How to Write Data Into a CSV File in C#
-
C# Program to Write Data Into a
CSV
File UsingCsvHelper
Class Methods -
C# Program to Write Data Into a
CSV
File UsingWriteAllText()
Method
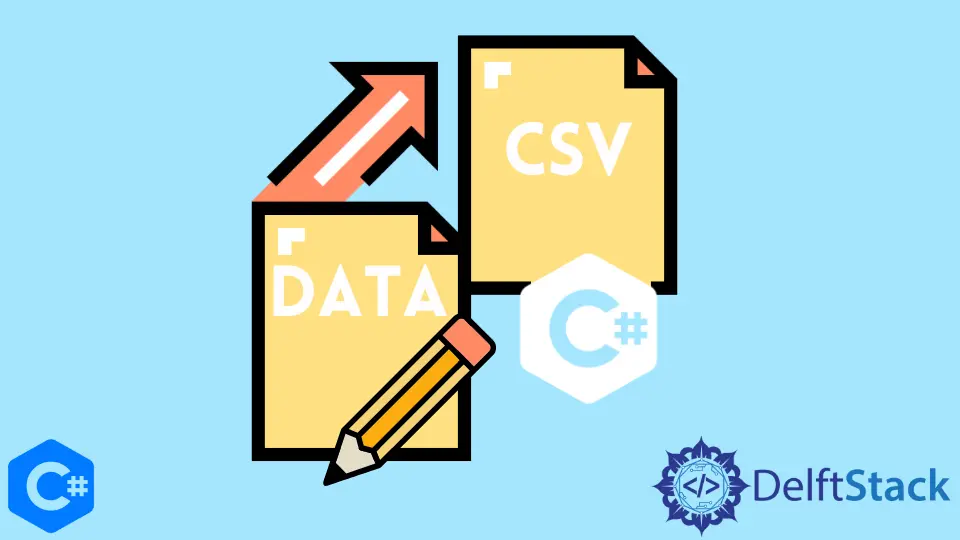
CSV
files are of great use in storing data in an organized way. You can write and read data to and from a CSV
file using different programming languages.
In C# we have different methods for reading a CSV
file and writing the data to a CSV
file. This article focusses on the different methods to write data into a CSV
file.
C# Program to Write Data Into a CSV
File Using CsvHelper
Class Methods
In C#, we have a library named CsvHelper
for reading and writing CSV
files. This library is not provided by default. Rather, we have to download it. It provides methods like GetRecords
and WriteRecords
to read and write a CSV file
. This is an efficient library to read and write CSV files
easily.
The following example code shows a program that creates a CSV file
and writes data into it.
Example Code:
using System;
using System.IO;
using System.Text;
using CsvHelper;
namespace CsvExample {
public class Project {
public string PersonName { get; set; }
public string Title { get; set; }
}
public class Program {
static void Main(string[] args) {
var data = new[] { new Project { CustomerName = "Olivia", Title = "Mother" },
new Project { CustomerName = "Lili", Title = "Elder Sister" } };
using (var mem = new MemoryStream()) using (var writer = new StreamWriter(mem)) using (
var csvWriter = new CsvWriter(writer)) {
csvWriter.Configuration.Delimiter = ";";
csvWriter.Configuration.HasHeaderRecord = true;
csvWriter.Configuration.AutoMap<Project>();
csvWriter.WriteHeader<Project>();
csvWriter.WriteRecords(data);
writer.Flush();
var result = Encoding.UTF8.GetString(mem.ToArray());
Console.WriteLine(result);
}
}
}
}
Output:
PersonName;Title
Olivia;Mother
Lili;Elder Sister
C# Program to Write Data Into a CSV
File Using WriteAllText()
Method
The method WriteAllText()
creates a file, writes data into the file and then closes the file. If the file already exists then it overwrites data in the file.
The correct syntax to use this method is as follows:
WriteAllText(StringPath, StringContents);
Example Code:
using System;
using System.IO;
using System.Text;
namespace CsvExample {
public class Program {
static void Main(string[] args) {
string strFilePath = @"D:\New folder\Data.csv";
string strSeperator = ",";
StringBuilder sbOutput = new StringBuilder();
int[][] inaOutput = new int[][] { new int[] { 1000, 2000, 3000, 4000, 5000 },
new int[] { 6000, 7000, 8000, 9000, 10000 },
new int[] { 11000, 12000, 13000, 14000, 15000 } };
int ilength = inaOutput.GetLength(0);
for (int i = 0; i & amp; lt; ilength; i++)
sbOutput.AppendLine(string.Join(strSeperator, inaOutput[i]));
// Create and write the csv file
File.WriteAllText(strFilePath, sbOutput.ToString());
// To append more lines to the csv file
File.AppendAllText(strFilePath, sbOutput.ToString());
}
}
}
Output:
//CSV file created in the given directory
It gives several exceptions like ArgumentException
, ArgumentNullException
, PathTooLongException
, DirectoryNotFoundException
, IOException
and UnauthorizedAccessException
. These exceptions could be handled using a try-catch
block.