C# Convert Int to Char
-
Cast
int
tochar
in C# Using Explicit Type Cast -
Cast
int
tochar
in C# Using theConvert.ToChar()
Method -
Cast
int
tochar
in C# Using theChar.ConvertFromUtf32()
Method -
Cast
int
tochar
in C# Using theToString()
Method - Conclusion
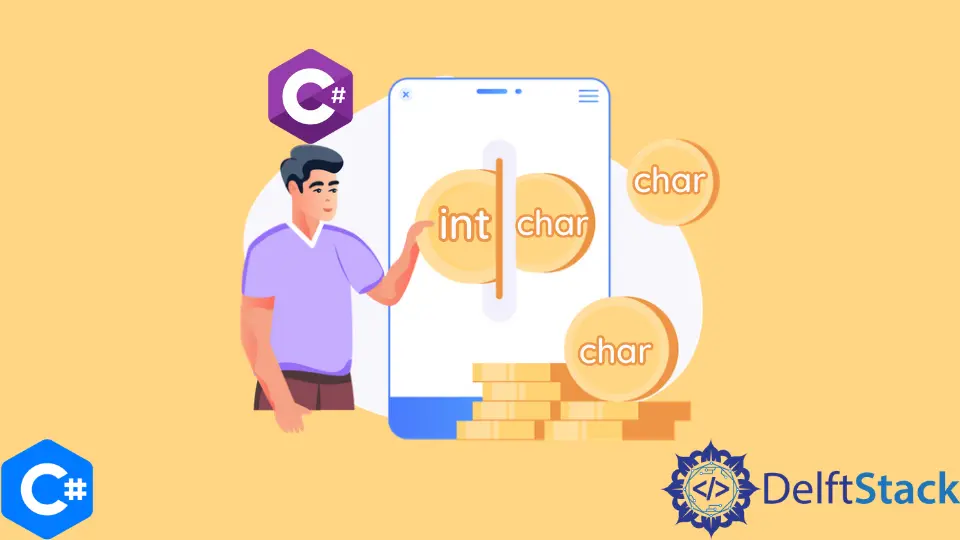
Casting an int
to a char
is a common operation in C# when working with character representations of numerical values.
In this article, we’ll explore various methods to accomplish this conversion. From the classic explicit type cast to specialized methods like Convert.ToChar()
and Char.ConvertFromUtf32()
, each approach has its merits.
Understanding these techniques is important for efficiently handling numeric-to-character conversions in C# applications.
Cast int
to char
in C# Using Explicit Type Cast
In C#, casting an int
to a char
is a straightforward process that involves using explicit type casting.
This method allows you to explicitly specify the type of conversion you want to perform. In this article, we’ll explore the syntax and functionality of explicit type casting for converting an int
to a char
in C#.
The syntax for explicit type casting in C# involves placing the target type in parentheses before the value you want to cast. Here’s the basic syntax for casting an int
to a char
:
(char)intValue;
In this syntax, (char)
indicates the explicit type cast, and intValue
is the int
variable you want to convert to a char
.
Let’s dive into a complete working example to illustrate how explicit type casting is used to convert an int
to a char
.
using System;
public class Program {
public static void Main() {
int intValue = 102;
char charValue = (char)intValue;
Console.WriteLine($"(char){intValue} is {charValue}");
}
}
In the provided example, we start by defining an int
variable named intValue
with a value of 102. The next line demonstrates the explicit type cast, where (char)
is used to convert the intValue
to a char
, assigned to the variable charValue
.
The output statement uses Console.WriteLine
to display the result. The string includes the original integer value and the resulting character value.
Output:
(char)102 is f
In this example, we successfully cast the int
value 102 to the corresponding char
, which is f
, using explicit type casting in C#.
Cast int
to char
in C# Using the Convert.ToChar()
Method
Another method for casting an int
to a char
involves using the Convert.ToChar()
method. This method is specifically designed for explicit type conversion and provides a convenient way to achieve the desired result.
The syntax for using the Convert.ToChar()
method is quite straightforward. You simply provide the int
value as an argument to the method, and it returns the corresponding char
value:
Convert.ToChar(intValue);
Here, intValue
represents the int
variable you want to convert to a char
.
Let’s delve into a complete working example to illustrate how the Convert.ToChar()
method is used to convert an int
to a char
in C#.
using System;
public class Program {
public static void Main() {
int intValue = 65;
char charValue = Convert.ToChar(intValue);
Console.WriteLine($"Convert.ToChar({intValue}) is {charValue}");
}
}
In this example, we initiate the process by declaring an int
variable named intValue
with a value of 65. The subsequent line demonstrates the use of the Convert.ToChar()
method, where Convert.ToChar(intValue)
converts the intValue
to a char
, and the result is assigned to the variable charValue
.
Output:
Convert.ToChar(65) is A
In this example, we successfully employed the Convert.ToChar()
method to cast the int
value 65 to the corresponding char
, which is A
, in C#.
Cast int
to char
in C# Using the Char.ConvertFromUtf32()
Method
When dealing with Unicode code points, the Char.ConvertFromUtf32()
method provides a specialized way to convert an int
to a char
. This method is particularly useful when the int
value represents a Unicode code point.
The syntax for using the Char.ConvertFromUtf32()
method involves providing the int
value (Unicode code point) as an argument to the method. It returns a string containing the corresponding char
value:
Char.ConvertFromUtf32(unicodeCodePoint);
Here, unicodeCodePoint
represents the int
value (Unicode code point) you want to convert to a char
.
Let’s explore a complete working example to illustrate how the Char.ConvertFromUtf32()
method is used to convert an int
to a char
in C#.
using System;
public class Program {
public static void Main() {
int unicodeCodePoint = 65;
string charString = Char.ConvertFromUtf32(unicodeCodePoint);
char charValue = charString[0];
Console.WriteLine($"Char.ConvertFromUtf32({unicodeCodePoint}) is {charValue}");
}
}
In this example, we start by declaring an int
variable named unicodeCodePoint
with a value of 65, representing the Unicode code point for the character A
. The subsequent line utilizes the Char.ConvertFromUtf32()
method, where Char.ConvertFromUtf32(unicodeCodePoint)
converts the unicodeCodePoint
to a string containing the corresponding char
value.
Since the result is a string, we extract the first character using charString[0]
and store it in the charValue
variable. We use Console.WriteLine
to output the result.
Output:
Char.ConvertFromUtf32(65) is A
In this example, we successfully utilized the Char.ConvertFromUtf32()
method to cast the int
value 65 (Unicode code point for A
) to the corresponding char
in C#.
Cast int
to char
in C# Using the ToString()
Method
Another approach to casting an int
to a char
involves using explicit conversion via the ToString()
method. While this method might seem less direct, it provides a way to convert an int
to a string and then extract the first character from that string.
The syntax for using explicit conversion via the ToString()
method involves converting the int
value to a string and then extracting the first character:
intValue.ToString()[0];
Here, intValue
is the int
variable you want to convert to a char
.
Let’s proceed with a complete working example to illustrate how explicit conversion via the ToString()
method is used to cast an int
to a char
in C#.
using System;
public class Program {
public static void Main() {
int intValue = 80;
char charValue = intValue.ToString()[0];
Console.WriteLine($"intValue.ToString()[0] is {charValue}");
}
}
In this example, we begin by declaring an int
variable named intValue
with a value of 80. The next line demonstrates explicit conversion via the ToString()
method, where intValue.ToString()[0]
converts the intValue
to a string and extracts the first character from that string, assigning it to the variable charValue
.
We use Console.WriteLine
to output the result.
Output:
intValue.ToString()[0] is 8
In this example, we successfully utilized explicit conversion via the ToString()
method to cast the int
value 80 to the char
in C#.
Conclusion
Casting an int
to a char
in C# can be achieved through various methods, each serving specific scenarios. The explicit type cast, Convert.ToChar()
method, Char.ConvertFromUtf32()
method, and explicit conversion via the ToString()
method offer different approaches, providing flexibility based on the requirements of the code.
Whether opting for simplicity with direct casts or considering specialized scenarios such as Unicode code points, C# developers have a range of techniques to choose from when converting int
to char
. Understanding these methods and their syntax allows programmers to make informed decisions tailored to the specific needs of their applications.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn