Equivalent of SQL Bigint in C#
-
Use the
BigInteger
Struct in C# as the Equivalent of SQLBIGINT
in C# -
Use
long
orint64
as the Equivalent of SQLBIGINT
in C# - Conclusion
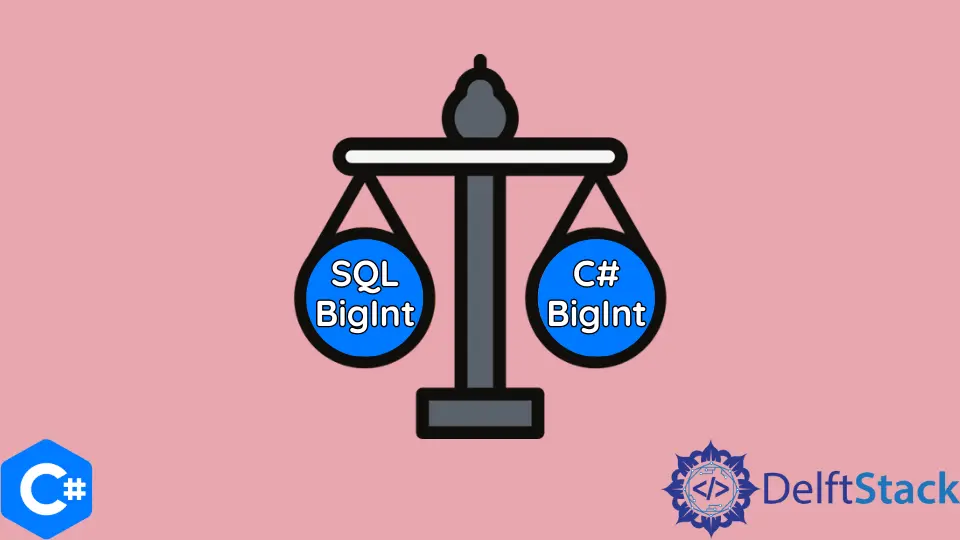
The SQL BIGINT
data type is a workhorse for storing large integer values. It can represent 64-bit signed integers and has a vast range, spanning from -2^63 (-9,223,372,036,854,775,808)
to 2^63 (9,223,372,036,854,775,807)
.
These expansive boundaries make it a popular choice in databases for storing everything from unique identifiers to large quantities. When working with SQL databases in C#, you might wonder how to handle these BIGINT
values, given that there isn’t a direct equivalent data type.
In this article, we’ll explore two primary methods for managing BIGINT
values in C#: the BigInteger
and the long
data types.
Use the BigInteger
Struct in C# as the Equivalent of SQL BIGINT
in C#
The BigInteger
is a data type that differentiates itself from the usual integral types in C#. What sets it apart is its capability to represent arbitrarily large signed integers, effectively making it an ideal candidate for seamlessly handling values akin to SQL’s BIGINT
.
The BigInteger
has no predefined upper or lower limits, which means it can accommodate numbers of any magnitude.
Let’s delve into the essential aspects of the BigInteger
:
Versatile Numerical Representation
The BigInteger
can handle both positive and negative numbers, as well as zero. It has a wide range, accommodating any integer value within the available memory.
This versatility makes it suitable for a wide array of applications, from cryptography to scientific computing.
Mathematical Operations
The BigInteger
operates similarly to other integral types in C#. It allows you to perform basic mathematical operations by overloading standard numeric operators.
This includes addition, subtraction, multiplication, and division, among others. These operations are intuitive and make working with large numbers straightforward.
Initialization
To declare a BigInteger
, you can use one of its constructors, such as new BigInteger(long)
, new BigInteger(ulong)
, or new BigInteger(byte[])
, which accepts an array of bytes. This means you can initialize a BigInteger
from different numeric data sources.
Let’s look at a practical example of how to use the BigInteger
data type in C#:
using System;
using System.Numerics;
public class BigIntegerExample {
public static void Main() {
// Declare a BigInteger
BigInteger bigIntValue = new BigInteger(1234567890123456789012345678901234567890);
// Perform arithmetic operations
BigInteger result = bigIntValue * 2;
Console.WriteLine("Original Value: " + bigIntValue);
Console.WriteLine("Result: " + result);
}
}
The code starts with two essential using
directives, importing the System
and System.Numerics
namespaces. These directives make the BigInteger
and Console
classes accessible for the program.
Following this, a class named BigIntegerExample
is defined, which encapsulates the code.
The core of the code lies in the declaration and manipulation of BigInteger
variables. A BigInteger
variable called bigIntValue
is declared and initialized with a colossal integer value, specifically 1234567890123456789012345678901234567890
.
This highlights how BigInteger
can effortlessly handle large numeric values. Additionally, the code showcases the simplicity of arithmetic operations with BigInteger
.
It multiplies bigIntValue
by 2
, resulting in a new BigInteger
variable named result
. The BigInteger
data type supports standard arithmetic operators, which makes working with vast numbers as straightforward as working with regular integers.
To conclude, the code wraps up by employing the Console.WriteLine
method to display the original value of bigIntValue
and the result of the multiplication operation in the console. The labels Original Value:
and Result:
are used for clarity and are concatenated with the respective BigInteger
values using the +
operator.
Use long
or int64
as the Equivalent of SQL BIGINT
in C#
When dealing with large integer values in C# that closely resembles SQL’s BIGINT
, the BigInteger
data type is not the only option available. For those who prefer a more direct and familiar alternative, the long
data type is a compelling choice.
A long
in C# is a 64-bit integer, occupying 8 bytes of memory, which closely aligns with the characteristics of a SQL BIGINT
. It can represent extremely large positive and negative integral numbers, and it falls within the range of -9,223,372,036,854,775,808
to 9,223,372,036,854,775,807
.
This range is sufficient for most practical applications, making long
an efficient choice for handling BIGINT
values in C#.
Here’s how you can utilize the long
data type to manage BIGINT
values in C#:
using System;
public class LongExample {
public static void Main() {
// Declare a long
long bigIntValue = 1234567890123456789;
// Perform arithmetic operations
long result = bigIntValue * 2;
Console.WriteLine("Original Value: " + bigIntValue);
Console.WriteLine("\nResult: " + result);
}
}
Output:
Original Value: 1234567890123456789
Result: 2469135780246913578
In this example, we declare a long
variable named bigIntValue
and initialize it with a substantial integer value, in this case, 1234567890123456789
. We then proceed to perform a multiplication operation by doubling this value and storing the result in a long
variable named result
.
Finally, we use the Console.WriteLine
method to output both the original value and the result to the console.
The choice of using long
to represent BIGINT
values in C# offers a familiar and efficient solution. This data type aligns closely with the SQL BIGINT
, and its range is adequate for most practical scenarios.
Whether you opt for the versatility of BigInteger
or the familiarity of long
, C# provides options to suit your needs when working with large integral numbers.
Conclusion
The choice between BigInteger
and long
depends on your specific needs and preferences. If you are dealing with numbers of truly astronomical magnitudes without any predefined limits, the BigInteger
offers boundless flexibility.
However, if your requirements fall within the range of a typical BIGINT
, and you prefer a more straightforward solution, long
provides a familiar and highly capable alternative.
Understanding the distinctions between these data types empowers you to effectively manage BIGINT
values from your SQL database in your C# applications, ensuring seamless integration and robust handling of large integer values. Whether you opt for the limitless capabilities of BigInteger
or the familiarity of long
, you’ll be well-equipped to tackle the world of large integers in C#.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub