C# 讀取 CSV 檔案並將其值儲存到陣列中
Minahil Noor
2023年10月12日
Csharp
Csharp CSV
Csharp Array
- C# 使用 StreamReader 類讀取 CSV 檔案並將其值儲存到陣列中
-
C# 使用
Microsoft.VisualBasic.FileIO
庫的TextFieldParser
讀取 CSV 檔案並將其值儲存到陣列中
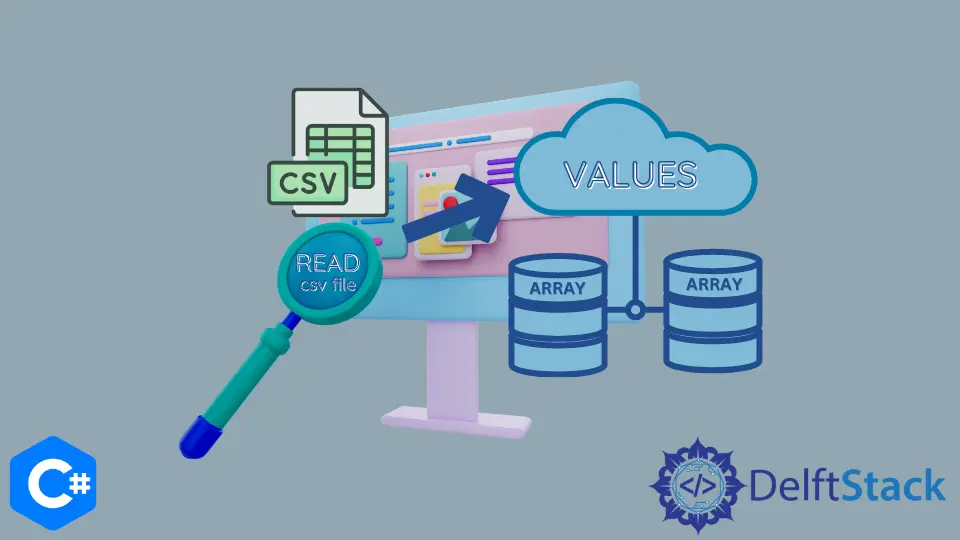
CSV 檔案是 Comma Separated Values
,用於以有組織的方式儲存資料。它通常以表格形式儲存資料。大多數企業組織將其資料儲存在 CSV 檔案中。
在 C# 中,我們可以對 CSV 檔案執行各種操作。我們可以讀取,寫入和更改 CSV
檔案。本文重點介紹各種讀取 CSV 檔案並將其資料儲存到陣列中的方法。
C# 使用 StreamReader 類讀取 CSV 檔案並將其值儲存到陣列中
在 C# 中,StreamReader
類用於處理檔案。它會開啟,讀取並幫助你對不同型別的檔案執行其他功能。使用此類時,我們還可以對 CSV 檔案執行不同的操作。
OpenRead()方法用於開啟 CSV 檔案,而 ReadLine()方法用於讀取其內容。
使用 OpenRead()和 ReadLine()方法的正確語法如下:
// OpenRead() Syntax
File.OpenRead(@"FilePath");
// ReadLine() Syntax
StreamReaderObject.ReadLine();
示例程式碼:
using System.IO;
using System.Collections.Generic;
using System;
class ReadingCSV {
static void Main(string[] args) {
var reader = new StreamReader(File.OpenRead(@"D:\New folder\Data.csv"));
List<string> listA = new List<string>();
List<string> listB = new List<string>();
while (!reader.EndOfStream) {
var line = reader.ReadLine();
var values = line.Split(';');
listA.Add(values[0]);
listB.Add(values[1]);
foreach (var coloumn1 in listA) {
Console.WriteLine(coloumn1);
}
foreach (var coloumn2 in listA) {
Console.WriteLine(coloumn2);
}
}
}
}
輸出:
//Contents of the CSV file
C# 使用 Microsoft.VisualBasic.FileIO
庫的 TextFieldParser
讀取 CSV 檔案並將其值儲存到陣列中
在 C# 中,我們有一個檔案解析器,可以根據檔案的內容來解析檔案。TextFieldParser
在 Microsoft.VisualBasic.FileIO
庫中定義。在執行下面的程式之前,請不要忘記新增對 Microsoft.VisualBasic 的引用。
使用此解析器的正確語法如下:
TextFieldParser ParserName = new TextFieldParser(PathString);
示例程式碼:
using System;
using Microsoft.VisualBasic.FileIO;
class ReadingCSV {
public static void Main() {
string coloumn1;
string coloumn2;
var path = @"D:\New folder\Data.csv";
using (TextFieldParser csvReader = new TextFieldParser(path)) {
csvReader.CommentTokens = new string[] { "#" };
csvReader.SetDelimiters(new string[] { "," });
csvReader.HasFieldsEnclosedInQuotes = true;
// Skip the row with the column names
csvReader.ReadLine();
while (!csvReader.EndOfData) {
// Read current line fields, pointer moves to the next line.
string[] fields = csvReader.ReadFields();
coloumn1 = fields[0];
coloumn2 = fields[1];
}
}
}
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe