Multiple Case Switch Statement in C#
-
Create Multiple Case Switch Statement in
C#
-
Create Multiple Case Switch Statement With Ranged Cases in
C#
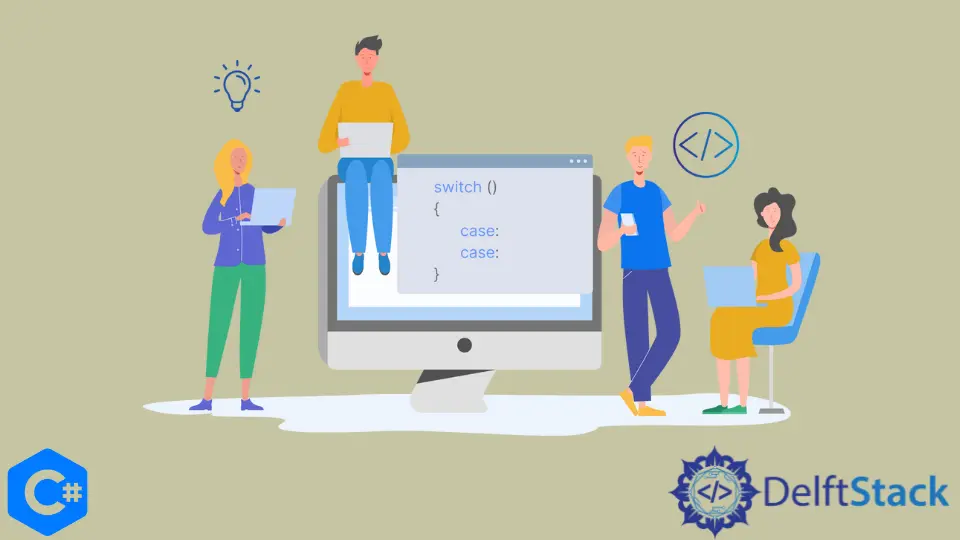
This tutorial will introduce methods to create a multiple-case switch statement in C#.
Create Multiple Case Switch Statement in C#
A switch
statement is a selection structure that is used to select one particular case from a range of cases based on some conditions. If we have a variable x
and we want to display The value is between 1 and 3
when the value of x
is 1
, 2
, or 3
, we have to write the conventional switch
statement as shown in the code example below.
using System;
namespace multiple_case_switch {
class Program {
static void Main(string[] args) {
int x = 3;
switch (x) {
case 1:
case 2:
case 3:
Console.WriteLine("The value is between 1 and 3");
break;
case 4:
case 5:
case 6:
Console.WriteLine("The value is between 4 and 6");
break;
}
}
}
}
Output:
The value is between 1 and 3
In the above code, we created a multiple case switch
statement that prints The value is between 1 and 3
for the values of x
between 1
and 3
and prints The value is between 4 and 6
if the value of x
is between 4
and 6
. This approach is alright if there is a small number of case labels. But for a large number of case labels, this method is not recommended because it is very labor-intensive and takes a lot of time.
Create Multiple Case Switch Statement With Ranged Cases in C#
The ranged case labels are used to perform an action for a range of values in C#. We can use the ranged case labels to achieve the same goal as the previous example. The when
keyword is used to specify a condition inside the case label to make it a ranged case in C#. The following code example shows us how we can use the ranged case label to create a multiple case switch
statement in C#.
using System;
namespace multiple_case_switch {
class Program {
static void method2() {}
static void Main(string[] args) {
int x = 5;
switch (x) {
case int n when (n >= 1 && n >= 3):
Console.WriteLine("The value is between 1 and 3");
break;
case int n when (n >= 4 && n <= 6):
Console.WriteLine("The value is between 4 and 6");
break;
}
}
}
}
Output:
The value is between 4 and 6
In the above code, we created a multiple case switch
statement that prints The value is between 1 and 3
for the values of x
between 1
and 3
and prints The value is between 4 and 6
if the value of x
is between 4
and 6
. We used the when
keyword to specify the condition that our value has to satisfy before executing the case label. This approach is preferred over the previous approach for a large number of case labels because we can specify a large range of values inside a single case label.a
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn