How to Generate a Random Float in C#
-
Generate a Random Float Between
0
and1
With theRandom.NextDouble()
Function in C# -
Generate Random Float Without a Range With the
Random.NextDouble()
Function in C# -
Generate Random Float Within a Specific Range With the
Random.NextDouble()
Function in C# -
Generate Random Float With the
Random.NextBytes()
Function inC#
-
Generate Random Float Using the
Random.Next()
Function and Scaling in C# -
Generate Random Float Using
System.Random
Function in C# - Conclusion
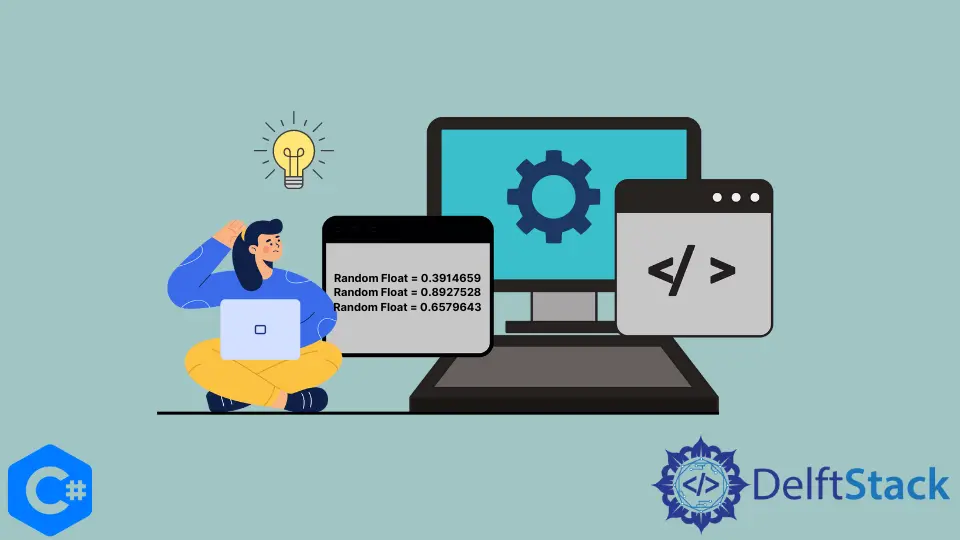
Generating random float values is a common task in programming. While C# does not provide a built-in method for generating random float values directly, we can utilize the Random
class and some mathematical techniques to achieve our goal.
In this tutorial, we will discuss methods to generate a random float value in C#.
Generate a Random Float Between 0
and 1
With the Random.NextDouble()
Function in C#
The Random.NextDouble()
function is used to generate a random double value between 0
and 1
. We can use the Random.NextDouble()
function to generate a random double value between 0
and 1
and then typecast that random value into a float.
Syntax:
Random random = new Random();
double randomNumber = random.NextDouble();
The Random.NextDouble()
does not take any parameters. It is a parameterless method that generates a random double-precision floating-point number between 0.0
(inclusive) and 1.0
(exclusive) using the current instance of the Random
class.
The following code example shows us how we can generate a random float value between 0
and 1
with the Random.NextDouble()
function.
using System;
namespace random_float {
class Program {
static void Main(string[] args) {
Random rand = new Random();
for (int i = 0; i < 10; i++) {
float randomFloat = (float)rand.NextDouble();
Console.WriteLine("Random Float = {0}", randomFloat);
}
}
}
}
Output:
Random Float = 0.3914659
Random Float = 0.8927528
Random Float = 0.6579643
Random Float = 0.4404075
Random Float = 0.3213561
Random Float = 0.3399849
Random Float = 0.04562188
Random Float = 0.1881405
Random Float = 0.7638124
Random Float = 0.1645804
In the code above, we initialized an instance rand
to generate random numbers. Then, inside a for
loop, it repeatedly generates a random double-precision floating-point number and converts it to a float, storing it in randomFloat
.
Each generated randomFloat
is then printed to the console. This process is repeated ten times, resulting in ten distinct random floating-point values being displayed on each program run.
Generate Random Float Without a Range With the Random.NextDouble()
Function in C#
We can also generate a random float value without setting the range between 0
and 1
with the Random.NextDouble()
function in C#. The only range in this method is the range of values that the float data type can hold in it.
We can use float.MaxValue
and float.MinValue
to specify the maximum and minimum values that the float data type can store. The following code example shows us how to generate a random float value without specifying a range with the Random.NextDouble()
function.
using System;
namespace random_float {
class Program {
static void Main(string[] args) {
Random rand = new Random();
double range = (double)float.MaxValue - (double)float.MinValue;
for (int i = 0; i < 10; i++) {
double sample = rand.NextDouble();
double scaled = (sample * range) + float.MinValue;
float f = (float)scaled;
Console.WriteLine(f);
}
}
}
}
Output:
1.500952E+38
-1.930891E+38
2.951987E+38
-1.205054E+38
-6.225039E+37
3.843309E+36
-1.241292E+38
1.360907E+38
1.769061E+38
-1.577001E+38
In the code above, we generate a random float value without a range with the Random.NextDouble()
function.
First, we initialized the double variable range
with float.MaxValue - float.MinValue
and then typecasting it into double data type. This ensures that the random value range stays within the range of values that a float data type can handle.
We calculated the random value by multiplying the value returned by the Random.NextDouble()
function with the range
and adding float.MinValue
to the result.
In the end, we converted the random value into float data type with typecasting.
Generate Random Float Within a Specific Range With the Random.NextDouble()
Function in C#
We can also generate a random value within a specific range with the Random.NextDouble()
function in C#.
For example, suppose we have to generate a random float value between 1
and 10
. In that case, we can generate a random value between 0
and 1
with the Random.NextDouble()
function, multiply it with the range, and then add the minimum value to it.
The following code example shows us how to generate a random number between a range of values with the Random.NextDouble()
function.
using System;
namespace random_float {
class Program {
static void Main(string[] args) {
Random rand = new Random();
double min = 1;
double max = 10;
double range = max - min;
for (int i = 0; i < 10; i++) {
double sample = rand.NextDouble();
double scaled = (sample * range) + min;
float f = (float)scaled;
Console.WriteLine(f);
}
}
}
}
Output:
3.468961
8.04868
2.210197
8.150612
4.217263
5.328617
4.730082
8.462176
2.679844
8.609394
In the code above, we generate a random float value between 1
and 10
with the Random.NextDouble()
function.
First, we specified the min
and max
values and calculated the range with range = max - min
. Then, we calculated the random value by multiplying the value returned by the Random.NextDouble()
function with the range
and adding min
to the result.
Lastly, we converted the random value into float data type with typecasting.
Generate Random Float With the Random.NextBytes()
Function in C#
We can also generate a random float value with the Random.NextBytes()
function in C#. The Random.NextBytes(byte[])
function is used to fill the byte[]
array with random byte values.
We can generate random float values by converting the bytes stored in byte[]
to float datatype.
Syntax:
Random random = new Random();
byte[] buffer = new byte[numberOfBytes];
random.NextBytes(buffer);
Parameters:
buffer
: Thebuffer
is a byte array that serves as the destination for the generated random bytes. The method will fill this array (buffer
) with random values.
The following code example shows us how we can generate a random float value with the Random.NextBytes()
function.
using System;
namespace random_float {
class Program {
static void Main(string[] args) {
Random rand = new Random();
for (int i = 0; i < 10; i++) {
var array = new byte[4];
rand.NextBytes(array);
float randomFloat = BitConverter.ToSingle(array, 0);
Console.WriteLine("Random Value = {0}", randomFloat);
}
}
}
}
Output:
Random Value = 2.021232E-20
Random Value = -121623
Random Value = -1.564382E+38
Random Value = 1.146448E-14
Random Value = -6.874067E-22
Random Value = -1.226989E+31
Random Value = -3.01435E+08
Random Value = -8.568415E+30
Random Value = -1.454825E+27
Random Value = 3.801608E-26
In the code, we generated a random float value with the Random.NextBytes()
function in C#.
First, we use the Random
class to generate random bytes and then convert these bytes into floating-point values. In each iteration of the for
loop, a byte array of length 4
is created and filled with random bytes using rand.NextBytes(array)
.
Then, the BitConverter.ToSingle(array, 0)
method is employed to convert these bytes into a floating-point number, which is then printed to the console. The output consists of ten distinct random floating-point values on each program run due to the random byte generation.
Generate Random Float Using the Random.Next()
Function and Scaling in C#
Instead of relying solely on Random.NextDouble()
, we can use Random.Next()
to generate random integers within a specified range and then scale those integers to the desired float range.
Syntax:
int Random.Next();
The Random.Next()
does not take any parameters.
The following code example shows us how we can generate a random float value using the Random.Next()
function and Scaling.
using System;
namespace random_float {
class Program {
static void Main(string[] args) {
Random rand = new Random();
for (int i = 0; i < 10; i++) {
float randomFloat = (float)(rand.Next(0, 10000) / 10000.0);
Console.WriteLine("Random Float = {0}", randomFloat);
}
}
}
}
Output:
Random Float = 0.156
Random Float = 0.9271
Random Float = 0.8455
Random Float = 0.2892
Random Float = 0.7208
Random Float = 0.4402
Random Float = 0.3794
Random Float = 0.8931
Random Float = 0.8759
Random Float = 0.9866
In the code above, we initialize a Random
object called rand
to facilitate random number generation. Within a for
loop that iterates ten times, it generates random integer values using rand.Next(0, 10000)
.
In order to convert the random integers into float values within the 0
to 1
range, each integer is divided by 10000.0
. These scaled float values are stored in the randomFloat
variable and are subsequently displayed on the console.
Generate Random Float Using System.Random
Function in C#
We can also use the System.Random
class instead of the default Random
class. The System.Random
provides better randomness and can be used similarly to generate random float values.
Syntax:
System.Random random = new System.Random();
This method doesn’t have parameters. The default constructor System.Random()
is used, which initializes the random number generator with a seed value derived from the current system time.
After we create the System.Random
instance, we can use its methods, such as Next()
, to generate random integers. The following code example shows us how we can generate a random float value using the System.Random
function.
using System;
namespace random_float {
class Program {
static void Main(string[] args) {
System.Random rand = new System.Random();
for (int i = 0; i < 10; i++) {
float randomFloat = (float)(rand.Next(0, 10000) / 10000.0);
Console.WriteLine("Random Float = {0}", randomFloat);
}
}
}
}
Output:
Random Float = 0.1235
Random Float = 0.7891
Random Float = 0.4567
Random Float = 0.9876
Random Float = 0.6543
Random Float = 0.2345
Random Float = 0.9876
Random Float = 0.7654
Random Float = 0.5432
Random Float = 0.1111
In the code above, we create an instance of the System.Random
class named rand
is created to ensure better randomness in number generation. Within a for
loop that iterates ten times, random integer values are generated using rand.Next(0, 10000)
.
These integers are then divided by 10000.0
to convert them into float values within the 0
to 1
range. These resulting float values are stored in the randomFloat
variable and are subsequently displayed on the console, each preceded by the label "Random Float = "
.
Conclusion
Generating random float values in C# is achievable through different methods, offering flexibility based on our needs. Whether we need random float values within a specific range or simply within the constraints of the float data type, C# provides versatile options.
By utilizing the Random
class and mathematical conversions, we can effectively introduce randomness into our C# applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn