C#에서 부울을 정수로 변환
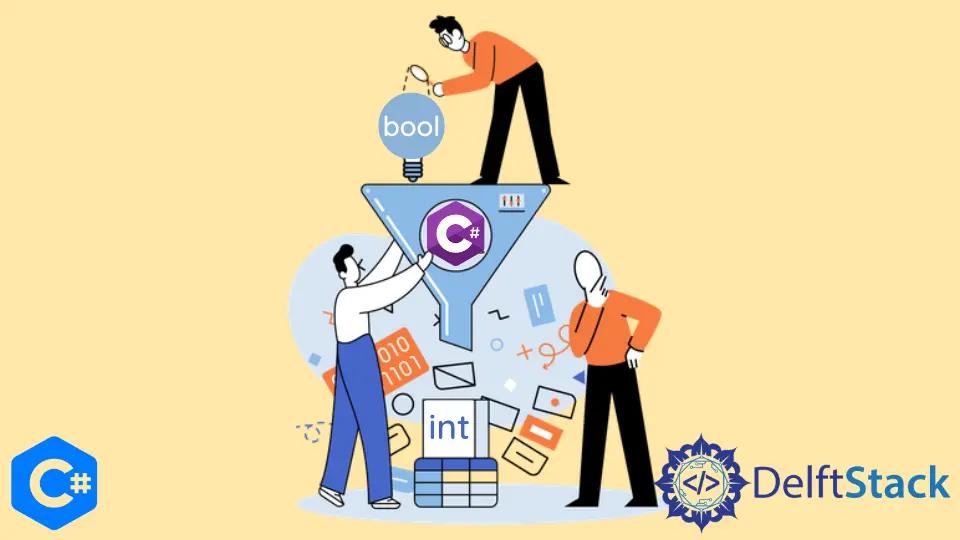
이 기사에서는 C#에서 부울 데이터 유형을 정수로 변환하는 방법을 소개합니다.
C#
에서 ConvertToInt32
문을 사용하여 부울을 정수로 변환
전통적으로 부울에서 정수로 데이터 유형의 암시적 변환이 없습니다. 그러나 Convert.ToInt32()
메서드는 지정된 값을 32비트 부호 있는 정수로 변환합니다.
Convert.ToInt32()
메소드는 int.Parse()
메소드와 유사하지만 int.Parse()
메소드는 문자열 데이터 유형만 인수로 받아들이고 오류가 발생한다는 점을 언급할 가치가 있습니다. null이 인수로 전달될 때.
이에 비해 Convert.ToInt32()
메서드는 이러한 제한 사항의 영향을 받지 않습니다.
추가 논의는 이 참조를 통해 가능합니다.
다음은 Convert.ToInt32()
메서드를 사용한 코드의 예입니다.
// C# program to illustrate the
// use of Convert.ToInt32 statement
// and Convert.ToBoolean
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = Convert.ToInt32(boolinput);
bool boolRresult = Convert.ToBoolean(intRresult);
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolRresult);
}
}
출력:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
다음은 int.Parse()
메서드를 사용한 코드의 예입니다.
// C# program to illustrate the
// use of int.Parse statement
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = int.Parse(boolinput);
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
}
}
출력:
program.cs(12,30): error CS1503: Argument 1: cannot convert from 'bool' to 'string'
위의 오류는 부울
데이터 유형을 정수
로 변환하기 위해 int.Parse
메서드를 사용하여 표시됩니다. 메소드는 Boolean
데이터 유형이 아니라 string
을 인수로 예상하고 있습니다.
C#
에서 switch
문을 사용하여 부울을 정수로 변환
switch
문은 프로그램 실행 중 조건부 분기에 사용됩니다. 실행할 코드 블록을 결정합니다. 식의 값을 각 경우의 값과 비교하고,
일치하는 항목이 있으면 연결된 코드 블록이 실행됩니다. switch
표현식은 한 번 평가됩니다.
다음은 switch
문을 사용한 코드의 예입니다.
// C# program to illustrate the
// use of switch statement
using System;
namespace Test {
class Program {
static void Main(string[] args) {
int i = 1;
bool b = true;
switch (i) {
case 0:
b = false;
Console.WriteLine("When integer is 0, boolean is:");
Console.WriteLine(b);
break;
case 1:
b = true;
Console.WriteLine("When integer is 1, boolean is:");
Console.WriteLine(b);
break;
}
switch (b) {
case true:
i = 1;
Console.WriteLine("When boolean is true, integer is:");
Console.WriteLine(i);
break;
case false:
i = 0;
Console.WriteLine("When boolean is false, integer is:");
Console.WriteLine(i);
break;
}
}
}
}
출력:
When an integer is 1, boolean is:
True
When boolean is true, integer is:
1
삼항 조건부 연산자를 사용하여 C#
에서 부울을 정수로 변환
삼항 조건 연산자라고도 하는 조건 연산자 ?:
는 if
문과 유사합니다. 부울 식을 평가하고 두 식 중 하나의 결과를 반환합니다.
부울 표현식이 참이면 첫 번째 명령문이 반환됩니다(즉, ?
뒤의 명령문). 두 번째 명령문이 반환됩니다(즉, :
뒤의 명령문). 추가 논의는 이 참조를 통해 가능합니다.
아래는 코드의 예입니다.
// C# program to illustrate the
// use of ternary operator
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = boolinput ? 1 : 0;
bool boolRresult = (intRresult == 1) ? true : false;
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolRresult);
}
}
출력:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
if
문을 사용하여 C#
에서 부울에서 정수로 변환
if
문은 일부 논리식을 실행한 후 특정 조건이 참인지 거짓인지 확인합니다. 표현식이 true
를 반환할 때마다 숫자 값 1, 0이 반환됩니다.
마찬가지로 숫자 값이 1이면 부울 값 true
가 반환됩니다. 그렇지 않으면 false
가 반환됩니다.
아래는 코드의 예입니다.
// C# program to illustrate the
// use of if statement
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intResult;
if (boolinput) {
intResult = 1;
} else {
intResult = 0;
}
bool boolResult;
if (intResult == 1) {
boolResult = true;
} else {
boolResult = false;
}
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intResult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolResult);
}
}
출력:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True