How to Efficiently Remove All Whitespaces From a String in C#
-
C# Program to Efficiently Remove All Whitespaces From a
String
UsingRegex.Replace()
Method -
C# Program to Efficiently Remove All Whitespaces From a
String
UsingString.Replace()
Method -
C# Program to Efficiently Remove All Whitespaces From a
String
UsingWhere
Method
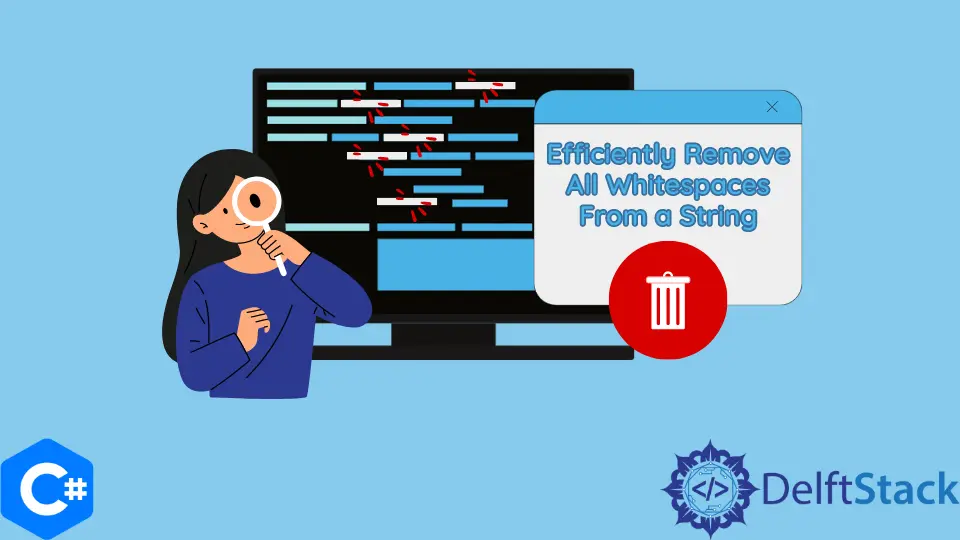
In C#, there are different methods to efficiently remove all whitespaces from a string
.
In this article, we are going to discuss various methods to efficiently remove all whitespaces from a string
.
C# Program to Efficiently Remove All Whitespaces From a String
Using Regex.Replace()
Method
Regular expression is the most efficient pattern matching feature in C#. It has a specific pattern for every operation. By using regular expressions, we can also remove all whitespaces from a string. We will use the Regex.Replace()
method to efficiently remove all whitespaces. It belongs to the Regex class in C#.
The correct syntax to use this method is as follows:
Regex.Replace(String, @"\s", "")
Here ""
represents an empty string
. The regular expression pattern for a whitespace character is \s
. The Regex.Replace()
method finds a whitespace character
in the given string
and replaces it with an empty string
.
Example Code:
using System;
using System.Text.RegularExpressions;
namespace Example {
class RemoveAllWhitespaces {
static void Main(string[] args) {
string OldString = "This is a String.";
Console.WriteLine("The old string is: " + OldString);
string NewString = Regex.Replace(OldString, @"\s", "");
Console.WriteLine("The new string is: " + NewString);
}
}
}
Output:
The old string is: This is a String.
The new string is: ThisisaString.
C# Program to Efficiently Remove All Whitespaces From a String
Using String.Replace()
Method
This is the simplest method to remove whitespaces from a given string
. The method Replace()
replaces a given string
or character
with the desired string
or character
.
The correct syntax to use this method is as follows:
String.Replace(" ", String.Empty);
Here String.Empty
represents an empty string
. This method finds whitespaces in a string
and replaces it with an empty string
.
Example Code:
using System;
namespace Example {
class RemoveWhitespaces {
static void Main(string[] args) {
string String1 = "This is a String.";
String1 = String1.Replace(" ", String.Empty);
Console.WriteLine(String1);
}
}
}
Output:
The old string is: This is a String.
The new string is: ThisisaString.
" "
but not other white spaces like tab (\t
) or newline (\n
).C# Program to Efficiently Remove All Whitespaces From a String
Using Where
Method
The method Where
is a LINQ class method. It is used to perform various useful operations. We have used it here to remove all the whitespaces from a string
.
The correct syntax to use this method to remove all whitespaces is as follows:
String.Concat(OldString.Where(c => !Char.IsWhiteSpace(c)));
Here, we have used Where
to find all the non-whitespaces of the string
with the help of IsWhiteSpace()
method. We have checked the string
character by character. We have then used the method String.Concat
to join all these characters
to form the string
again.
Example Code:
using System;
using System.Linq;
namespace Example {
class RemoveAllWhitespaces {
static void Main(string[] args) {
string OldString = "This is a String.";
Console.WriteLine("The old string is: " + OldString);
string NewString = String.Concat(OldString.Where(c => !Char.IsWhiteSpace(c)));
Console.WriteLine("The new string is: " + NewString);
}
}
}
Output:
The old string is: This is a String.
The new string is: ThisisaString.