic;
using System;
class ReadingCSV {
static void Main(string[] args) {
var reader = new StreamReader(File.OpenRead(@"D:\New folder\Data.csv"));
List<string> listA = new List<string>();
List<string> listB = new List<string>();
while (!reader.EndOfStream) {
var line = reader.ReadLine();
var values = line.Split(';');
listA.Add(values[0]);
listB.Add(values[1]);
foreach (var coloumn1 in listA) {
Console.WriteLine(coloumn1);
}
foreach (var coloumn2 in listA) {
Console.WriteLine(coloumn2);
}
}
}
}
输出:
//Contents of the CSV file
C# 使用 Microsoft.VisualBasic.FileIO
库的 TextFieldParser
读取 CSV 文件并将其值存储到数组中
在 C# 中,我们有一个文件解析器,可以根据文件的内容来解析文件。TextFieldParser
在 Microsoft.VisualBasic.FileIO
库中定义。在执行下面的程序之前,请不要忘记添加对 Microsoft.VisualBasic 的引用。
使用此解析器的正确语法如下:
TextFieldParser ParserName = new TextFieldParser(PathString);
示例代码:
using System;
using Microsoft.VisualBasic.FileIO;
class ReadingCSV {
public static void Main() {
string coloumn1;
string coloumn2;
var path = @"D:\New folder\Data.csv";
using (TextFieldParser csvReader = new TextFieldParser(path)) {
csvReader.CommentTokens = new string[] { "#" };
csvReader.SetDelimiters(new string[] { "," });
csvReader.HasFieldsEnclosedInQuotes = true;
// Skip the row with the column names
csvReader.ReadLine();
while (!csvReader.EndOfData) {
// Read current line fields, pointer moves to the next line.
string[] fields = csvReader.ReadFields();
coloumn1 = fields[0];
coloumn2 = fields[1];
}
}
}
}