C# で列挙型を文字列に変換する
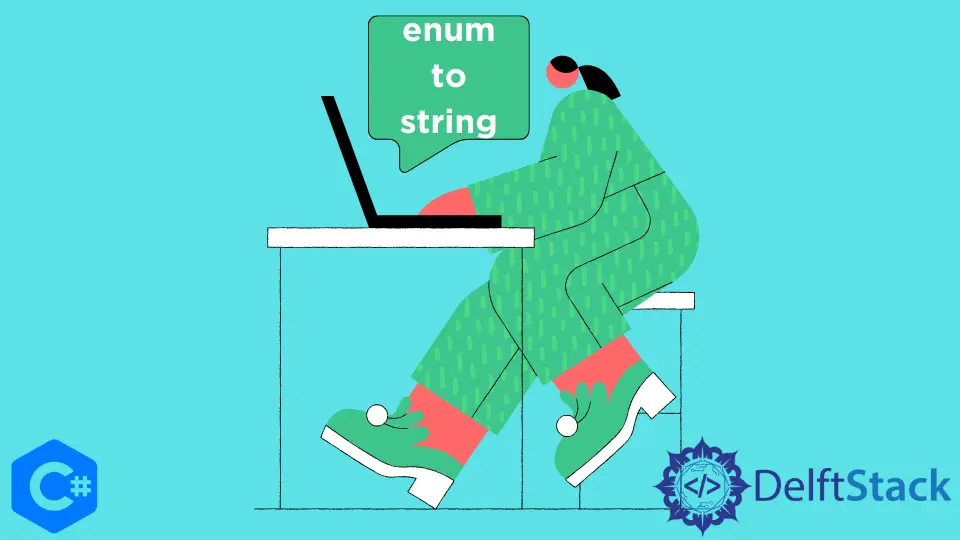
このチュートリアルでは、C# で列挙型を文字列に変換する方法について説明します。
C# の Description
属性を使用して列挙型を文字列に変換する
命名規則に従った単純な列挙値の文字列に変換するために何も使用する必要はありません。これは、C# の Console.WriteLine()
関数を使用してユーザーに表示できます。これは、以下のコーディング例に示されています。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace enum_to_string {
public enum Status { InProgress, Completed }
class Program {
static void Main(string[] args) {
Status complete = Status.Completed;
Console.WriteLine(complete);
}
}
}
出力:
Completed
上記のコードでは、列挙値 Completed
を文字列形式で C# で直接出力しました。これが可能だったのは、列挙値が C# の変数の命名規則に従っているためです。ただし、読みやすい文字列を表示する場合は、C# の Enums の Description
属性を使用する必要があります。Description
属性は、列挙型の各値を説明するために使用されます。列挙型の Description
属性内に文字列を書き込むことで、列挙型を読みやすい文字列に変換できます。次のコード例は、列挙型の値を C# の Description
属性を持つ文字列に変換する方法を示しています。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Reflection;
namespace enum_to_string {
public enum Status {
[Description("The Desired Task is InProgress")] InProgress,
[Description("The Desired Task is Successfully Completed")] Completed
}
static class extensionClass {
public static string getDescription(this Enum e) {
Type eType = e.GetType();
string eName = Enum.GetName(eType, e);
if (eName != null) {
FieldInfo fieldInfo = eType.GetField(eName);
if (fieldInfo != null) {
DescriptionAttribute descriptionAttribute = Attribute.GetCustomAttribute(
fieldInfo, typeof(DescriptionAttribute)) as DescriptionAttribute;
if (descriptionAttribute != null) {
return descriptionAttribute.Description;
}
}
}
return null;
}
}
class Program {
static void Main(string[] args) {
Status complete = Status.Completed;
string description = complete.getDescription();
Console.WriteLine(description);
}
}
}
出力:
The Desired Task is Successfully Completed
上記のコードでは、C# で列挙値の説明を返す拡張メソッド getDescription
を作成しました。この方法は完全にうまく機能しますが、少し複雑です。この複雑さは、次のセクションで簡略化されます。
C# の switch
ステートメントを使用して列挙型を文字列に変換する
C# で switch
ステートメントを使用すると、前の方法の多くの複雑さを単純化できます。C# の switch
ステートメントを使用して、各 Enum 値の文字列変数に目的の値を割り当てることができます。次のコード例を参照してください。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Reflection;
namespace enum_to_string {
public enum Status { InProgress, Completed }
static class extensionClass {
public static string getValue(this Status e) {
switch (e) {
case Status.InProgress:
return "The Desired Task is InProgress";
case Status.Completed:
return "The Desired Task is Successfully Completed";
}
return String.Empty;
}
}
class Program {
static void Main(string[] args) {
Status complete = Status.Completed;
string value = complete.getValue();
Console.WriteLine(value);
}
}
}
出力:
The Desired Task is Successfully Completed
上記のコードでは、C# の switch
ステートメントを使用して Enum 値に基づいて文字列を返す拡張メソッド getValue()
を作成しました。getValue()
関数は switch
ステートメントを使用し、指定された列挙型の値ごとに異なる文字列を返します。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn