How to Create a Generic Function in C#
-
Create a Generic Function With Function Overloading in
C#
-
Create a Generic Function With
T
Class inC#
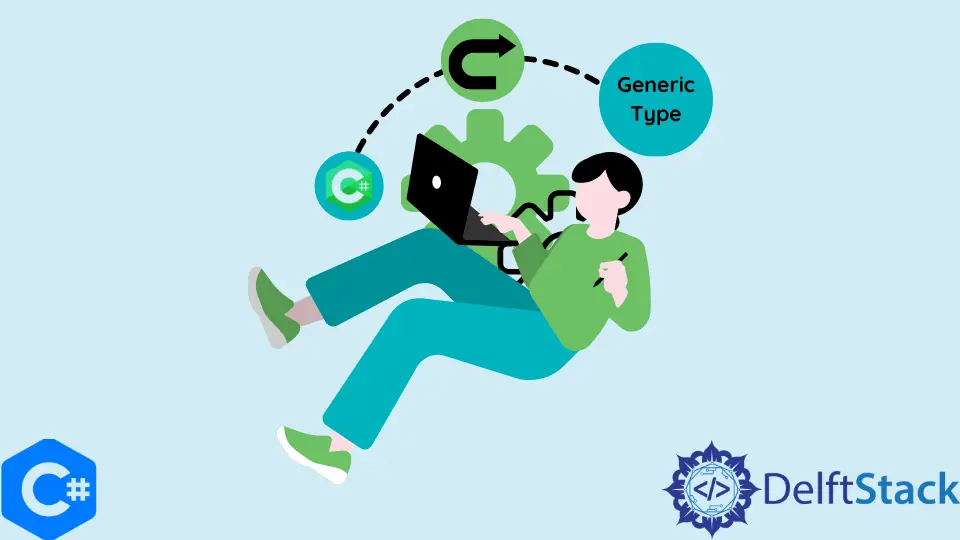
This tutorial will discuss the methods to create a function with a generic return type in C#.
Create a Generic Function With Function Overloading in C#
If we face a scenario where we need the same function to be applied to different data types, we can have multiple solutions for that problem.
Our first solution involves function overloading. In this, we create multiple functions with the same name but different return types and or different parameters.
The following code snippet demonstrates how we can achieve a generic function using function overloading in C#.
class myClass {
public int div(int a, int b) {
return a / b;
}
public double div(double a, double b) {
return a / b;
}
public static void Main(String[] args) {
myClass ob = new myClass();
int result1 = ob.div(12, 2);
Console.WriteLine(result1);
double result2 = ob.div(13, 2.5);
Console.WriteLine(result2);
}
}
Output:
6
5.2
We defined two different functions of the same name, div()
, which handle integer and decimal division in C#. Similarly, more functions can be written to handle more cases.
The advantage of this method is that we aren’t bound to do the same thing with all the data types. We can do one thing with one data type and do the opposite thing with the other data type.
It all depends on our scenario and needs.
The clear disadvantage of this approach is that we have to write a lot of code. This problem becomes apparent when we do the same thing but need to cover all the data types.
Create a Generic Function With T
Class in C#
This approach is the best in scenarios where we are doing the same thing to all the data types and need to handle many cases.
An example of this type of task is reading data from a database. Here, we know that the data types of one column are the same but have no idea about the data type or the column in advance.
In C#, a generic function is a function that is declared with a type parameter T
. This type parameter T
is used while calling the function to define the function type.
We can call the same function with different data types as type parameters each time.
The following code snippet demonstrates how we can use the type parameter to declare and use a function with a generic return type in C#.
static T changeType<T>(string v) {
return (T)Convert.ChangeType(v, typeof(T));
}
string s = "92";
int ci = changeType<int>(s);
float cf = changeType<float>(s);
decimal cd = changeType<decimal>(s);
Console.WriteLine(ci);
Console.WriteLine(cf);
Console.WriteLine(cd);
Output:
92
92
92
In the above code, we declared a function changeType<T>()
with the type parameter T
. This function takes a string variable v
as an input parameter, converts the data type of the input parameter v
to T
, casts the result into T
, and returns it.
The output shows what happens when we try to change the string 92
data type to integer, float, and decimal, respectively.
The problem with this approach is that if the string isn’t type-castable to the type parameter T
, it would give a runtime error.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn