How to Read a CSV File and Store Its Values Into an Array in C#
-
C# Program to Read a
CSV File
and Store Its Value Into anArray
UsingStreamReader
Class -
C# Program to Read a
CSV
File and Store Its Value Into anArray
UsingMicrosoft.VisualBasic.FileIO
Library’sTextFieldParser
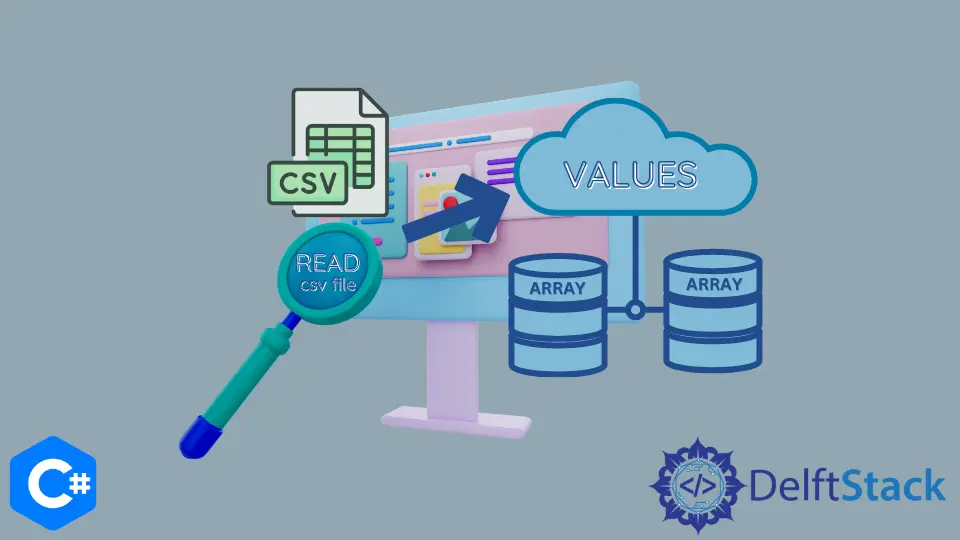
A CSV
file is a comma-separated file, that is used to store data in an organized way. It usually stores data in tabular form. Most of the business organizations store their data in CSV files
.
In C#, we can perform various operations on a CSV
file. We can read, write and alter a CSV
file. This article focuses on various methods to read a CSV
file and store its data into an array.
C# Program to Read a CSV File
and Store Its Value Into an Array
Using StreamReader
Class
In C#, StreamReader
class is used to deal with the files. It opens, reads and helps in performing other functions to different types of files. We can also perform different operations on a CSV
file while using this class.
OpenRead()
method is used to open a CSV
file and ReadLine()
method is used to read its contents.
The correct syntax to use OpenRead()
and ReadLine()
method is as follows:
// OpenRead() Syntax
File.OpenRead(@"FilePath");
// ReadLine() Syntax
StreamReaderObject.ReadLine();
Example Code:
using System.IO;
using System.Collections.Generic;
using System;
class ReadingCSV {
static void Main(string[] args) {
var reader = new StreamReader(File.OpenRead(@"D:\New folder\Data.csv"));
List<string> listA = new List<string>();
List<string> listB = new List<string>();
while (!reader.EndOfStream) {
var line = reader.ReadLine();
var values = line.Split(';');
listA.Add(values[0]);
listB.Add(values[1]);
foreach (var coloumn1 in listA) {
Console.WriteLine(coloumn1);
}
foreach (var coloumn2 in listA) {
Console.WriteLine(coloumn2);
}
}
}
}
Output:
//Contents of the CSV file
C# Program to Read a CSV
File and Store Its Value Into an Array
Using Microsoft.VisualBasic.FileIO
Library’s TextFieldParser
In C#, we have a File Parser
that parses the file based on its contents. The TextFieldParser
is defined in Microsoft.VisualBasic.FileIO
library. Before executing the program below, don’t forget to add reference to Microsoft.VisualBasic
.
The correct syntax to use this parser is as follows:
TextFieldParser ParserName = new TextFieldParser(PathString);
Example Code:
using System;
using Microsoft.VisualBasic.FileIO;
class ReadingCSV {
public static void Main() {
string coloumn1;
string coloumn2;
var path = @"D:\New folder\Data.csv";
using (TextFieldParser csvReader = new TextFieldParser(path)) {
csvReader.CommentTokens = new string[] { "#" };
csvReader.SetDelimiters(new string[] { "," });
csvReader.HasFieldsEnclosedInQuotes = true;
// Skip the row with the column names
csvReader.ReadLine();
while (!csvReader.EndOfData) {
// Read current line fields, pointer moves to the next line.
string[] fields = csvReader.ReadFields();
coloumn1 = fields[0];
coloumn2 = fields[1];
}
}
}
}
Related Article - Csharp CSV
- How to Write Data Into a CSV File in C#
- How to Read a CSV File Into a DataTable in C#
- How to Convert DataTable to CSV in C#
- How to Parse CSV File in C#