C# Random Bool
-
Generate a Random Boolean in C# Using the
Next()
Method FromRandom
Class -
Generate a Random Boolean in C# Using the
NextDouble()
Method FromRandom
Class -
Generate a Random Boolean in C# Using the
Guid.NewGuid()
Method -
Generate a Random Boolean in C# Using the
Environment.TickCount
Property - Conclusion
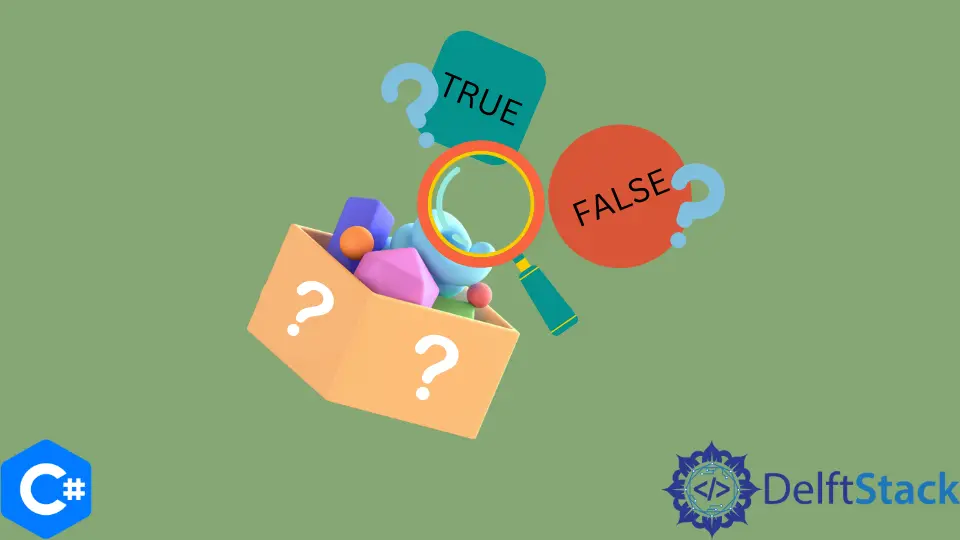
Have you ever found yourself in a scenario where you needed to programmatically flip a coin or distribute items randomly between two databases? The solution you’re seeking lies in what we call a random boolean—a variable that can hold only two values: true
or false
.
While the standard method involves using the Random
class, there are alternative and unconventional ways to generate a random boolean value in C#. In this article, we will explore four distinct methods: using the Next()
method from the Random
class, the NextDouble()
method, Guid.NewGuid()
, and Environment.TickCount
.
Generate a Random Boolean in C# Using the Next()
Method From Random
Class
In C#, generating a random boolean value can be achieved using the Next()
method from the Random
class. The Random
class provides methods to generate random numbers of different data types, and by utilizing the Next()
method, we can easily create a random boolean value.
Syntax:
Random random = new Random();
bool randomBoolean = random.Next(2) == 0;
In this syntax:
Random random = new Random();
creates an instance of theRandom
class.random.Next(2)
generates a random integer, either0
or1
.randomBoolean = random.Next(2) == 0;
assignstrue
if the randomly generated integer is0
, andfalse
otherwise.
Code example:
using System;
class Program {
static void Main() {
Random random = new Random();
bool randomBoolean = random.Next(2) == 0;
Console.WriteLine($"Random Boolean: {randomBoolean}");
}
}
We start by creating an instance of the Random
class using Random random = new Random();
, which is then used to generate a random integer using the Next(2)
method. The Next(2)
method produces a random integer that can be 0
or 1
.
We assign the result of random.Next(2) == 0
to the randomBoolean
variable. This expression evaluates to true
if the randomly generated integer is 0
and false
if it’s 1
.
Finally, we display the generated random Boolean using Console.WriteLine($"Random Boolean: {randomBoolean}");
.
The output of the code will be a line stating the value of the generated random Boolean, which will either be true
or false
:
Random Boolean: True
Note: Each time you run the program, the output may vary as it depends on the randomly generated boolean value.
Generate a Random Boolean in C# Using the NextDouble()
Method From Random
Class
In addition to using the Next()
method, another way to generate a random boolean value in C# is by using the NextDouble()
method from the Random
class. This method returns a random floating-point number greater than or equal to 0.0
and less than 1.0
.
By checking whether this value is less than 0.5
, we can determine a boolean outcome.
Syntax:
Random random = new Random();
bool randomBoolean = random.NextDouble() < 0.5;
Where:
Random random = new Random();
creates an instance of theRandom
class.random.NextDouble()
generates a random floating-point number in the range[0.0, 1.0)
.randomBoolean = random.NextDouble() < 0.5;
assignstrue
if the generated number is less than0.5
, andfalse
otherwise.
Code Example:
using System;
class Program {
static void Main() {
Random random = new Random();
bool randomBoolean = random.NextDouble() < 0.5;
Console.WriteLine($"Random Boolean: {randomBoolean}");
}
}
Similar to the previous example, we start by creating an instance of the Random
class with Random random = new Random();
. The NextDouble()
method is then used to obtain a random floating-point number in the range [0.0, 1.0)
.
We assign the result of random.NextDouble() < 0.5
to the randomBoolean
variable. If the generated floating-point number is less than 0.5
, the expression evaluates to true
; otherwise, it evaluates to false
.
Finally, we display the generated random Boolean using Console.WriteLine($"Random Boolean: {randomBoolean}");
.
Output:
Random Boolean: False
Generate a Random Boolean in C# Using the Guid.NewGuid()
Method
Another unconventional yet effective way to generate a random boolean value is by utilizing the Guid.NewGuid()
method. A Guid
(Globally Unique Identifier) is a 128-bit integer often used for unique identification.
By comparing the least significant bit of a randomly generated Guid
with 1
, we can determine a boolean outcome.
Syntax:
bool randomBoolean = (Guid.NewGuid().ToByteArray()[15] & 1) == 1;
In this syntax:
Guid.NewGuid()
generates a new unique identifier of typeGuid
.ToByteArray()
converts theGuid
to a byte array.ToByteArray()[15]
accesses the least significant byte of theGuid
.(ToByteArray()[15] & 1) == 1
checks if the least significant bit is set, resulting in eithertrue
orfalse
.
Code Example:
using System;
class Program {
static void Main() {
bool randomBoolean = (Guid.NewGuid().ToByteArray()[15] & 1) == 1;
Console.WriteLine($"Random Boolean: {randomBoolean}");
}
}
The code begins by generating a new Guid
using Guid.NewGuid()
. We then convert this Guid
to a byte array using ToByteArray()
.
The least significant byte, which resides at index 15
in the array, is accessed with ToByteArray()[15]
.
By performing a bitwise AND
operation with 1
(& 1
), we isolate the least significant bit. The expression (ToByteArray()[15] & 1) == 1
checks if this bit is set, resulting in either true
or false
.
Finally, we display the generated random Boolean using Console.WriteLine($"Random Boolean: {randomBoolean}");
.
Output:
Random Boolean: True
Generate a Random Boolean in C# Using the Environment.TickCount
Property
An alternative method for generating a random boolean involves utilizing the Environment.TickCount
property. This property returns the number of milliseconds that have elapsed since the system started.
By examining the least significant bit of the tick count, we can determine a boolean outcome.
Syntax:
bool randomBoolean = (Environment.TickCount & 1) == 1;
In this syntax:
Environment.TickCount
retrieves the number of milliseconds elapsed since the system started.(Environment.TickCount & 1)
performs a bitwiseAND
operation to isolate the least significant bit.(Environment.TickCount & 1) == 1
checks if the least significant bit is set, resulting in eithertrue
orfalse
.
Code Example:
using System;
class Program {
static void Main() {
bool randomBoolean = (Environment.TickCount & 1) == 1;
Console.WriteLine($"Random Boolean: {randomBoolean}");
}
}
The code starts by retrieving the number of milliseconds elapsed since the system started using Environment.TickCount
. We then perform a bitwise AND
operation with 1
(& 1
), isolating the least significant bit of the tick count.
The expression (Environment.TickCount & 1) == 1
checks whether this bit is set, resulting in either true
or false
. Finally, we display the generated random Boolean using Console.WriteLine($"Random Boolean: {randomBoolean}");
.
Output:
Random Boolean: False
Conclusion
Each of these methods offers a distinct way to generate random boolean values in C#. The choice of method may depend on the specific requirements of your application.
Whether you prefer the simplicity of the Random
class, the uniqueness of Guid
, or the system-dependent Environment.TickCount
, these unconventional approaches provide diverse options for introducing randomness into your code.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn