C# Int를 문자열로 변환
-
C#
int
를string
으로 변환-Int16.ToString()
/Int32.ToString()
/Int64.ToString()
메소드 -
C#
int
를string
으로 변환-Convert.ToString()
메소드 -
C#
int
를string
으로 변환-String.Format()
메소드 -
C#
int
에서string
로의 변환-StringBuilder
메소드 -
C#
int
를string
으로 변환-+
연산자 사용
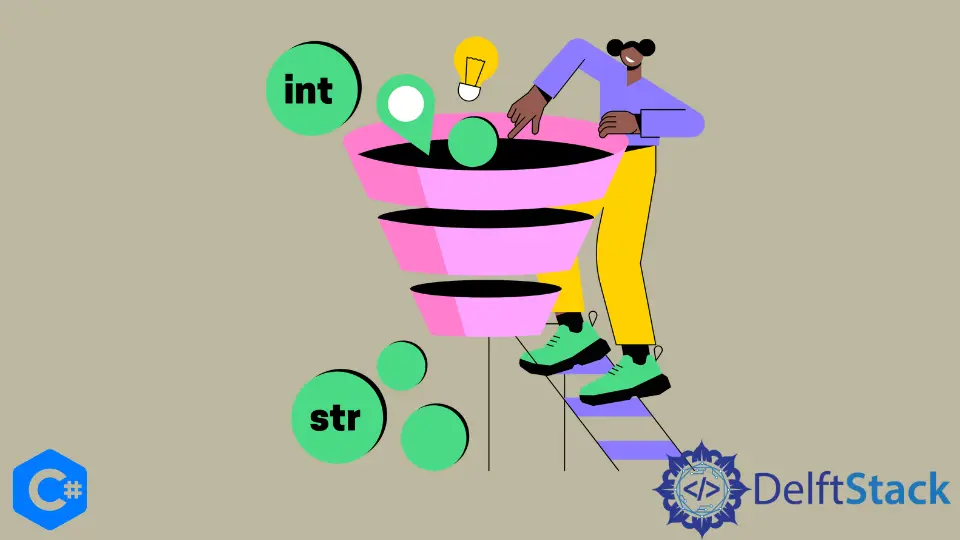
C#에는 int를 문자열로 변환하는 다른 방법이 있습니다. 이 기사에서는ToString
메소드,Convert.ToString
메소드, 문자열 형식화 및StringBuilder
메소드와 같은 메소드를 소개합니다.
C#int
를string
으로 변환-Int16.ToString()
/Int32.ToString()
/Int64.ToString()
메소드
Int16/32/64
데이터 타입의ToString()
메소드는 정수를 문자열 표현으로 변환하며 주로 디스플레이 목적으로 사용됩니다.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = num.ToString();
System.Console.WriteLine(numString);
}
}
출력:
80
C#int
를string
으로 변환-Convert.ToString()
메소드
System
네임 스페이스의Convert
클래스는 데이터 유형을 다른 데이터 유형으로 변환합니다. Convert.ToString()
메소드는 주어진 값을 문자열 표현으로 변환합니다.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = Convert.ToString(num);
System.Console.WriteLine(numString);
}
}
출력:
80
C#int
를string
으로 변환-String.Format()
메소드
String.Format
메소드는 지정된 형식에 따라 주어진 객체를 문자열로 변환합니다.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = string.Format("{0}", num);
System.Console.WriteLine(numString);
}
}
여기서{0}
은 형식 항목이고0
은 해당 위치에 문자열 표현이 삽입 된 객체의 시작 색인입니다.
C#int
에서string
로의 변환-StringBuilder
메소드
System.Text
네임 스페이스의StringBuilder
는 변경 가능한 문자열입니다. StringBuilder
객체는 문자열에 더 많은 문자를 추가하기 위해 버퍼를 유지합니다.
using System;
using System.Text;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = new StringBuilder().Append(num).ToString();
System.Console.WriteLine(numString);
}
}
StringBuilder
의 인수가 비어 있으면 String.Empty
값으로 StringBuilder
를 인스턴스화합니다.
Append(num)
은num
의 문자열 표현을StringBuilder
에 추가합니다.
ToString()
메소드는 StringBuilder
유형을 string
으로 변환합니다.
C#int
를string
으로 변환-+
연산자 사용
string
변수와int
변수가+
연산자에 의해 추가되면, 자동으로int.ToString()
메소드를 호출하여 정수를 주어진string
변수와 연결될 문자열로 변환합니다.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = "" + num;
System.Console.WriteLine(numString);
}
}
출력:
80
string numString = "" + num;
+
연산자의 다른 변수가 빈 문자열 인 경우에만int
를string
으로 변환합니다-""
또는String.Empty
.
""
는int
앞이나int
뒤에있을 수 있습니다. 두 동작 모두 동일합니다.
using System;
public class Demo {
public static void Main() {
// Your code here!
int num = 80;
string numString = num + "";
System.Console.WriteLine(numString);
}
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook