How to Parse CSV File in C#
-
Parse CSV File With the
TextFieldParser
Class inC#
-
Parse CSV File With the
FileHelpers
Library inC#
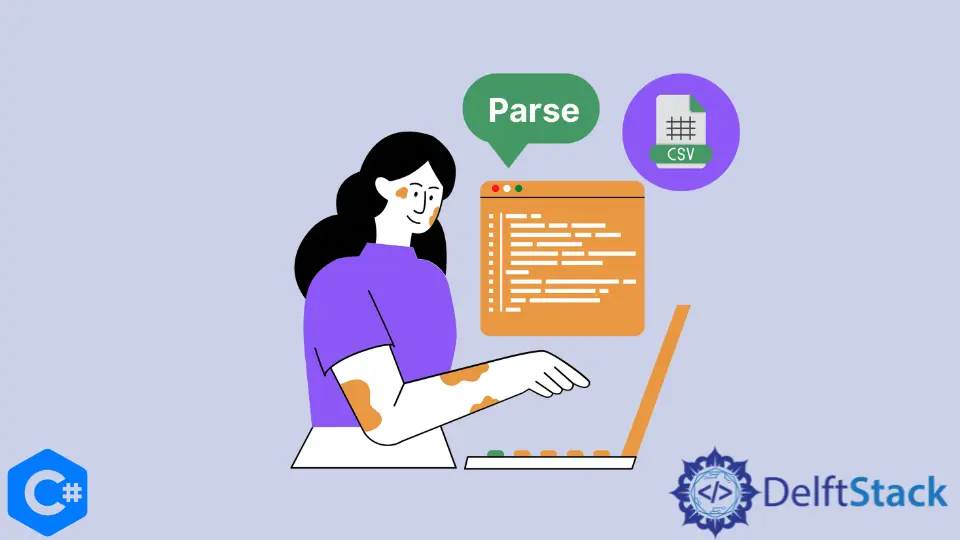
This tutorial will discuss the methods to parse a CSV file in C#.
Parse CSV File With the TextFieldParser
Class in C#
To use the TextFieldParser
class, we have to reference the Microsoft.VisualBasic.dll
in our C# code. The TextFieldParser
class contains many methods for parsing structured text files in C#. We can read a CSV file with the TextFieldParser
class by setting the delimiters to ,
with the SetDelimiters()
function inside the TextFieldParser
class. The following code example shows us how to parse a CSV file with the TextFieldParser
class in C#.
using System;
using Microsoft.VisualBasic.FileIO;
namespace parse_csv {
class Program {
static void Main(string[] args) {
using (TextFieldParser textFieldParser = new TextFieldParser(@"C:\File\Sheet1.csv")) {
textFieldParser.TextFieldType = FieldType.Delimited;
textFieldParser.SetDelimiters(",");
while (!textFieldParser.EndOfData) {
string[] rows = textFieldParser.ReadFields();
}
}
}
}
}
In the above code, we initialized the instance textFieldParser
of the TextFieldParser
class by specifying the path to our CSV file in the constructor. We then set our text field type to be delimited with the textFieldParser.TextFieldType = FieldType.Delimited
and set ,
as the delimiter with textFieldParser.SetDelimiter(',')
function. We then used a while
loop to read the CSV file to the end with the textFieldParser.EndofData
. We stored the data inside an array of strings with the ReadFields()
function.
Parse CSV File With the FileHelpers
Library in C#
The FileHelpers
library is used to read and write data to files, streams, and strings in C#. It is a third-party library and does not come pre-installed with the .NET
framework. We can easily install it by searching it in the NuGet package manager in the Visual Studio IDE. We can use the FileHelpersEngine
class to parse data from a CSV file in C#. The FileHelperEngine
class gets the data from the file into class objects in C#. So, we have first to create a model class that can hold our data from the file. The class would contain fields that represent columns in the CSV file. We can use the [DelimitedRecord(",")]
to specify that the ,
is used as a delimiter here. We can use the ReadFile(path)
function to read data inside an array of class objects from the file in the specified path. The following code example shows us how to parse a CSV file with the FileHelpers
library in C#.
using FileHelpers;
using System;
namespace parse_csv {
[DelimitedRecord(",")]
public class Record {
public string Name;
public string Age;
}
class Program {
static void Main(string[] args) {
var fileHelperEngine = new FileHelperEngine<Record>();
var records = fileHelperEngine.ReadFile(@"C:\File\records.csv");
foreach (var record in records) {
Console.WriteLine(record.Name);
Console.WriteLine(record.Age);
}
}
}
}
Output:
Name
Age
MMA
22
SDA
19
SHA
11
In the above code, we read the data inside the C:\File\records.csv
file and save it in an array of objects of the Record
class with the FileHelpers
library in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn