Enum Strings in C#
-
Create Enumeration of Strings With Extension Function in
C#
-
Create Enumeration of Strings With Constant Variables in
C#
-
Create Enumeration of Strings With the
ToString()
Function inC#
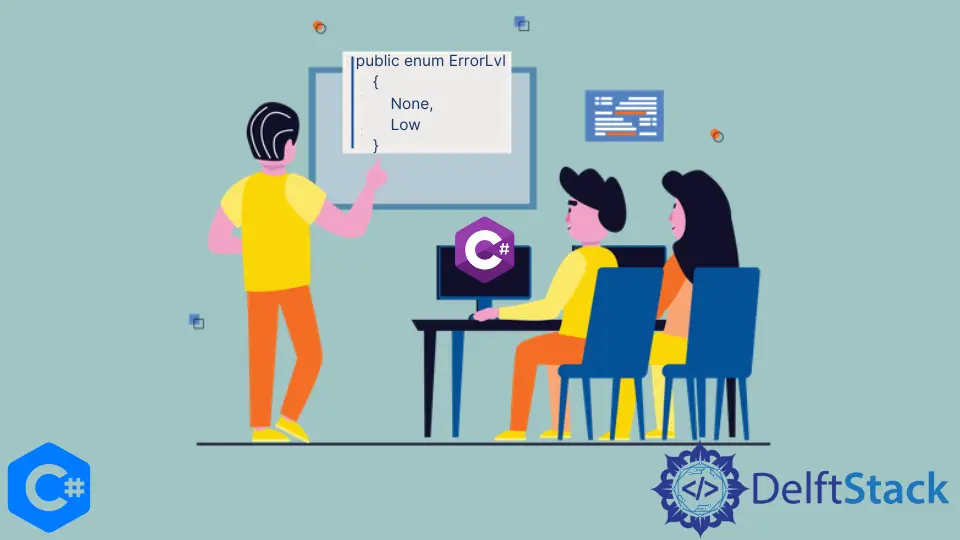
This tutorial will introduce methods to create an enumeration of strings in C#.
Create Enumeration of Strings With Extension Function in C#
An enumeration is a pre-defined set of constant values that a variable can store. The enum
class is used to declare an enumeration in C#. By default, the type of variables inside the enum
class is int. There is no built-in method of declaring an enumeration with string values. If we want to declare an enumeration with string constants, we can use the enum
class and an extension function to achieve this goal. The following code example shows us how to create an enumeration of string values with the enum
class and extension function in C#.
using System;
namespace enum_with_string {
public enum ErrorLevel { None, Low, Medium, High }
public static class ErrorLevelExtensions {
public static string GetString(this ErrorLevel me) {
switch (me) {
case ErrorLevel.None:
return "NO ERRORS";
case ErrorLevel.Low:
return "LOW LEVEL ERROR";
case ErrorLevel.Medium:
return "MEDIUM LEVEL ERROR";
case ErrorLevel.High:
return "HIGH LEVEL ERROR";
default:
return "NO VALUE GIVEN";
}
}
}
class Program {
static void Main(string[] args) {
ErrorLevel e = ErrorLevel.None;
string output = e.GetString();
Console.WriteLine(output);
}
}
}
Output:
NO ERRORS
We declared the enumeration ErrorLevel
with the constant values None, Low, Medium, High
with the enum
keyword. Then we created the extension function GetString()
that uses a switch()
statement to determine the value of the enumeration and return a string according to the value. The result of the GetString()
function is saved in the output
string.
Create Enumeration of Strings With Constant Variables in C#
We can also achieve an enumeration’s functionality by initializing some constant variables inside a class in C#. The const
keyword specifies that a variable is now constant and we cannot change its value. The following code example shows us how we can create an enumeration of strings with constant variables in C#.
using System;
namespace enum_with_string {
public static class Error {
public const string None = "No Errors";
public const string Low = "Low Level Errors";
public const string Medium = "Medium Level Errors";
public const string High = "High Level Errors";
}
class Program {
static void Main(string[] args) {
String output = Error.Low;
Console.WriteLine(output);
}
}
}
Output:
Low Level Errors
We declared a public static class
named Error
and initialized all the public const string
variables inside the class. The reason for keeping the class static
is that we don’t want to create an instance of the Error
class inside the main()
function. We stored the value of Error.Low
inside the string variable output
. This approach is much simpler and easy-to-understand. The same thing can also be done with a structure with the struct
keyword in place of the class
keyword.
Create Enumeration of Strings With the ToString()
Function in C#
This is an improvised method of converting the values’ names inside the enumeration to string in C#. We can use the ToString()
function with the instance of an enumeration to convert the value’s name inside the enumeration to a string. The following code example shows us how to create an enumeration of strings with the ToString()
function in C#.
using System;
namespace enum_with_string {
public enum ErrorLevel { None, Low, Medium, High }
class Program {
static void Main(string[] args) {
ErrorLevel e = ErrorLevel.None;
string output = e.ToString();
Console.WriteLine(output);
}
}
}
Output:
None
We converted the name None
inside the enumeration ErrorLevel
to a string with the ToString()
function in C#. This method is easy-to-use, but there are a few drawbacks. We cannot get the value Low Level Errors
like our previous examples with this approach. We have to follow the naming conventions of C# in this approach. This method is only advisable if we want to create an enumeration of only single-worded strings. For anything else, we should use one of the two ways discussed above.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#