Konvertieren Int in Byte in C#
-
Verwenden Sie die
ToByte(String)
-Methode, umInt
inByte[]
inC#
zu konvertieren -
Verwenden Sie die Methode
ToByte(UInt16)
, umInt
inByte[]
inC#
zu konvertieren -
Verwenden Sie die Methode
ToByte(String, Int32)
umInt
inByte[]
inC#
zu konvertieren
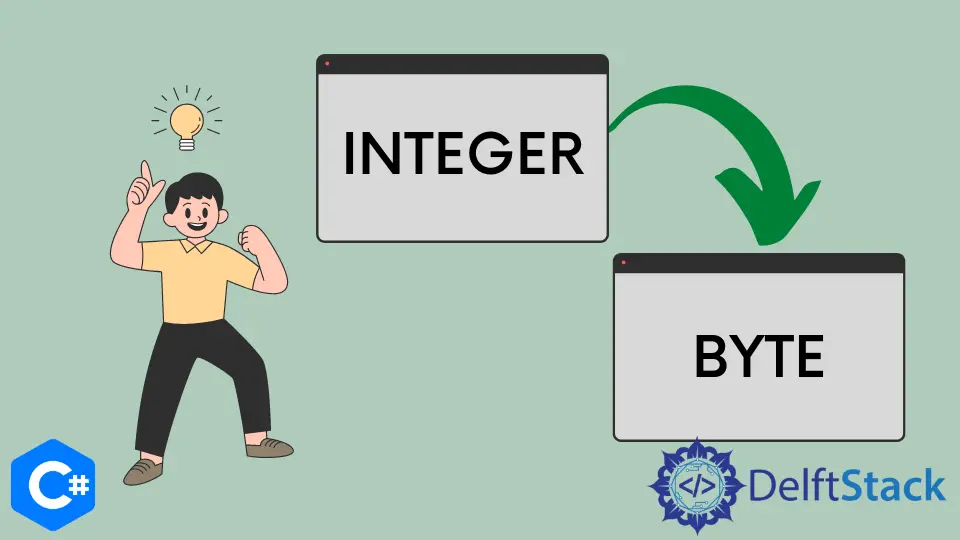
Wir werden uns ein paar verschiedene Techniken ansehen, um Int
in Byte[]
in C# umzuwandeln.
Verwenden Sie die ToByte(String)
-Methode, um Int
in Byte[]
in C#
zu konvertieren
Dieser Ansatz funktioniert, indem die bereitgestellte Zeichenfolgendarstellung einer Zahl mithilfe der Methode ToByte(String)
in eine entsprechende 8-Bit-Ganzzahl ohne Vorzeichen umgewandelt wird. Es wird als String-Argument verwendet, das die zu konvertierende Zahl enthält.
Das folgende Beispiel erstellt ein String-Array und konvertiert jeden String in ein Byte.
Fügen Sie zuerst diese Bibliotheken hinzu.
using System;
using System.Diagnostics;
Wir beginnen damit, eine Variable vom Typ string[]
mit dem Namen Alldatavalues
zu erstellen und ihr einige Werte zuzuweisen.
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
Verwenden Sie nun eine for each
-Schleife, um mit Convert.ToByte()
Integer in Byte umzuwandeln.
byte num = Convert.ToByte(value);
Eine Formatausnahme tritt auf (0 bis 9), wenn ein Wert kein optionales Zeichen gefolgt von einer Reihe von Zahlen hat. Wir verwenden den Handler FormatException
, um dies zu überwinden.
catch (FormatException) {
Console.WriteLine("Unsuported Format: '{0}'", value == null ? "<null>" : value);
}
Ein Überlauffehler tritt auf, wenn ein Wert kleiner als MinValue
oder grösser als MaxValue
ist.
catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
Quellcode:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
string[] Alldatavalues = { null, "", "3227", "It5", "99", "9*9", "25.6", "$50", "-11", "St8" };
foreach (var value in Alldatavalues) {
try {
byte num = Convert.ToByte(value);
Console.WriteLine("'{0}' --> {1}", value == null ? "<null>" : value, num);
} catch (FormatException) {
Console.WriteLine("Unsupported Format: '{0}'", value == null ? "<null>" : value);
} catch (OverflowException) {
Console.WriteLine("Data Overflow Exception: '{0}'", value);
}
}
}
}
Ausgabe:
'<null>' --> 0
Unsupported Format: ''
Data Overflow Exception: '3227'
Unsupported Format: 'It5'
'99' --> 99
Unsupported Format: '9*9'
Unsupported Format: '25.6'
Unsupported Format: '$50'
Data Overflow Exception: '-11'
Unsupported Format: 'St8'
Verwenden Sie die Methode ToByte(UInt16)
, um Int
in Byte[]
in C#
zu konvertieren
Die Methode ToByte(UInt16)
konvertiert den Wert einer 16-Bit-Ganzzahl ohne Vorzeichen in eine 8-Bit-Ganzzahl ohne Vorzeichen. Zum Konvertieren ist eine 16-Bit-Ganzzahl ohne Vorzeichen als Argument erforderlich.
Im folgenden Beispiel wird ein Array aus vorzeichenlosen 16-Bit-Ganzzahlen in Byte-Werte konvertiert.
Bibliotheken, die hinzugefügt werden sollen, sind:
using System;
using System.Diagnostics;
Initialisieren Sie zuerst eine Variable vom Typ ushort[]
mit dem Namen data
; Danach weisen wir ihm einen Wert zu, wie UInt16.MinValue
als Mindestwert, UInt16.MaxValue
als Höchstwert und andere Werte, die wir konvertieren möchten, als hätten wir 90
und 880
eingefügt. .
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
Dann erstellen wir eine Variable vom Typ byte
mit dem Namen result
.
byte result;
Wenden Sie danach eine for each
-Schleife an, die Daten in den Typ Byte
konvertiert; Ansonsten wird eine Ausnahme angezeigt, wenn eine Zahl den Bereich von Byte
überschreitet.
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.", numberdata.GetType().Name,
numberdata);
}
}
Quellcode:
using System;
using System.Diagnostics;
public class ToByteString {
public static void Main() {
ushort[] data = { UInt16.MinValue, 90, 880, UInt16.MaxValue };
byte result;
foreach (ushort numberdata in data) {
try {
result = Convert.ToByte(numberdata);
Console.WriteLine("Successfully converted the {0} value {1} to the {2} value {3}.",
numberdata.GetType().Name, numberdata, result.GetType().Name, result);
} catch (OverflowException) {
Console.WriteLine("The {0} value {1} is outside the range of Byte.",
numberdata.GetType().Name, numberdata);
}
}
}
}
Ausgabe:
Successfully converted the UInt16 value 0 to the Byte value 0.
Successfully converted the UInt16 value 90 to the Byte value 90.
The UInt16 value 880 is outside the range of Byte.
The UInt16 value 65535 is outside the range of Byte.
Verwenden Sie die Methode ToByte(String, Int32)
um Int
in Byte[]
in C#
zu konvertieren
Diese Methode konvertiert die Zeichenfolgendarstellung einer Zahl in eine äquivalente 8-Bit-Ganzzahl ohne Vorzeichen in einer bestimmten Basis. Es nimmt einen string
-Parameterwert an, der die zu konvertierende Zahl enthält.
Die folgenden Bibliotheken werden hinzugefügt.
using System;
using System.Diagnostics;
Zuerst konstruieren wir eine Variable vom Typ int[]
namens Allbasedata
und weisen ihr die Basen 8
und 10
zu.
int[] Allbasedata = { 8, 10 };
Jetzt erstellen wir eine Variable vom Typ string[]
namens values
und weisen ihr Werte zu und deklarieren eine Variable vom Typ byte
namens number
.
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
Dann wenden wir eine foreach
-Schleife an, in der die Zahl in das byte
-Format konvertiert wird, und es behandelt auch Ausnahmen wie:
FormatException
: Dieser Fehler tritt auf, wenn der Wert ein Zeichen enthält, das keine gültige Ziffer in der von base gelieferten Basis ist.OverflowException
: Dieser Fehler tritt auf, wenn einem Wert, der eine vorzeichenlose Zahl zur Basis 10 darstellt, ein negatives Vorzeichen vorangestellt wird.ArgumentException
: Dieser Fehler tritt auf, wenn der Wert leer ist.
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
Quellcode:
using System;
using System.Diagnostics;
public class ToByteStringInt32 {
public static void Main() {
int[] Allbasedata = { 8, 10 };
string[] values = { "80000000", "1201", "-6", "6", "07", "MZ", "99", "12", "70", "255" };
byte number;
foreach (int numberBase in Allbasedata) {
Console.WriteLine("Base Number {0}:", numberBase);
foreach (string value in values) {
try {
number = Convert.ToByte(value, numberBase);
Console.WriteLine(" Converted '{0}' to {1}.", value, number);
} catch (FormatException) {
Console.WriteLine(" '{0}' is an Invalid format for a base {1} byte value.", value,
numberBase);
} catch (OverflowException) {
Console.WriteLine(" '{0}' is Outside the Range of Byte type.", value);
} catch (ArgumentException) {
Console.WriteLine(" '{0}' is invalid in base {1}.", value, numberBase);
}
}
}
}
}
Ausgabe:
Base Number 8:
'80000000' is an Invalid format for a base 8 byte value.
'1201' is Outside the Range of Byte type.
'-6' is invalid in base 8.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 8 byte value.
'99' is an Invalid format for a base 8 byte value.
Converted '12' to 10.
Converted '70' to 56.
Converted '255' to 173.
Base Number 10:
'80000000' is Outside the Range of Byte type.
'1201' is Outside the Range of Byte type.
'-6' is Outside the Range of Byte type.
Converted '6' to 6.
Converted '07' to 7.
'MZ' is an Invalid format for a base 10 byte value.
Converted '99' to 99.
Converted '12' to 12.
Converted '70' to 70.
Converted '255' to 255.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedInVerwandter Artikel - Csharp Int
- C# Konvertieren eines Zeichens in ein int
- Konvertieren Char in Int in C#
- Konvertieren Int in Float in C#