How to Convert Int to Byte in C#
-
Use the
ToByte(String)
Method to ConvertInt
toByte[]
in C# -
Use the
ToByte(UInt16)
Method to ConvertInt
toByte[]
in C# -
Use the
ToByte(String, Int32)
Method to ConvertInt
toByte[]
in C# -
Use
BitConverter.GetBytes
to ConvertInt
toByte[]
in C# -
Use
BinaryWriter
WithMemoryStream
to ConvertInt
toByte[]
in C# -
Use
BitConverter.GetBytes
With Endianness to ConvertInt
toByte[]
in C# - Conclusion
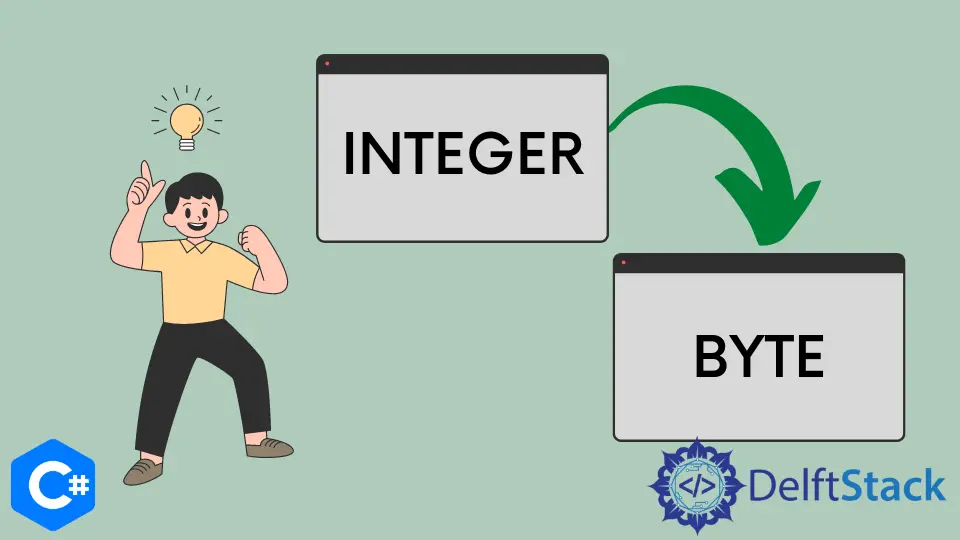
In C#, converting an integer to a byte array is a common operation, often required for tasks like data serialization, network communication, cryptography, and more. This guide explores multiple methods available in C# to perform this conversion, each offering its advantages and suitability for different scenarios.
Use the ToByte(String)
Method to Convert Int
to Byte[]
in C#
While C# doesn’t offer a direct method to convert an int
to a byte[]
using ToByte(string)
, we can leverage string encoding to achieve this conversion by first converting the int
to its string representation and then encoding that string into a byte[]
.
The Convert.ToByte(string)
method in C# is used to convert a string representation of a number to its equivalent 8-bit unsigned integer (byte
) representation. Here is the syntax:
public static byte ToByte(string value)
Parameters:
value
: The string representation of the number to be converted to abyte
. This can represent a number in decimal, hexadecimal, or octal format.
Return Value:
- Returns a
byte
value that represents the parsed string, converted to an 8-bit unsigned integer.
Exceptions:
- The method throws an
OverflowException
if the string representation is outside the range of a validbyte
value (0 to 255). - It throws a
FormatException
if the string is not in a valid format that can be parsed as abyte
.
Example:
First, add these libraries:
using System;
using System.Diagnostics;
We begin by creating a string[]
type variable called Alldatavalues
and assigning some values.
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
Now use a for each
loop to iterate through each element in the Alldatavalues
array, then convert integer to byte using Convert.ToByte()
.
foreach (var value in Alldatavalues) {
byte num = Convert.ToByte(value);
}
A format exception occurs (0 through 9) when a value does not have an optional sign followed by a series of numbers. We utilize the FormatException
handler to overcome this.
catch (FormatException) {
Console.WriteLine("Unsuported Format: '{0}'", value == null ? "<null>" : value);
}
An overflow error arises when a value reflects lesser than MinValue
or greater than MaxValue
.
catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
Full code:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
foreach (var value in Alldatavalues) {
try {
byte num = Convert.ToByte(value);
Console.WriteLine("'{0}' > {1}", value == null ? "<null>" : value, num);
} catch (FormatException) {
Console.WriteLine("Unsupported Format: '{0}'", value == null ? "<null>" : value);
} catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
}
}
}
Output:
'<null>' > 0
Unsupported Format: ''
Data Overflow Exception: '3227'
Unsupported Format: 'It5'
'99' > 99
Unsupported Format: '9*9'
Unsupported Format: '25.6'
Unsupported Format: '$50'
Data Overflow Exception: '-11'
Unsupported Format: 'St8'
Use the ToByte(UInt16)
Method to Convert Int
to Byte[]
in C#
In C#, the Convert.ToByte(UInt16)
method is used to convert a 16-bit unsigned integer (UInt16
) value to an 8-bit unsigned integer (byte
). Here’s the syntax:
public static byte ToByte(UInt16 value)
Parameters:
value
: The 16-bit unsigned integer (UInt16
) value that you want to convert to an 8-bit unsigned integer (byte
).
Return Value:
- Returns a
byte
value that represents the converted 8-bit unsigned integer from the providedUInt16
value.
Exceptions:
- This method will throw an
OverflowException
if thevalue
is greater thanbyte.MaxValue
(255).
In the following example, an array of unsigned 16-bit integers is converted to byte values.
Libraries to be added are:
using System;
using System.Diagnostics;
First, initialize a ushort[]
type variable named data
; after that, we’ll assign some value to it like UInt16.MinValue
as a minimum value, UInt16.MaxValue
as the maximum value and other values that we want to convert like we have inserted 90
and 880
.
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
Then we’ll create a byte
type variable named result
.
byte result;
After that, apply a foreach
loop, which will convert data into Byte
type; other than that, if a number exceeds the range of Byte
, it will show an exception.
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.", numberdata.GetType().Name,
numberdata);
}
}
Full Code:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
byte result;
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.",
numberdata.GetType().Name, numberdata);
}
}
}
}
Output:
Successfully converted the UInt16 value 0 to the Byte value 0.
Successfully converted the UInt16 value 90 to the Byte value 90.
The UInt16 value 880 is outside the range of Byte.
The UInt16 value 65535 is outside the range of Byte.
Use the ToByte(String, Int32)
Method to Convert Int
to Byte[]
in C#
The Convert.ToByte(string, int)
method in C# is used to convert a specified string representation of a number in a given base to its equivalent 8-bit unsigned integer (byte
). Here’s the syntax:
Syntax:
public static byte ToByte(string value, int fromBase)
Parameters:
value
: The string representation of the number to be converted to abyte
.fromBase
: An integer specifying the base of the number in thevalue
parameter. It must be between 2 and 36, inclusive.
Return Value:
- Returns a
byte
value that represents the parsed string, converted to an 8-bit unsigned integer.
Exceptions:
- The method throws a
FormatException
ifvalue
is not in the correct format or cannot be parsed as a number in the specified base. - It throws an
OverflowException
if the parsed number exceeds the range of a validbyte
value (0 to 255).
Example:
The following libraries will be added:
using System;
using System.Diagnostics;
First, we’ll construct an int[]
type variable called Allbasedata
and assign the bases 8
and 10
.
int[] Allbasedata = { 8, 10 };
Now we create a string[]
type variable called values
and assign values to it, and declare a byte
type variable called number
.
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
Then we’ll apply a foreach
loop in which it converts the number to byte
format, and it will also handle exceptions like:
FormatException
: This error occurs when the value contains a character that is not a valid digit in the base supplied by from base.OverflowException
: This error occurs when a negative sign is prefixed to a value representing a base 10 unsigned number.ArgumentException
: This error occurs when the value is empty.
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
Full code:
using System;
using System.Diagnostics;
public class ToByteStringInt32 {
public static void Main() {
int[] Allbasedata = { 8, 10 };
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
foreach (int numberBase in Allbasedata) {
Console.WriteLine("Base Number {0}:", numberBase);
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
}
}
}
Output:
Base Number 8:
'80000000' is an Invalid format for a base 8 byte value.
'1201' is Outside the Range of Byte type.
'-6' is invalid in base 8.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 8 byte value.
'99' is an Invalid format for a base 8 byte value.
Converted '12' to 10.
Converted '70' to 56.
Converted '255' to 173.
Base Number 10:
'80000000' is Outside the Range of Byte type.
'1201' is Outside the Range of Byte type.
'-6' is Outside the Range of Byte type.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 10 byte value.
Converted '99' to 99.
Converted '12' to 12.
Converted '70' to 70.
Converted '255' to 255.
Use BitConverter.GetBytes
to Convert Int
to Byte[]
in C#
The GetBytes
method within BitConverter
is used to convert an int
to a byte array. This method takes an int
value as input and returns a byte array representing the binary representation of that integer.
Syntax:
public static byte[] GetBytes(int value)
Parameters:
value
: The integer value to be converted to a byte array.
Return Value:
- Returns a byte array representing the binary data of the input integer.
The BitConverter.GetBytes
method is a straightforward way to convert an integer to a byte array by leveraging the BitConverter
class.
Example Code:
using System;
class Program {
static void Main() {
int number = 123456789;
byte[] byteArray = BitConverter.GetBytes(number);
Console.WriteLine("Byte Array:");
foreach (byte b in byteArray) {
Console.Write(b + " ");
}
}
}
Output:
Byte Array:
21 205 91 7
The code converts the integer 123456789
into a byte array and displays the resulting byte sequence in the console. Using BitConverter.GetBytes()
, the integer is transformed into a byte array, representing its binary form.
The code then prints the byte array values to the console as a sequence of four bytes: 21 205 91 7
. The byte order may vary depending on the system’s endianness.
Use BinaryWriter
With MemoryStream
to Convert Int
to Byte[]
in C#
Using BinaryWriter
in combination with a MemoryStream
provides a flexible approach for converting integers to byte arrays.
BinaryWriter
: A class in C# that provides methods to write primitive data types as binary values in a specific encoding to a stream.MemoryStream
: Represents a stream whose backing store is memory. It allows for reading and writing bytes that are stored in memory.
Example Code:
using System;
using System.IO;
class Program {
static void Main() {
int number = 987654321;
using (MemoryStream stream = new MemoryStream()) {
using (BinaryWriter writer = new BinaryWriter(stream)) {
writer.Write(number);
}
byte[] byteArray = stream.ToArray();
Console.WriteLine("Byte Array:");
foreach (byte b in byteArray) {
Console.Write(b + " ");
}
}
}
}
Output:
Byte Array:
177 104 222 58
The code uses BinaryWriter
with MemoryStream
to convert an integer (987654321
) into a byte array. Within a nested using
statement, the code writes the integer to the MemoryStream
using BinaryWriter
, retrieves the resulting byte array, and displays it in the console as a sequence of bytes separated by spaces.
This method showcases a streamlined approach for converting integers to byte arrays in C#, providing control over binary data manipulation and efficient byte array retrieval.
Use BitConverter.GetBytes
With Endianness to Convert Int
to Byte[]
in C#
For controlling the endianness (byte order) of the resulting byte array, BitConverter
can be combined with manual byte array reversal.
BitConverter
: A class in C# that provides static methods to convert base data types to an array of bytes.Endianness
: The arrangement of bytes that form larger data types such as integers. It defines whether the most significant byte (MSB) or least significant byte (LSB) comes first in memory.
Example Code:
using System;
class Program {
static void Main() {
int number = 567890123;
byte[] byteArray = BitConverter.GetBytes(number);
if (BitConverter.IsLittleEndian) {
Array.Reverse(byteArray);
}
Console.WriteLine("Byte Array:");
foreach (byte b in byteArray) {
Console.Write(b + " ");
}
}
}
Output:
Byte Array:
33 217 80 203
This code snippet uses BitConverter.GetBytes
to convert the integer 567890123
into a byte array. It checks the system’s endianness using BitConverter.IsLittleEndian
and reverses the byte array if the system is little-endian.
The resulting byte array is then displayed in the console as a sequence of bytes separated by spaces. This approach ensures consistent byte representation across different system architectures when converting integers to byte arrays in C#.
Conclusion
In conclusion, the article explores various methods to convert integers to byte arrays in C#, each suited for different scenarios. It covers methodologies such as using the ToByte(String)
method, ToByte(UInt16)
method, and ToByte(String, Int32)
method, demonstrating their usage along with error handling for unsupported formats or overflow exceptions.
Additionally, it explains the utilization of BitConverter.GetBytes
to directly convert an int
to a byte[]
, showcasing its simplicity and efficiency. The code samples illustrate how to obtain byte arrays representing integers, considering system endianness and controlling byte order for consistent representation across different architectures.
By understanding these diverse approaches, developers can efficiently handle integer-to-byte-array conversions in C#, addressing various encoding needs and ensuring compatibility and reliability across different system architectures and data representations. Each method offers its advantages and capabilities, empowering developers to choose the most suitable approach based on their specific requirements.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedInRelated Article - Csharp Int
- How to Convert a Char to an Int in C#
- How to Convert Int to Float in C#
- How to Convert Char to Int in C#