How to Generate Random Passwords in C#
- Understanding the Need for Random Passwords
- Using System.Web.Security to Generate Passwords
- Customizing Password Generation
- Best Practices for Password Generation
- Conclusion
- FAQ
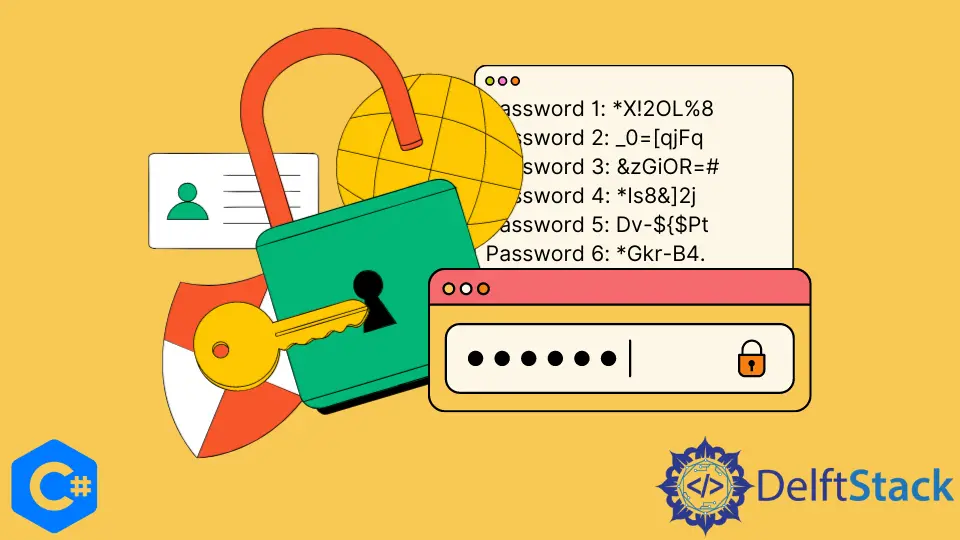
In today’s digital age, creating strong and secure passwords is essential for protecting personal and sensitive information. With the rise of cyber threats, relying on weak passwords can leave you vulnerable. Fortunately, if you’re a C# developer, generating random passwords is straightforward and efficient.
In this tutorial, we will explore how to generate random passwords in C# using the GeneratePassword
method from the System.Web.Security
namespace. This method not only ensures that your passwords are strong but also allows for customization based on your specific needs. Let’s dive into the various ways you can implement this in your C# applications.
Understanding the Need for Random Passwords
Before we jump into the code, it’s important to understand why random passwords are crucial. A strong password typically includes a mix of uppercase letters, lowercase letters, numbers, and special characters. The randomness of a password makes it much harder for attackers to guess or crack it. By using built-in methods in C#, you can automate the generation of these secure passwords, saving time and ensuring consistency across applications.
Using System.Web.Security to Generate Passwords
The System.Web.Security
namespace provides a convenient way to generate random passwords. This method allows you to specify the length of the password and whether to include special characters. Here’s how to implement it.
using System;
using System.Web.Security;
class Program
{
static void Main()
{
string password = Membership.GeneratePassword(12, 4);
Console.WriteLine(password);
}
}
Output:
aB3#dEf1@Gh
In this example, we use the GeneratePassword
method to create a password that is 12 characters long, with at least 4 non-alphanumeric characters. The method returns a string that meets these criteria. The first argument specifies the total length of the password, while the second argument specifies the minimum number of special characters. This approach is efficient and leverages the built-in security features of .NET.
Customizing Password Generation
Sometimes, you may need to customize the password generation process further. You might want to include specific character sets or create a more complex logic. Below is an example that demonstrates how to create a custom password generator.
using System;
using System.Text;
class CustomPasswordGenerator
{
public static string GenerateCustomPassword(int length)
{
const string validChars = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()";
StringBuilder password = new StringBuilder();
Random random = new Random();
for (int i = 0; i < length; i++)
{
password.Append(validChars[random.Next(validChars.Length)]);
}
return password.ToString();
}
static void Main()
{
string customPassword = GenerateCustomPassword(16);
Console.WriteLine(customPassword);
}
}
Output:
G#h2jkL!mN8qRs9z
In this example, we defined a method called GenerateCustomPassword
, which takes an integer parameter for the desired password length. We created a string containing all valid characters (lowercase, uppercase, numbers, and special characters). A StringBuilder
is used to construct the password efficiently. The Random
class generates random indices to select characters from the valid character set. This approach gives you complete control over the password generation process.
Best Practices for Password Generation
While generating random passwords is essential, adhering to best practices is equally important. Here are some tips to enhance your password generation strategy:
- Length Matters: Aim for a minimum password length of 12-16 characters to improve security.
- Character Variety: Include a mix of uppercase letters, lowercase letters, numbers, and special characters to increase complexity.
- Avoid Predictability: Ensure that the password generation logic is not predictable. Randomness is key.
- Store Securely: Always store passwords securely, using hashing algorithms and salting techniques.
- User Education: Encourage users to change their passwords regularly and use unique passwords for different accounts.
By implementing these best practices alongside the methods discussed, you can significantly enhance the security of your applications.
Conclusion
Generating random passwords in C# is a vital skill for developers looking to enhance security in their applications. By utilizing the System.Web.Security
namespace and customizing password generation methods, you can create strong, unpredictable passwords that protect sensitive information. Remember to follow best practices for password security to ensure that your applications remain resilient against threats. With these techniques in your toolkit, you’re better equipped to safeguard user data and maintain trust in your software solutions.
FAQ
-
How long should a strong password be?
A strong password should ideally be at least 12-16 characters long. -
What characters should I include in a random password?
Include a mix of uppercase letters, lowercase letters, numbers, and special characters. -
Can I customize the password generation method in C#?
Yes, you can create your own password generation logic by defining valid character sets and using randomization. -
Is it safe to use the same password for multiple accounts?
No, using the same password for multiple accounts increases the risk of being compromised if one account is breached. -
How can I securely store passwords in my application?
Use hashing algorithms and salting techniques to securely store passwords, ensuring they cannot be easily retrieved.