How to Enumerate an Enum in C#
-
Enum.GetNames()
Method to Enumerateenum
-
Enum.GetValues()
Method to Enumerateenum
inC#
- More Examples
- Remarks:
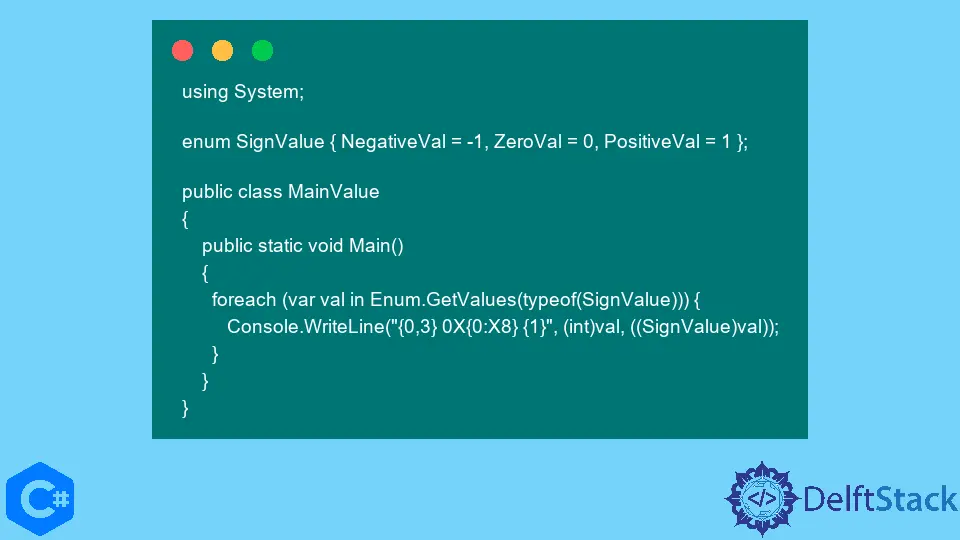
This tutorial introduces how to enumerate an enum
type in C# and demonstrates it through some list of code examples.
In the previous tutorial, we have learned how to retrieve int
value from enum
using C#. As mentioned in the last tutorial, Enumeration
or enum
is a user-defined value type that defines a set of named constants of the underlying integer type.
We know the common syntax for enum
is as follows:
enum<enum_name> { enumeration_list }
;
enum_name
specifies the name of the enumeration type, and enumeration_list
specifies a list of comma-separated identifiers.
Next, we will see how to loop through or enumerate all enum
values in C#. There are several ways to enumerate an enum
. The following examples will illustrate these ways.
Enum.GetNames()
Method to Enumerate enum
The aim is to get an array of names of the constants in the enumeration list
using the Enum.GetName()
method. It prints the array of names using the foreach
loop.
using System;
public class Example1 {
public enum Fruits { Apple, Banana, Orange, Watermelon, Mango, Strawberry }
public static void Main() {
foreach (string fruit in Enum.GetNames(typeof(Fruits))) {
Console.WriteLine(fruit);
}
}
}
Output:
Apple
Banana
Orange
Watermelon
Mango
Strawberry
Enum.GetValues()
Method to Enumerate enum
in C#
Another approach is to use the Enum.GetValues()
method that retrieves an array of the constants’ values in the enumeration list. It also prints each value using the foreach
loop.
using System;
public class Example2 {
public enum Subjects { Maths, Biology, English, Chemistry, Physics, History }
public static void Main() {
foreach (Subjects sub in Enum.GetValues(typeof(Subjects))) {
Console.WriteLine(sub);
}
}
}
Output:
Maths
Biology
English
Chemistry
Physics
History
Let’s discuss some more examples to get a complete picture of using the Enum.GetValues()
method.
More Examples
Example 1:
using System;
public class Demo1 {
enum Colors { White, Black, Red, Green, Blue }
;
enum Planets { Earth = 0, Jupiter = 10, Saturn = 20, Mars = 30, Venus = 40 }
;
public static void Main() {
Console.WriteLine("The list of Colors Enum are:");
foreach (int i in Enum.GetValues(typeof(Colors))) Console.WriteLine(i);
Console.WriteLine();
Console.WriteLine("The list of Planets Enum are:");
foreach (int i in Enum.GetValues(typeof(Planets))) Console.WriteLine(i);
}
}
Output:
The list of Colors Enum are:
0
1
2
3
4
The list of Planets Enum are:
0
10
20
30
40
In the first part of the above example, the array elements are sorted by the binary values (that is, the unsigned values) of the enumeration
constants.
Example 2:
using System;
enum SignValue { NegativeVal = -1, ZeroVal = 0, PositiveVal = 1 }
;
public class MainValue {
public static void Main() {
foreach (var val in Enum.GetValues(typeof(SignValue))) {
Console.WriteLine("{0,3} 0X{0:X8} {1}", (int)val, ((SignValue)val));
}
}
}
Output:
0 ZeroVal 0X00000000
1 PositiveVal 0X00000001
-1 NegativeVal 0XFFFFFFFF
The above example shows information about the array returned by the Enum.GetValues()
method for the enumeration list that includes zero, negative and positive values.
Remarks:
The Enum.GetValues()
method returns an array that contains a value for each element of the enum
. if multiple elements have the same value, the generated array includes duplicate values. In this case, calling the Enum.GetNames()
method with each value in the returned array does not restore the unique names assigned to elements that have duplicate values. To retrieve all the enumeration members’ names successfully, we recommend calling the Enum.GetNames()
method.