How to Convert Int to Enum in C#
- Use Direct Casting to Convert an Int to Enum in C#
-
Use the
Enum.Parse
Method to Convert an Int to Enum in C# -
Use the
Enum.IsDefined
Method to Convert an Int to Enum in C# -
Use the
Enum.TryParse
Method to Convert an Int to Enum in C# - Conclusion
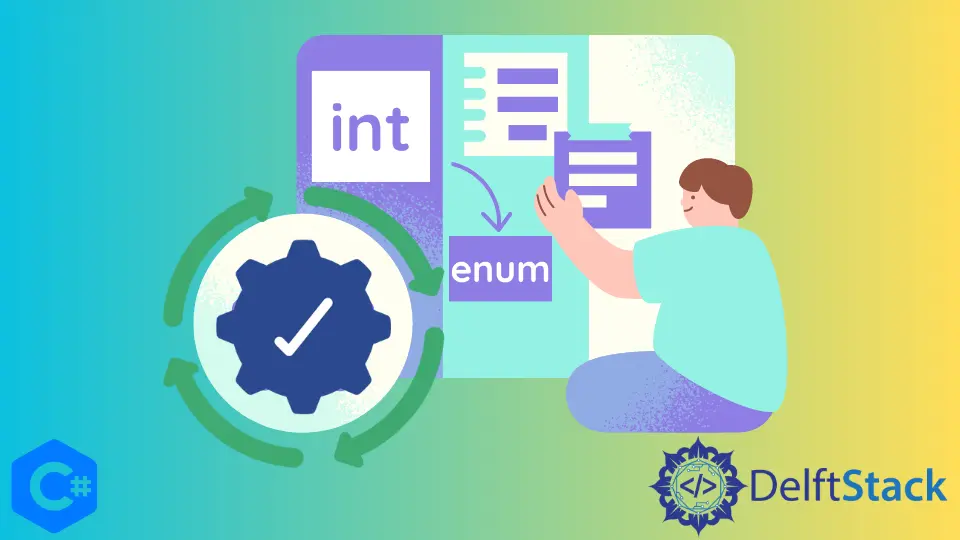
Working with enumerations (enums) in C# provides a powerful way to define named integral constants, giving code clarity and enhancing readability. However, situations may arise where you need to convert an integer to an enum, especially when dealing with external data or dynamic inputs.
In this article, we’ll explore several methods to achieve this conversion in C#.
Use Direct Casting to Convert an Int to Enum in C#
One straightforward and commonly used approach to convert an integer to an enum type is through direct casting. This method involves casting the integer directly to the enum type, assuming the integer value corresponds to a valid enum value.
The syntax for direct casting an integer to an enum in C# is as follows:
YourEnumType enumVariable = (YourEnumType)intValue;
Here, YourEnumType
represents the enum type, and intValue
is the integer value you want to convert to the enum type. The (YourEnumType)
part denotes the explicit cast, informing the compiler that you are converting the integer to the specified enum type.
Let’s now dive into a practical example to demonstrate the process of converting an int to an enum using direct casting.
using System;
public class Program {
public enum MyEnum { Zero = 0, One = 1, Two = 2 }
public static void Main() {
int intValue = 2;
MyEnum enumValue = (MyEnum)intValue;
Console.WriteLine($"Converted Enum Value: {enumValue}");
}
}
In the provided code example, we begin by defining an enumeration called MyEnum
within the Program
class, comprising three constant values: Zero
, One
, and Two
. These constants represent the possible states or values that our enum type can take.
Moving into the Main
method, we initialize an integer variable named intValue
with a value of 2
. This integer is the value we intend to convert to the enum type.
The crux of the conversion occurs with the use of direct casting, denoted by (MyEnum)intValue
. This expression explicitly instructs the compiler to convert the integer value to the specified enum type, MyEnum
.
The result of this cast is then stored in the variable enumValue
.
Output:
Converted Enum Value: Two
In this case, the integer value 2
has been successfully converted to the enum constant Two
using direct casting.
Use the Enum.Parse
Method to Convert an Int to Enum in C#
An alternative method to convert an integer to an enum is by using the Enum.Parse
method. This approach is particularly useful when the integer value is dynamic and needs to be converted to an enum at runtime.
The Enum.Parse
method allows us to convert a string representation or a numeric value to an enum. The syntax is as follows:
YourEnumType enumVariable = (YourEnumType)Enum.Parse(typeof(YourEnumType), stringValueOrIntValue);
Here, YourEnumType
represents the enum type, and stringValueOrIntValue
is either the string representation or the integer value that you want to convert to the enum type. The typeof(YourEnumType)
part specifies the target enum type for parsing.
Let’s now explore a practical example demonstrating the use of the Enum.Parse
method to convert an integer to an enum:
using System;
public class Program {
public enum MyEnum { Zero = 0, One = 1, Two = 2 }
public static void Main() {
int intValue = 1;
MyEnum enumValue = (MyEnum)Enum.Parse(typeof(MyEnum), intValue.ToString());
Console.WriteLine($"Converted Enum Value: {enumValue}");
}
}
To begin, we define an enum named MyEnum
within the Program
class, outlining constant values Zero
, One
, and Two
that represent the possible states or values of our enum type.
As we step into the Main
method, we initialize an integer variable, intValue
, with a value of 1
. This integer serves as the dynamic value we aim to convert to the enum type at runtime.
The crux of the conversion lies in the Enum.Parse
method: (MyEnum)Enum.Parse(typeof(MyEnum), intValue.ToString())
. Here, the integer is converted to its string representation, and the Enum.Parse
method dynamically parses it into the specified MyEnum
enum type.
The result of this parse operation is stored in the variable enumValue
. Subsequently, we print the converted enum value to the console using Console.WriteLine($"Converted Enum Value: {enumValue}")
.
Output:
Converted Enum Value: One
Here, the integer value 1
has been successfully converted to the enum constant One
using the Enum.Parse
method. This method proves particularly useful when dealing with dynamic or user-input values that need to be converted to enum types at runtime.
Use the Enum.IsDefined
Method to Convert an Int to Enum in C#
When tasked with converting an integer to an enum in C#, another method we can use is the Enum.IsDefined
method. This method allows us to check if an integer corresponds to a defined enum value before performing the conversion.
The syntax is as follows:
if (Enum.IsDefined(typeof(YourEnumType), intValue)) {
YourEnumType enumVariable = (YourEnumType)intValue;
}
Here, YourEnumType
represents the enum type, and intValue
is the integer value you want to convert.
The method returns true
if the value is a named constant in the specified enumeration; otherwise, it returns false
. If the value is defined, we proceed with the direct casting conversion.
Let’s delve into a practical example demonstrating the use of the Enum.IsDefined
method to convert an integer to an enum:
using System;
public class Program {
public enum MyEnum { Zero = 0, One = 1, Two = 2 }
public static void Main() {
int intValue = 2;
if (Enum.IsDefined(typeof(MyEnum), intValue)) {
MyEnum enumValue = (MyEnum)intValue;
Console.WriteLine($"Converted Enum Value: {enumValue}");
} else {
Console.WriteLine("Invalid Enum Value");
}
}
}
Here, we introduce a robust method for converting an integer to an enum in C# using the Enum.IsDefined
method. First, we define an enum named MyEnum
within the Program
class, outlining constant values Zero
, One
, and Two
to represent potential states or values of our enum type.
As we transition into the Main
method, an integer variable, intValue
, is initialized with a value of 2
. This integer serves as the candidate for conversion to the enum type.
The important step involves using the Enum.IsDefined
method to check if intValue
corresponds to a defined enum value in MyEnum
. If the condition is met, indicating a valid enum value, we proceed with the conversion using direct casting: (MyEnum)intValue
.
Importantly, we also account for the scenario where the integer value is not a valid enum constant. In such cases, we print Invalid Enum Value
to notify the user that the conversion is not possible.
Output:
Converted Enum Value: Two
This method proves useful for ensuring that the integer value corresponds to a valid enum value before proceeding with the conversion.
Use the Enum.TryParse
Method to Convert an Int to Enum in C#
Another method for converting an integer to an enum is through the use of Enum.TryParse
. This method is particularly advantageous as it not only performs the conversion but also checks whether the conversion is successful, avoiding potential exceptions.
The Enum.TryParse
method attempts to convert the string representation of an enum constant to its equivalent. The syntax is as follows:
if (Enum.TryParse<YourEnumType>(stringValue, out YourEnumType enumVariable)) {
// Conversion successful, use enumVariable
} else {
// Handle the case where the conversion is not successful
}
Here, YourEnumType
represents the enum type, and stringValue
is the string representation of the enum constant. The method returns true
if the conversion is successful, and the result is stored in the enumVariable
.
If the conversion fails, it returns false
, allowing you to handle such cases gracefully.
Let’s dive into a practical example demonstrating the use of the Enum.TryParse
method to convert an integer to an enum:
using System;
public class Program {
public enum MyEnum { Zero = 0, One = 1, Two = 2 }
public static void Main() {
int intValue = 1;
MyEnum enumValue;
if (Enum.TryParse<MyEnum>(intValue.ToString(), out enumValue)) {
Console.WriteLine($"Converted Enum Value: {enumValue}");
} else {
Console.WriteLine("Invalid Enum Value");
}
}
}
In this code, we introduce a powerful method for converting an integer to an enum in C# using the Enum.TryParse
method. Initially, we define an enum named MyEnum
within the Program
class, outlining constant values Zero
, One
, and Two
that represent potential states or values of our enum type.
As we step into the Main
method, an integer variable, intValue
, is initialized with a value of 1
. This integer serves as the candidate for conversion to the enum type. We declare a variable enumValue
of type MyEnum
to capture the result of the conversion.
Here, the key step is the use of the Enum.TryParse
method. We convert the integer to its string representation and attempt to parse it into the MyEnum
enum type. If successful, the result is stored in enumValue
.
To handle the outcome, we utilize an if-else
statement. If the conversion succeeds, we print the converted enum value to the console; otherwise, we print the Invalid Enum Value
to display that the conversion did not occur.
Output:
Converted Enum Value: One
The Enum.TryParse
method provides a reliable and safe way to handle enum conversions, ensuring a smooth process and allowing for proper handling of cases where the conversion is not successful.
Conclusion
These diverse methods for converting an integer to an enum in C# offer us flexibility and adaptability to various scenarios. Direct casting proves straightforward when dealing with known and fixed values, while Enum.Parse
accommodates dynamic or user-provided inputs.
Enum.IsDefined
adds a layer of validation, ensuring the integer corresponds to a defined enum value. Enum.TryParse
offers safety by avoiding exceptions, making it ideal for handling uncertain conversions.
The selection of a method hinges on factors such as input variability, validation needs, and the nature of the enum and integer values involved.
Related Article - Csharp Integer
- How to Convert Int to String in C#
- Random Int in C#
- Random Number in a Range in C#
- How to Convert String to Int in C#
- Integer Division in C#