C# Converte un carattere in un int
-
Programma C# per convertire un
Char
in unInt
utilizzando il metodoGetNumericValue()
-
Programma C# per convertire un
Char
in unInt
usando il metodoDifference with 0
-
Programma C# per convertire un
Char
in unInt
usando il metodoInt32.Parse()
-
Programma C# per convertire un
Char
in unInt
utilizzando il metodoGetDecimalDigitValue()
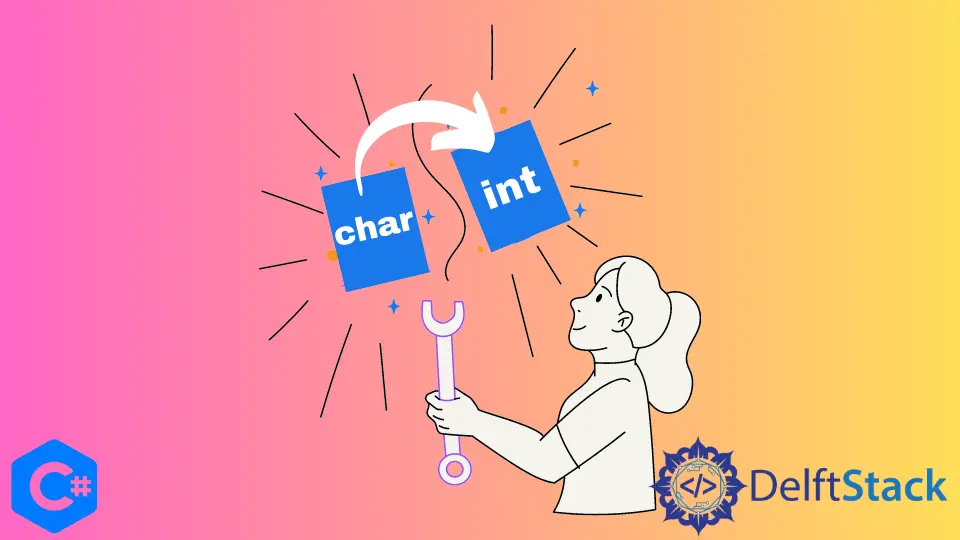
In questo articolo, discuteremo diversi metodi per convertire un carattere in un numero intero.
Programma C# per convertire un Char
in un Int
utilizzando il metodo GetNumericValue()
GetNumericValue()
è un metodo integrato per convertire un carattere
in un intero se il carattere
è un valore numerico. Se il “carattere” non è un valore numerico, restituisce un valore negativo.
La sintassi corretta per utilizzare questo metodo è la seguente:
(int)Char.GetNumericValue(CharacterName);
Questo metodo restituisce un valore del tipo di dati double
. Per convertirlo in un int
, potremmo usare typecasting.
Codice di esempio:
using System;
namespace Example {
class Conversion {
static void Main(string[] args) {
char Character = '9';
Console.WriteLine("The character is: " + Character);
int integer = (int)Char.GetNumericValue(Character);
Console.WriteLine("The integer is: {0}", integer);
}
}
}
Produzione:
The character is : 9 The integer is : 9
Programma C# per convertire un Char
in un Int
usando il metodo Difference with 0
Sappiamo tutti che abbiamo caratteri ASCII che vanno da 0 a 127. Per convertire un carattere numerico in un intero, sottrarremo semplicemente uno zero carattere
('0'
) da esso. Il valore risultante sarà un valore intero. Se il carattere non è numerico, la sottrazione di uno zero risulterà in un valore intero casuale.
La sintassi corretta per utilizzare questo metodo è la seguente:
IntegerName = CharacterName - '0';
Codice di esempio:
using System;
namespace Example {
class Conversion {
static void Main(string[] args) {
char Character = '9';
Console.WriteLine("The character is: " + Character);
int integer = Character - '0';
Console.WriteLine("The integer is: {0}", integer);
}
}
}
Produzione:
The character is : 9 The integer is : 9
Programma C# per convertire un Char
in un Int
usando il metodo Int32.Parse()
Il metodo Int32.Parse()
converte una stringa in un numero intero. Possiamo anche usarlo per convertire un carattere
in un numero intero.
La sintassi corretta per utilizzare questo metodo è la seguente:
int.Parse(CharacterName.ToString());
Qui abbiamo passato Character.ToString()
come parametro al metodo int.Parse()
. Il metodo Character.ToString()
converte il carattere
in una stringa. Questa stringa viene quindi convertita in un numero intero.
Codice di esempio:
using System;
namespace Example {
class Conversion {
static void Main(string[] args) {
char Character = '9';
Console.WriteLine("The character is: " + Character);
int integer = int.Parse(Character.ToString());
Console.WriteLine("The integer is: {0}", integer);
}
}
}
Produzione:
The character is : 9 The integer is : 9
Programma C# per convertire un Char
in un Int
utilizzando il metodo GetDecimalDigitValue()
Il metodo GetDecimalDigitValue()
accetta un carattere Unicode
come parametro e restituisce il valore della cifra decimale del carattere Unicode
. Questo metodo appartiene allo spazio dei nomi System.Globalization
.
La sintassi corretta per utilizzare questo metodo è la seguente:
CharUnicodeInfo.GetDecimalDigitValue(CharacterName);
Codice di esempio:
using System;
using System.Globalization;
namespace Example {
class Conversion {
static void Main(string[] args) {
char Character = '9';
Console.WriteLine("The character is: " + Character);
int integer = CharUnicodeInfo.GetDecimalDigitValue(Character);
Console.WriteLine("The integer is: {0}", integer);
}
}
}
Produzione:
The character is : 9 The integer is : 9