How to Convert Int to Float in C#
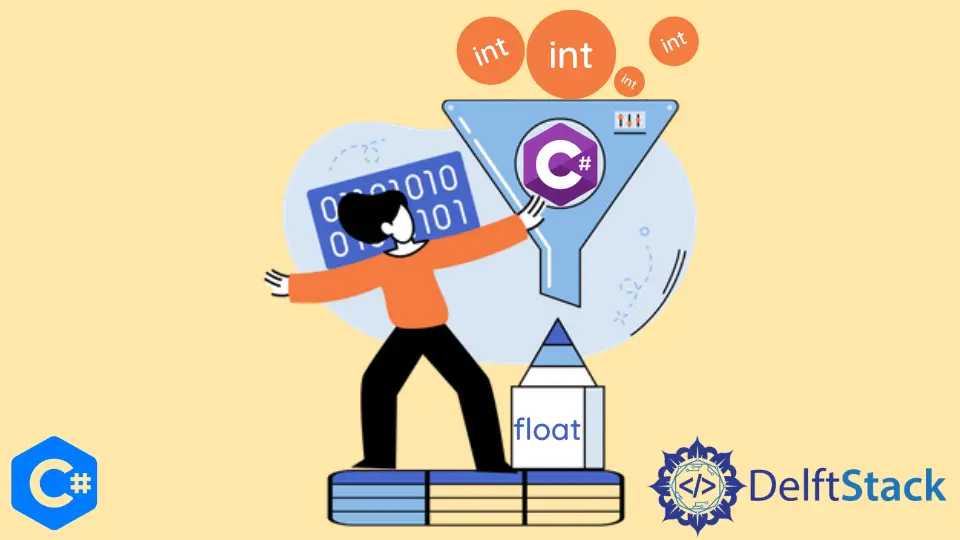
Converting an integer to a float in C# is a fundamental skill that every programmer should master. Whether you’re performing calculations that require decimal precision or simply need to handle data types effectively, understanding how to convert between these types is essential. In this guide, we will explore various methods to convert an int to a float in C#. The process is straightforward and can be accomplished through simple casting. We’ll walk you through the steps with clear code examples and detailed explanations. By the end of this article, you’ll have a solid grasp of how to perform this conversion seamlessly in your C# applications.
Method 1: Using Casting
Casting is one of the simplest ways to convert an integer to a float in C#. This method involves explicitly specifying the conversion you want to perform. Here’s how it works:
int integerValue = 42;
float floatValue = (float)integerValue;
Console.WriteLine(floatValue);
Output:
42
In this example, we start with an integer variable named integerValue
set to 42. By using the casting syntax (float)
, we convert integerValue
to a float and store it in floatValue
. The Console.WriteLine method then prints the value of floatValue
, which will display 42 as a floating-point number.
Casting is direct and efficient, making it a popular choice among developers. However, it’s important to note that casting from int to float can lead to loss of precision if you’re working with very large integers, as floats have a limited range and precision compared to integers. This method is best used when you’re confident that the integer value is within a range that won’t cause precision issues.
Method 2: Using Convert.ToSingle
Another reliable method for converting an integer to a float in C# is by using the Convert.ToSingle
method. This approach is particularly useful when you want to ensure that the conversion is safe and handles different data types gracefully.
int integerValue = 42;
float floatValue = Convert.ToSingle(integerValue);
Console.WriteLine(floatValue);
Output:
42
In this snippet, we again start with an integer variable, integerValue
, which is set to 42. By calling Convert.ToSingle(integerValue)
, we convert the integer to a float and assign it to floatValue
. The result is then printed to the console.
Using Convert.ToSingle
is advantageous because it can handle null values and other data types, returning 0 for nulls, which can help avoid exceptions in your code. This method is preferred in scenarios where you might be dealing with user input or data from external sources, as it provides a layer of safety and robustness in your application.
Method 3: Using Float.Parse
If you have an integer value in string format and you want to convert it to a float, you can use the float.Parse
method. This method is particularly beneficial when working with user input or data that comes from external sources, such as files or databases.
string integerString = "42";
float floatValue = float.Parse(integerString);
Console.WriteLine(floatValue);
Output:
42
In this example, we have a string variable integerString
that contains the value “42”. By using float.Parse(integerString)
, we convert the string representation of the integer to a float, which we then store in floatValue
. Finally, we print the value of floatValue
to the console.
It’s important to note that float.Parse
can throw exceptions if the string is not a valid number, so it’s a good practice to use it within a try-catch block or to validate the input beforehand. This method is ideal for scenarios where you’re dealing with user input or data that may not always be formatted correctly.
Conclusion
Converting an int to a float in C# can be done in several straightforward ways, including casting, using Convert.ToSingle
, and float.Parse
. Each method has its own advantages, and the right choice depends on your specific use case. By understanding these methods, you can ensure that your applications handle numerical data effectively and accurately. Whether you’re working with user input, performing calculations, or processing data, mastering these conversion techniques will enhance your programming skills and improve your code’s reliability.
FAQ
-
What is the difference between casting and using Convert.ToSingle?
Casting is a direct method to convert types, while Convert.ToSingle is safer and can handle different data types gracefully. -
Can I convert a float back to an int in C#?
Yes, you can convert a float back to an int using casting, but be aware that this will truncate the decimal part. -
What happens if I try to convert a string that is not a number using float.Parse?
It will throw a FormatException, so ensure the string is a valid number before parsing. -
Is there a performance difference between these conversion methods?
Generally, casting is the fastest, but Convert.ToSingle and float.Parse are safer for handling various input types.
- Can I use these conversion methods in a loop?
Absolutely! You can use any of these methods in loops for batch processing of data.
with this comprehensive guide. We’ll cover simple casting and other methods like Convert.ToSingle and float.Parse. With clear examples and detailed explanations, you’ll master the conversion process effortlessly. Perfect for beginners and experienced developers alike, this article will enhance your programming skills and improve your code’s reliability.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Csharp Int
- How to Convert a Char to an Int in C#
- How to Convert Char to Int in C#
- How to Convert Int to Byte in C#