How to Convert a Char to an Int in C#
-
C# Program to Convert a
Char
to anInt
UsingGetNumericValue()
Method -
C# Program to Convert a
Char
to anInt
UsingDifference with 0
Method -
C# Program to Convert a
Char
to anInt
UsingInt32.Parse()
Method -
C# Program to Convert a
Char
to anInt
UsingGetDecimalDigitValue()
Method
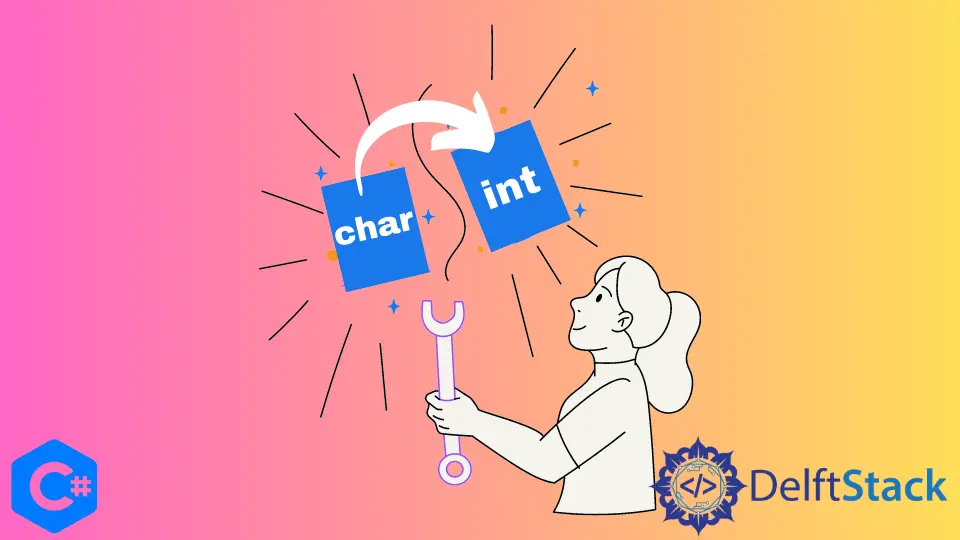
In this article, we are going to discuss different methods to convert a character into an integer.
You can learn how to convert an int to char in this article.
C# Program to Convert a Char
to an Int
Using GetNumericValue()
Method
GetNumericValue()
is a built-in method to convert a character
to an integer if the character
is a numeric value. If the character
is not a numeric value, it returns a negative value.
The correct syntax to use this method is as follows:
(int)Char.GetNumericValue(CharacterName);
This method returns a value of data type double
. To convert it to an int
, we could use typecasting.
Example Code:
using System;
namespace Example {
class Conversion {
static void Main(string[] args) {
char Character = '9';
Console.WriteLine("The character is: " + Character);
int integer = (int)Char.GetNumericValue(Character);
Console.WriteLine("The integer is: {0}", integer);
}
}
}
Output:
The character is: 9
The integer is: 9
C# Program to Convert a Char
to an Int
Using Difference with 0
Method
We all know that we have ASCII characters ranging from 0-127. In order to convert a numeric character to an integer, we will simply subtract a zero character
('0'
) from it. The resultant value will be an integer value. If the character is non-numeric, then subtracting a zero from it will result in a random integer value.
The correct syntax to use this method is as follows:
IntegerName = CharacterName - '0';
Example Code:
using System;
namespace Example {
class Conversion {
static void Main(string[] args) {
char Character = '9';
Console.WriteLine("The character is: " + Character);
int integer = Character - '0';
Console.WriteLine("The integer is: {0}", integer);
}
}
}
Output:
The character is: 9
The integer is: 9
C# Program to Convert a Char
to an Int
Using Int32.Parse()
Method
The method Int32.Parse()
converts a string into an integer. We can also use it to convert a character
into an integer.
The correct syntax to use this method is as follows:
int.Parse(CharacterName.ToString());
Here we have passed Character.ToString()
as a parameter to int.Parse()
method. The method Character.ToString()
converts the character
to a string. This string is then converted into an integer.
Example Code:
using System;
namespace Example {
class Conversion {
static void Main(string[] args) {
char Character = '9';
Console.WriteLine("The character is: " + Character);
int integer = int.Parse(Character.ToString());
Console.WriteLine("The integer is: {0}", integer);
}
}
}
Output:
The character is: 9
The integer is: 9
C# Program to Convert a Char
to an Int
Using GetDecimalDigitValue()
Method
GetDecimalDigitValue()
method accepts a Unicode
character as a parameter and returns the decimal digit value of the Unicode
character. This method belongs to System.Globalization
namespace.
The correct syntax to use this method is as follows:
CharUnicodeInfo.GetDecimalDigitValue(CharacterName);
Example Code:
using System;
using System.Globalization;
namespace Example {
class Conversion {
static void Main(string[] args) {
char Character = '9';
Console.WriteLine("The character is: " + Character);
int integer = CharUnicodeInfo.GetDecimalDigitValue(Character);
Console.WriteLine("The integer is: {0}", integer);
}
}
}
Output:
The character is: 9
The integer is: 9
Related Article - Csharp Char
- How to Convert Char to Int in C#
- How to Count Occurrences of a Character Inside a String in C#
- How to Get the First Character of a String in C#
- How to Get ASCII Value of Character in C#
- How to Remove a Character From a String in C#