How to Convert Boolean to Integer in C#
-
Use the
ConvertToInt32
Statement to Convert Boolean to Integer in C# - Use the Ternary Conditional Operator to Convert Boolean to Integer in C#
-
Use the
if
Statement to Convert From Boolean to Integer in C# - Conclusion
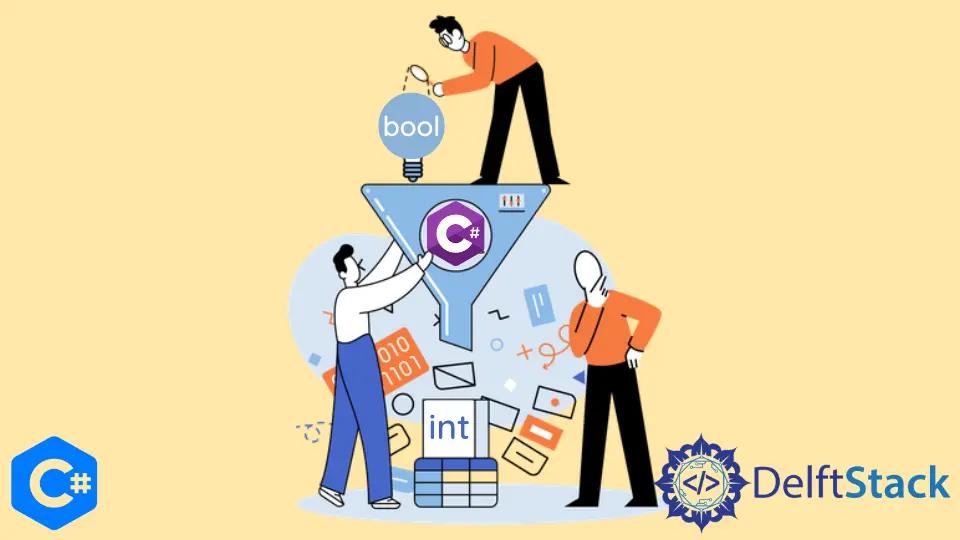
In C#, converting a boolean value to an integer is a common task, and several approaches can be employed to achieve this conversion.
This article will introduce distinct methods of converting boolean data type to integer in C#.
Each method is presented with comprehensive examples to demonstrate their application and efficiency. By exploring these techniques, developers can make informed decisions based on their specific requirements and coding preferences.
Use the ConvertToInt32
Statement to Convert Boolean to Integer in C#
Traditionally, there is no implicit conversion of data type from a boolean to an integer. However, the Convert.ToInt32()
method converts a specified value to a 32-bit signed integer.
It is worth mentioning that the Convert.ToInt32()
method is similar to the int.Parse()
method, but the int.Parse()
method only accepts string data type as an argument and throws an error when a null is passed as argument.
In comparison, the Convert.ToInt32()
method is not affected by these limitations. Further discussion is available via this reference.
In this article, we will explore the straightforward and efficient approach of using the Convert.ToInt32
method in C# to achieve this conversion.
using System;
class Program {
static void Main() {
// Declare a boolean variable
bool myBool = true;
// Use Convert.ToInt32 method to convert boolean to integer
int myInt = Convert.ToInt32(myBool);
// Display the result
Console.WriteLine($"Converted Integer: {myInt}");
}
}
We start by declaring a boolean variable myBool
and assigning it the value true
. This boolean variable represents a condition or a state that we want to convert into its integer equivalent.
The crucial step in this conversion is performed by the Convert.ToInt32
method. This method is part of the System
namespace and is designed to handle various data type conversions.
In our case, it takes a boolean value as an argument and returns the corresponding integer representation.
The converted integer is then stored in the variable myInt
. Finally, we display the result using Console.WriteLine
, providing a clear output for further analysis.
Output:
Converted Integer: 1
Use the switch
Statement to Convert Boolean to Integer in C#
The switch
statement is used to conditionally branch during the execution of a program. It decides the code block to be executed.
The value of the expression is compared with the values of each case.
If there is a match, the associated block of code is executed. The switch
expression is evaluated once.
Let’s start by examining a complete working example.
using System;
class Program {
static void Main() {
// Declare a boolean variable
bool myBool = true;
// Declare an integer variable to store the result
int myInt;
// Implement a switch statement for boolean to integer conversion
switch (myBool) {
case true:
myInt = 1;
break;
case false:
myInt = 0;
break;
default:
// Handle unexpected case if needed
myInt = -1;
break;
}
// Display the result
Console.WriteLine($"Converted Integer: {myInt}");
}
}
We begin by declaring a boolean variable, myBool
, and assigning it the value true
. This boolean variable represents a condition or state that we wish to translate into an integer equivalent.
To accomplish this conversion, we employ a switch statement. The switch statement provides a clear and structured way to handle multiple conditions.
In our case, it evaluates the boolean variable myBool
and executes the corresponding case.
The first case checks if myBool
is true
. If true, it assigns the value 1
to the integer variable myInt
.
The second case handles the scenario when myBool
is false
, assigning 0
to myInt
. The default case is included to handle unexpected situations`; although, in this specific conversion, it may not be necessary.
After the switch
statement, the result is displayed using Console.WriteLine
, providing immediate visibility into the converted integer value.
Output:
Converted Integer: 1
Use the Ternary Conditional Operator to Convert Boolean to Integer in C#
The conditional operator ?:
, also known as the Ternary Conditional Operator, is similar to the if
statement. It evaluates a Boolean expression and returns the result of one of two expressions.
If the Boolean expression is true, the first statement is returned (i.e., statement after the ?
); the second statement is returned (i.e., statement after the :
). Further discussion is available via this reference.
Let’s dive into a complete working example.
using System;
class Program {
static void Main() {
// Declare a boolean variable
bool myBool = false;
// Use the ternary conditional operator for boolean to integer conversion
int myInt = myBool ? 1 : 0;
// Display the result
Console.WriteLine($"Converted Integer: {myInt}");
}
}
We start by declaring a boolean variable named myBool
and assign it the value false
. This boolean variable represents a condition or state that we want to convert into an integer equivalent.
The crux of the conversion lies in a single line of code that uses the ternary conditional operator (? :
). This operator is a concise way to express a conditional statement, and it is particularly useful when the result of the condition is assigned to a variable.
In our case, the condition being evaluated is myBool
. If myBool
is true
, the operator returns 1
; otherwise, it returns 0
.
The result of this operation is then assigned to the integer variable myInt
.
To conclude, the converted integer value is displayed using Console.WriteLine
, providing immediate visibility into the result of the boolean to integer conversion.
Output:
Converted Integer: 0
Use the if
Statement to Convert From Boolean to Integer in C#
The if
statement checks whether or not a specific condition is true or false after executing some logical expression. Whenever the expression returns true
, the numeric value 1 is returned.
Similarly, when the numeric value is 1, the Boolean value true
is returned. Else, false
is returned.
Let’s explore a complete working example.
using System;
class Program {
static void Main() {
// Declare a boolean variable
bool myBool = false;
// Declare an integer variable to store the result
int myInt;
// Implement an if statement for boolean to integer conversion
if (myBool) {
myInt = 1;
} else {
myInt = 0;
}
// Display the result
Console.WriteLine($"Converted Integer: {myInt}");
}
}
We begin by declaring a boolean variable named myBool
and assign it the value false
. This boolean variable represents a condition or state that we aim to convert into an integer equivalent.
The core of the conversion process lies in the if
statement. The if
statement evaluates the boolean expression within its parentheses, and if the expression is true, the code block following the if
keyword is executed.
If myBool
is true
, the code within the if
block assigns 1
to the integer variable myInt
. Conversely, if myBool
is false
, the else
block is executed, assigning 0
to myInt
.
Finally, the converted integer value is displayed using Console.WriteLine
, providing immediate visibility into the result of the boolean to integer conversion.
Output:
Converted Integer: 0
Conclusion
This article has covered multiple strategies for converting boolean values to integers in C#, showcasing the flexibility and versatility of the language. Whether developers prefer the simplicity of the Convert.ToInt32
method, the structured approach of the switch
statement, the concise syntax of the Ternary Conditional Operator, or the classic logic of the if
statement, they now have a range of tools at their disposal.
Choosing the right method depends on the specific context and the developer’s coding style, ensuring that the code remains both functional and easily maintainable.