How to Convert Byte Array to String in C#
-
Convert a Byte Array to a
String
UsingEncoding.GetString()
Method inC#
-
C# Program to Convert a
Byte Array
to aString
UsingMemoryStream
Method
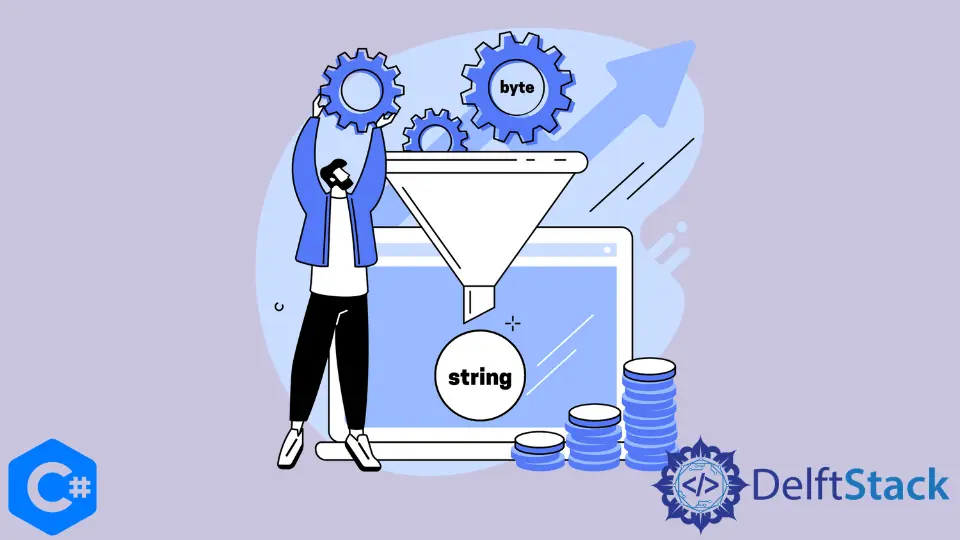
A byte array is an array of bytes. In C#, a byte array is used to store only positive values ranging from 0-255. Each element in the array has a memory space of 1 byte (8 bits
).
In C#, a byte array can be processed just like a normal array. It is interesting to know that a byte array can be converted to its equivalent string. A string can be converted to different encoding values and then stored in a byte array.
In this article, we are going to check out different methods to convert a byte array into a string. Let’s dive in.
Convert a Byte Array to a String
Using Encoding.GetString()
Method in C#
The method Encoding.GetString()
converts all bytes of a byte array into a string. This method belongs to the Encoding
class. This class has different encoding schemes like Unicode
, UTF8
, ASCII
, UTF32
, etc.
The correct syntax to use this method is as follows:
Encoding.Default.GetString(ByteArrayName);
Here Encoding.Default.GetString()
is used, it will convert the bytes of the byte array into the default type string. It will use the default encoding scheme.
Example Code:
using System;
using System.Text;
namespace Example {
class Conversion {
static void Main(string[] args) {
byte[] ByteArray = {
84, 104, 105, 115, 32, 105, 115, 32, 101, 120, 97, 109, 112, 108, 101,
};
Console.WriteLine("The Byte Array is: " + String.Join(" ", ByteArray));
string String = Encoding.Default.GetString(ByteArray);
Console.WriteLine("The String is: " + String);
}
}
}
Output:
The Byte Array is: 84 104 105 115 32 105 115 32 101 120 97 109 112 108 101
The String is: This is example
C# Program to Convert a Byte Array
to a String
Using MemoryStream
Method
In C#, MemoryStream
class is used to create a stream of data. This class belongs to System.IO
namespace. It can be used to convert a byte array to a string.
The correct syntax to use this method is as follows:
using (MemoryStream Stream = new MemoryStream(ByteArrayName)) {
using (StreamReader streamReader = new StreamReader(Stream)) {
return streamReader.ReadToEnd();
}
}
Here, we have created a MemoryStream
that contains the bytes of a specific byte array. Then, we have used a StreamReader
to read all the bytes from MemoryStream
and return the stream as a string.
We have created a customized method named BytesToStringConversion()
that accepts a byte array as a parameter and then returns a string.
Example Code:
using System;
using System.Text;
using System.IO;
namespace Example {
class Conversion {
static string BytesToStringConversion(byte[] bytes) {
using (MemoryStream Stream = new MemoryStream(bytes)) {
using (StreamReader streamReader = new StreamReader(Stream)) {
return streamReader.ReadToEnd();
}
}
}
static void Main(string[] args) {
byte[] ByteArray = {
84, 104, 105, 115, 32, 105, 115, 32, 101, 120, 97, 109, 112, 108, 101,
};
Console.WriteLine("The Byte Array is: " + String.Join(" ", ByteArray));
string str = BytesToStringConversion(ByteArray);
Console.WriteLine("The String is: " + str);
}
}
}
Output:
The Byte Array is: 84 104 105 115 32 105 115 32 101 120 97 109 112 108 101
The String is: This is example