在 C# 中將 Int 轉換為位元組
-
在
C#
中使用ToByte(String)
方法將Int
轉換為Byte[]
-
在
C#
中使用ToByte(UInt16)
方法將Int
轉換為Byte[]
-
在
C#
中使用ToByte(String, Int32)
方法將Int
轉換為Byte[]
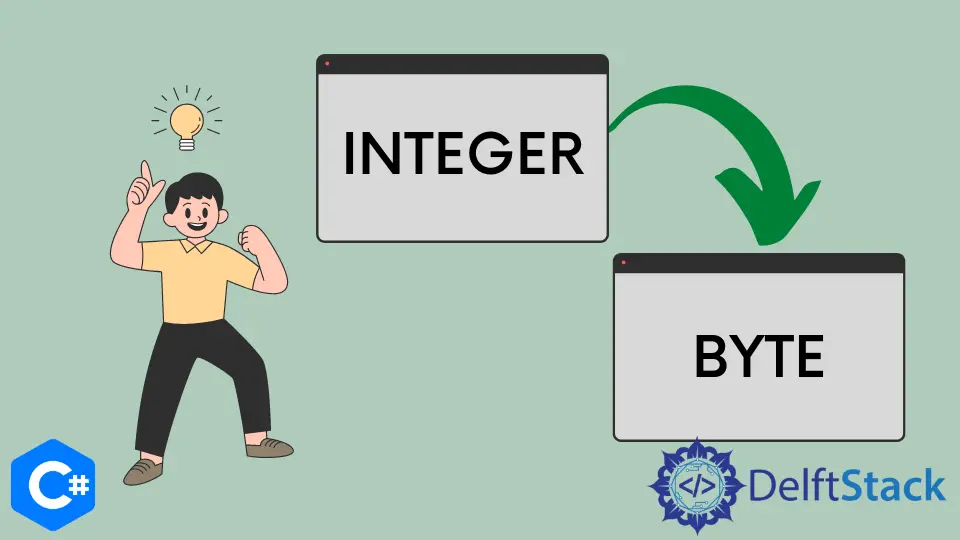
我們將研究在 C# 中將 Int
轉換為 Byte[]
的幾種不同技術。
在 C#
中使用 ToByte(String)
方法將 Int
轉換為 Byte[]
這種方法通過使用 ToByte(String)
方法將提供的數字字串表示形式轉換為等效的 8 位無符號整數來工作。它作為一個字串引數,包含要轉換的數字。
下面的示例建立一個字串陣列並將每個字串轉換為一個位元組。
首先,新增這些庫。
using System;
using System.Diagnostics;
我們首先建立一個名為 Alldatavalues
的 string[]
型別變數並分配一些值。
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
現在使用 Convert.ToByte()
使用 for each
迴圈將整數轉換為位元組。
byte num = Convert.ToByte(value);
當值沒有可選符號後跟一系列數字時,會發生格式異常(0 到 9)。我們利用 FormatException
處理程式來克服這個問題。
catch (FormatException) {
Console.WriteLine("Unsuported Format: '{0}'", value == null ? "<null>" : value);
}
當值反映小於 MinValue
或大於 MaxValue
時,會出現溢位錯誤。
catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
原始碼:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
foreach (var value in Alldatavalues) {
try {
byte num = Convert.ToByte(value);
Console.WriteLine("'{0}' --> {1}", value == null ? "<null>" : value, num);
} catch (FormatException) {
Console.WriteLine("Unsupported Format: '{0}'", value == null ? "<null>" : value);
} catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
}
}
}
輸出:
'<null>' --> 0
Unsupported Format: ''
Data Overflow Exception: '3227'
Unsupported Format: 'It5'
'99' --> 99
Unsupported Format: '9*9'
Unsupported Format: '25.6'
Unsupported Format: '$50'
Data Overflow Exception: '-11'
Unsupported Format: 'St8'
在 C#
中使用 ToByte(UInt16)
方法將 Int
轉換為 Byte[]
ToByte(UInt16)
方法將 16 位無符號整數的值轉換為等效的 8 位無符號整數。要進行轉換,它需要一個 16 位無符號整數作為引數。
在以下示例中,無符號 16 位整數陣列被轉換為位元組值。
要新增的庫有:
using System;
using System.Diagnostics;
首先,初始化一個名為 data
的 ushort[]
型別變數;之後,我們將為其分配一些值,例如 UInt16.MinValue
作為最小值,UInt16.MaxValue
作為最大值以及我們想要轉換的其他值,就像我們插入 90
和 880
.
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
然後我們將建立一個名為 result
的 byte
型別變數。
byte result;
之後,應用一個 for each
迴圈,它將轉換為 Byte
型別的資料;除此之外,如果一個數字超出 Byte
的範圍,它將顯示異常。
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.", numberdata.GetType().Name,
numberdata);
}
}
原始碼:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
byte result;
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.",
numberdata.GetType().Name, numberdata);
}
}
}
}
輸出:
Successfully converted the UInt16 value 0 to the Byte value 0.
Successfully converted the UInt16 value 90 to the Byte value 90.
The UInt16 value 880 is outside the range of Byte.
The UInt16 value 65535 is outside the range of Byte.
在 C#
中使用 ToByte(String, Int32)
方法將 Int
轉換為 Byte[]
此方法將數字的字串表示形式轉換為給定基數的等效 8 位無符號整數。它需要一個包含要轉換的數字的 string
引數值。
將新增以下庫。
using System;
using System.Diagnostics;
首先,我們將構造一個名為 Allbasedata
的 int[]
型別變數並分配基數 8
和 10
。
int[] Allbasedata = { 8, 10 };
現在我們建立一個名為 values
的 string[]
型別變數併為其賦值,並宣告一個名為 number
的 byte
型別變數。
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
然後我們將應用一個 foreach
迴圈,它將數字轉換為 byte
格式,它還將處理以下異常:
FormatException
:當值包含的字元不是來自 base 提供的 base 中的有效數字時,會發生此錯誤。OverflowException
:當負號字首到表示以 10 為基數的無符號數的值時,會發生此錯誤。ArgumentException
:當值為空時發生此錯誤。
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
原始碼:
using System;
using System.Diagnostics;
public class ToByteStringInt32 {
public static void Main() {
int[] Allbasedata = { 8, 10 };
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
foreach (int numberBase in Allbasedata) {
Console.WriteLine("Base Number {0}:", numberBase);
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
}
}
}
輸出:
Base Number 8:
'80000000' is an Invalid format for a base 8 byte value.
'1201' is Outside the Range of Byte type.
'-6' is invalid in base 8.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 8 byte value.
'99' is an Invalid format for a base 8 byte value.
Converted '12' to 10.
Converted '70' to 56.
Converted '255' to 173.
Base Number 10:
'80000000' is Outside the Range of Byte type.
'1201' is Outside the Range of Byte type.
'-6' is Outside the Range of Byte type.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 10 byte value.
Converted '99' to 99.
Converted '12' to 12.
Converted '70' to 70.
Converted '255' to 255.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn