C# CSV 파일에 데이터 쓰기
Minahil Noor
2023년10월12일
Csharp
Csharp CSV
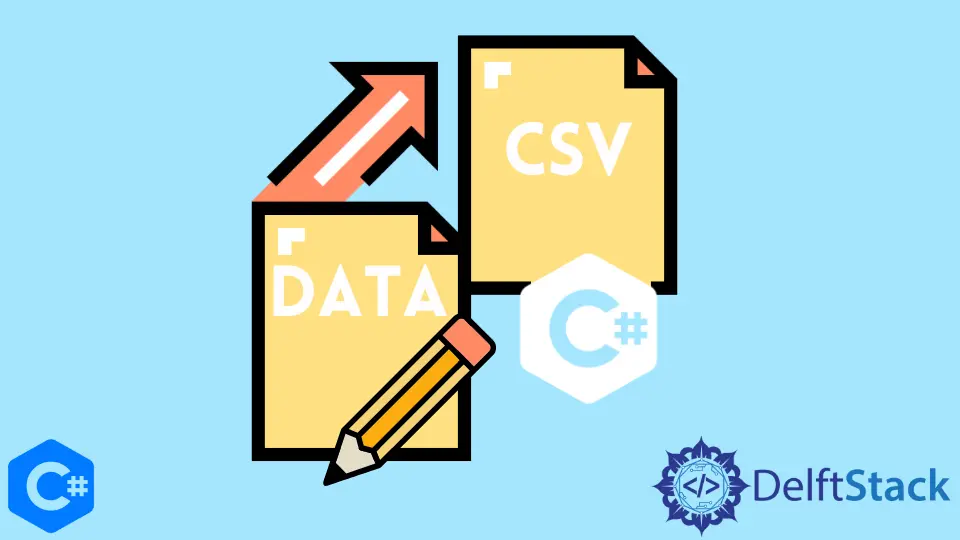
CSV
파일은 체계적인 방식으로 데이터를 저장하는 데 매우 유용합니다. 다른 프로그래밍 언어를 사용하여CSV
파일에서 데이터를 쓰고 읽을 수 있습니다.
C#에는CSV
파일을 읽고CSV
파일에 데이터를 쓰는 다른 방법이 있습니다. 이 기사는CSV
파일에 데이터를 쓰는 다른 방법에 중점을 둡니다.
CsvHelper
클래스 메소드를 사용하여CSV
파일에 데이터를 쓰는 C# 프로그램
C#에는CSV
파일 ‘을 읽고 쓰는 라이브러리 인CsvHelper
가 있습니다. 이 라이브러리는 기본적으로 제공되지 않습니다. 오히려 다운로드가 필요합니다. GetRecords
및WriteRecords
와 같은 메소드를 제공하여CSV 파일
을 읽고 쓸 수 있습니다. CSV 파일을 쉽게 읽고 쓸 수있는 효율적인 라이브러리입니다.
다음 예제 코드는 ‘CSV 파일’을 작성하고 데이터를 쓰는 프로그램을 보여줍니다.
예제 코드:
using System;
using System.IO;
using System.Text;
using CsvHelper;
namespace CsvExample {
public class Project {
public string PersonName { get; set; }
public string Title { get; set; }
}
public class Program {
static void Main(string[] args) {
var data = new[] { new Project { CustomerName = "Olivia", Title = "Mother" },
new Project { CustomerName = "Lili", Title = "Elder Sister" } };
using (var mem = new MemoryStream()) using (var writer = new StreamWriter(mem)) using (
var csvWriter = new CsvWriter(writer)) {
csvWriter.Configuration.Delimiter = ";";
csvWriter.Configuration.HasHeaderRecord = true;
csvWriter.Configuration.AutoMap<Project>();
csvWriter.WriteHeader<Project>();
csvWriter.WriteRecords(data);
writer.Flush();
var result = Encoding.UTF8.GetString(mem.ToArray());
Console.WriteLine(result);
}
}
}
}
출력:
PersonName;Title
Olivia;Mother
Lili;Elder Sister
WriteAllText()
메소드를 사용하여CSV
파일에 데이터를 쓰는 C# 프로그램
WriteAllText()
메소드는 파일을 생성하고 파일에 데이터를 쓴 다음 파일을 닫습니다. 파일이 이미 존재하면 파일의 데이터를 덮어 씁니다.
이 방법을 사용하는 올바른 구문은 다음과 같습니다.
WriteAllText(StringPath, StringContents);
예제 코드:
using System;
using System.IO;
using System.Text;
namespace CsvExample {
public class Program {
static void Main(string[] args) {
string strFilePath = @"D:\New folder\Data.csv";
string strSeperator = ",";
StringBuilder sbOutput = new StringBuilder();
int[][] inaOutput = new int[][] { new int[] { 1000, 2000, 3000, 4000, 5000 },
new int[] { 6000, 7000, 8000, 9000, 10000 },
new int[] { 11000, 12000, 13000, 14000, 15000 } };
int ilength = inaOutput.GetLength(0);
for (int i = 0; i & amp; lt; ilength; i++)
sbOutput.AppendLine(string.Join(strSeperator, inaOutput[i]));
// Create and write the csv file
File.WriteAllText(strFilePath, sbOutput.ToString());
// To append more lines to the csv file
File.AppendAllText(strFilePath, sbOutput.ToString());
}
}
}
출력:
//CSV file created in the given directory
ArgumentException
,ArgumentNullException
,PathTooLongException
,DirectoryNotFoundException
,IOException
및UnauthorizedAccessException
과 같은 몇 가지 예외를 제공합니다. 이러한 이상들은 try-catch
블록을 사용하여 처리할 수 있습니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다