How to Convert Stream to Byte Array in C#
-
Use
MemoryStream
to Convert Stream to Byte Array in C# -
Use
BinaryReader
to Convert Stream to Byte Array in C# -
Use
StreamReader
(For Text-Based Data) to Convert Stream to Byte Array in C# -
Use
BufferedStream
to Convert Stream to Byte Array in C# -
Use
FileStream
(For Reading From a File) to Convert Stream to Byte Array in C# - Conclusion
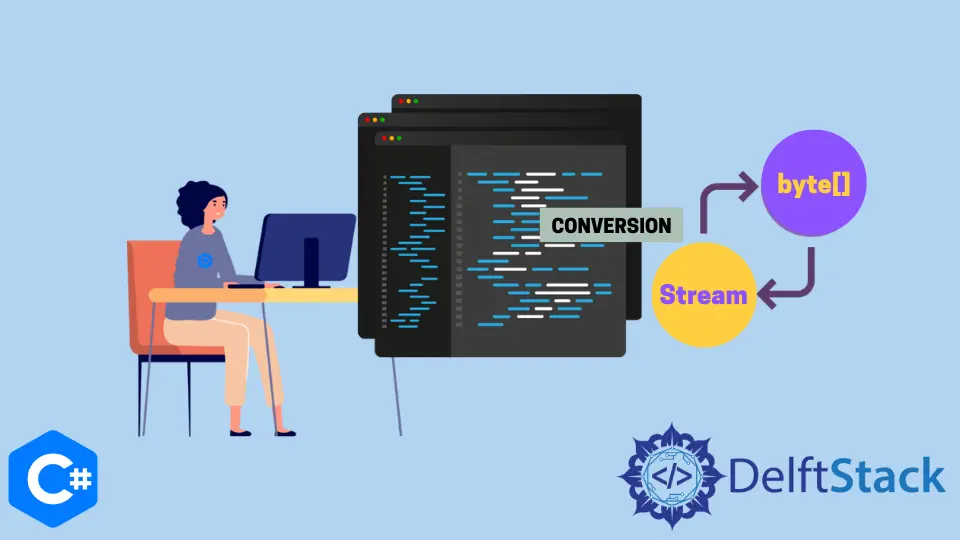
In C#, streams are the lifeblood of data handling. Whether you’re working with network data, file input/output, or any other form of data source, streams are the conduits through which your applications flow.
But what do you do when you need to convert that stream into a more manageable byte array? This article will discuss five different methods to convert streams into byte arrays in C#.
Use MemoryStream
to Convert Stream to Byte Array in C#
In C#, you can use the MemoryStream
class to convert a stream to a byte array. Here’s a step-by-step guide on how to do it:
-
Create the stream that you want to convert to a byte array. This could be a file stream, network stream, or any other type of stream.
For this example, let’s assume you have a
FileStream
:FileStream fileStream = new FileStream("example.txt", FileMode.Open);
-
Create a
MemoryStream
where you will store the data as a byte array:using (MemoryStream memoryStream = new MemoryStream()) { // Copy the data from the source stream to the memory stream fileStream.CopyTo(memoryStream); // Convert the memory stream to a byte array byte[] byteArray = memoryStream.ToArray(); // Now, byteArray contains the data from the source stream as a byte array // You can do whatever you want with the byteArray here }
-
Make sure to use the
using
statement with theMemoryStream
to ensure that it’s properly disposed of when you’re done with it. This will also flush and close theMemoryStream
.
In this example, we first create a MemoryStream
and then use the CopyTo
method to copy the data from the source stream (fileStream
) to the MemoryStream
. Finally, we use the ToArray
method of the MemoryStream
to obtain the byte array.
Use BinaryReader
to Convert Stream to Byte Array in C#
To convert a stream to a byte array using BinaryReader
in C#, you can follow these steps:
-
Create the stream that you want to convert to a byte array. This could be a file stream, network stream, or any other type of stream. For this example, let’s assume you have a
FileStream
:FileStream fileStream = new FileStream("example.txt", FileMode.Open);
-
Create a
BinaryReader
to read data from the source stream:using (BinaryReader reader = new BinaryReader(fileStream)) { // Read the data from the source stream into a byte array byte[] byteArray = reader.ReadBytes((int)fileStream.Length); // Now, byteArray contains the data from the source stream as a byte array // You can do whatever you want with the byteArray here }
-
Make sure to use the
using
statement with theBinaryReader
to ensure that it’s properly disposed of when you’re done with it. This will also close the underlyingFileStream
.
In this example, we create a BinaryReader
and then use its ReadBytes
method to read the entire content of the source stream (fileStream
) into a byte array. The length of the byte array is determined by the length of the source stream.
Use StreamReader
(For Text-Based Data) to Convert Stream to Byte Array in C#
If you have a text-based stream and you want to convert it to a byte array using a StreamReader
in C#, you can do so by first reading the text data and then encoding it into bytes using a specific character encoding, such as UTF-8. Here’s how you can do it:
// Create the stream that you want to convert to a byte array (e.g., FileStream or MemoryStream)
FileStream fileStream = new FileStream("example.txt", FileMode.Open);
// Create a StreamReader to read text data from the stream
using (StreamReader reader = new StreamReader(fileStream, Encoding.UTF8)) {
// Read the text data from the stream
string textData = reader.ReadToEnd();
// Convert the text data to bytes using UTF-8 encoding
byte[] byteArray = Encoding.UTF8.GetBytes(textData);
// Now, byteArray contains the text data from the stream as a byte array
// You can do whatever you want with the byteArray here
}
In this example:
-
We create a
StreamReader
and specify the character encoding (UTF-8) to use for reading text from the stream. -
We use the
ReadToEnd
method of theStreamReader
to read all the text data from the stream into a string (textData
). -
We then use
Encoding.UTF8.GetBytes
to convert the stringtextData
into a byte array. The byte array will contain the UTF-8 encoded representation of the text data.
Use BufferedStream
to Convert Stream to Byte Array in C#
You can use BufferedStream
to efficiently convert a stream to a byte array in C#. BufferedStream
provides buffering capabilities, which can improve performance when reading from or writing to a stream. Here’s how you can use it to convert a stream to a byte array:
// Create the stream that you want to convert to a byte array (e.g., FileStream or MemoryStream)
FileStream fileStream = new FileStream("example.txt", FileMode.Open);
// Create a BufferedStream for efficient reading
using (BufferedStream bufferedStream = new BufferedStream(fileStream)) {
// Create a MemoryStream to store the bytes
using (MemoryStream memoryStream = new MemoryStream()) {
// Read from the BufferedStream and write to the MemoryStream
byte[] buffer = new byte[4096]; // You can adjust the buffer size as needed
int bytesRead;
while ((bytesRead = bufferedStream.Read(buffer, 0, buffer.Length)) > 0) {
memoryStream.Write(buffer, 0, bytesRead);
}
// Convert the MemoryStream to a byte array
byte[] byteArray = memoryStream.ToArray();
// Now, byteArray contains the data from the source stream as a byte array
// You can do whatever you want with the byteArray here
}
}
In this example:
-
We create a
BufferedStream
to efficiently read from the source stream (fileStream
). You can adjust the buffer size as needed to match your requirements. -
We create a
MemoryStream
to store the bytes read from theBufferedStream
. -
We use a buffer (
byte[] buffer
) to read data from theBufferedStream
in chunks and write each chunk to theMemoryStream
. -
Finally, we use the
ToArray
method of theMemoryStream
to obtain the byte array containing the data from the source stream.
Use FileStream
(For Reading From a File) to Convert Stream to Byte Array in C#
To convert data from a FileStream
(used for reading from a file) to a byte array in C#, you can use the FileStream
and a MemoryStream
. Here’s a step-by-step guide on how to do it:
// Create a FileStream to read from a file (replace "example.txt" with your file path)
using (FileStream fileStream = new FileStream("example.txt", FileMode.Open, FileAccess.Read)) {
// Create a MemoryStream to store the byte data
using (MemoryStream memoryStream = new MemoryStream()) {
// Create a buffer to read data in chunks (adjust the buffer size as needed)
byte[] buffer = new byte[4096];
int bytesRead;
// Read data from the FileStream into the MemoryStream in chunks
while ((bytesRead = fileStream.Read(buffer, 0, buffer.Length)) > 0) {
memoryStream.Write(buffer, 0, bytesRead);
}
// Convert the MemoryStream to a byte array
byte[] byteArray = memoryStream.ToArray();
// Now, byteArray contains the data from the file as a byte array
// You can do whatever you want with the byteArray here
}
}
In this code:
-
We create a
FileStream
to read data from the file. Replace"example.txt"
with the path to your actual file. -
We create a
MemoryStream
to store the byte data that will be read from theFileStream
. -
We use a buffer (
byte[] buffer
) to read data from theFileStream
in chunks (adjust the buffer size as needed). -
We read data from the
FileStream
into theMemoryStream
in a loop until the entire file has been read. -
Finally, we use the
ToArray
method of theMemoryStream
to obtain the byte array containing the data from the file.
Make sure to handle exceptions and close/dispose of the streams appropriately in a production code context.
Conclusion
In C#, the ability to convert streams into byte arrays is an essential skill. Whether it’s binary or text data, large or small streams, or data from files, you now have the knowledge to efficiently transform streams into byte arrays.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn