How to Convert Enum to String in C#
-
Convert Enum to String With the
Description
Attribute inC#
-
Convert Enum to String With the
switch
Statement inC#
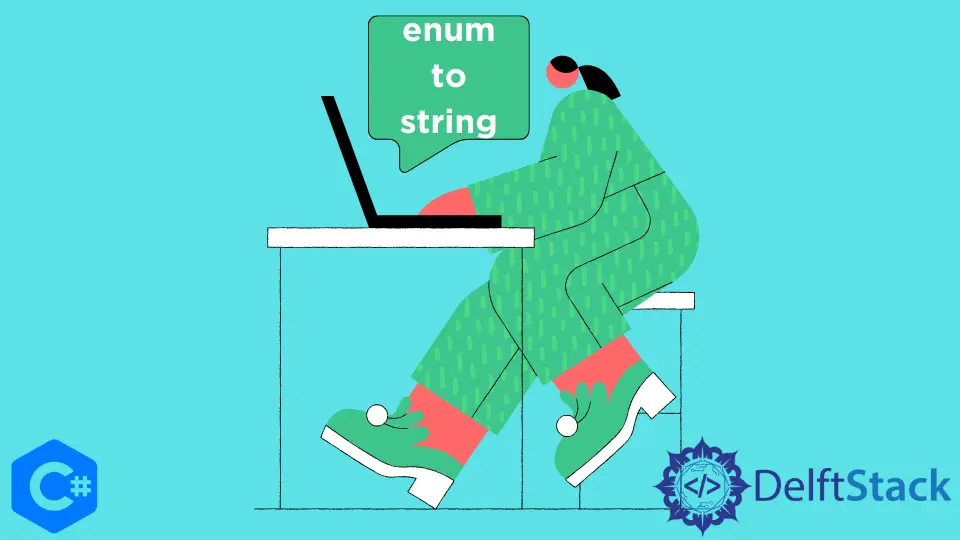
This tutorial will discuss the methods to convert an enum to a string in C#.
Convert Enum to String With the Description
Attribute in C#
We do not have to use anything to convert it to a string for a simple Enum value that follows the naming conventions. It can be displayed to the user with the Console.WriteLine()
function in C#. It is demonstrated in the coding example below.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace enum_to_string {
public enum Status { InProgress, Completed }
class Program {
static void Main(string[] args) {
Status complete = Status.Completed;
Console.WriteLine(complete);
}
}
}
Output:
Completed
In the above code, we directly printed the Enum value Completed
in string format in C#. This was possible because our Enum value followed the variable naming conventions in C#. But if we want to display a reader-friendly string, we have to use the Description
attribute of Enums in C#. The Description
attribute is used to describe each value of an Enum. We can convert our Enum to a reader-friendly string by writing the string inside the Description
attribute of the Enum. The following code example shows us how to convert an Enum value to a string with the Description
attribute in C#.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Reflection;
namespace enum_to_string {
public enum Status {
[Description("The Desired Task is InProgress")] InProgress,
[Description("The Desired Task is Successfully Completed")] Completed
}
static class extensionClass {
public static string getDescription(this Enum e) {
Type eType = e.GetType();
string eName = Enum.GetName(eType, e);
if (eName != null) {
FieldInfo fieldInfo = eType.GetField(eName);
if (fieldInfo != null) {
DescriptionAttribute descriptionAttribute = Attribute.GetCustomAttribute(
fieldInfo, typeof(DescriptionAttribute)) as DescriptionAttribute;
if (descriptionAttribute != null) {
return descriptionAttribute.Description;
}
}
}
return null;
}
}
class Program {
static void Main(string[] args) {
Status complete = Status.Completed;
string description = complete.getDescription();
Console.WriteLine(description);
}
}
}
Output:
The Desired Task is Successfully Completed
In the above code, we created an extension method, getDescription
, that returns the Enum value description in C#. This method works perfectly fine, but it is a little complex. This complexity is simplified in the next section.
Convert Enum to String With the switch
Statement in C#
A lot of complexity of the previous method can be simplified by using the switch
statement in C#. We can assign the desired value to our string variable for each Enum value with the switch
statement in C#. See the following code example.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Reflection;
namespace enum_to_string {
public enum Status { InProgress, Completed }
static class extensionClass {
public static string getValue(this Status e) {
switch (e) {
case Status.InProgress:
return "The Desired Task is InProgress";
case Status.Completed:
return "The Desired Task is Successfully Completed";
}
return String.Empty;
}
}
class Program {
static void Main(string[] args) {
Status complete = Status.Completed;
string value = complete.getValue();
Console.WriteLine(value);
}
}
}
Output:
The Desired Task is Successfully Completed
In the above code, we created an extension method, getValue()
, that returns a string based on the Enum value using the switch
statement in C#. The getValue()
function uses the switch
statement and returns a different string for each value of our specified Enum.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Enum
- How to Convert String to Enum in C#
- How to Convert Int to Enum in C#
- Enum Strings in C#
- How to Enumerate an Enum in C#
- How to Get Int Value From Enum in C#