C# 有效地删除字符串中的所有空格
Minahil Noor
2024年2月16日
Csharp
Csharp String
-
C# 使用
Regex.Replace()
方法有效地删除字符串中的所有空格 -
C# 使用
String.Replace()
方法有效地从字符串中删除所有空格 -
C# 使用
Where
方法有效地删除字符串中的所有空格
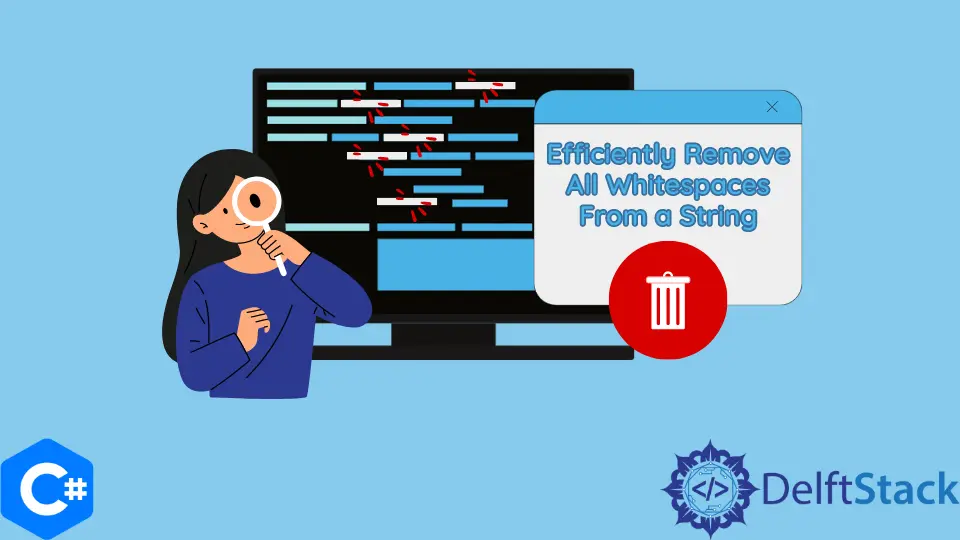
在 C# 中,有多种方法可以有效地删除字符串中的所有空格。在本文中,我们将讨论各种方法来删除字符串中的所有空格。
C# 使用 Regex.Replace()
方法有效地删除字符串中的所有空格
正则表达式是 C# 中最有效的模式匹配功能。每个操作都有特定的模式。通过使用正则表达式,我们还可以从字符串中删除所有空格。我们将使用 Regex.Replace()
方法有效地删除所有空格。它属于 C# 中的 Regex
类。
使用此方法的正确语法如下:
Regex.Replace(String, @"\s", "")
这里的 ""
代表一个空的字符串。空格字符的正则表达式模式为\s
。Regex.Replace()
方法在给定的字符串中找到一个空格字符,并将其替换为空的字符串。
示例代码:
using System;
using System.Text.RegularExpressions;
namespace Example {
class RemoveAllWhitespaces {
static void Main(string[] args) {
string OldString = "This is a String.";
Console.WriteLine("The old string is: " + OldString);
string NewString = Regex.Replace(OldString, @"\s", "");
Console.WriteLine("The new string is: " + NewString);
}
}
}
输出:
The old string is: This is a String.
The new string is: ThisisaString.
C# 使用 String.Replace()
方法有效地从字符串中删除所有空格
这是从给定的字符串中删除空格的最简单方法。方法 Replace()
将给定的字符串或 character
替换为所需的字符串或字符。
使用此方法的正确语法如下:
String.Replace(" ", String.Empty);
这里的 String.Empty
代表一个空的字符串。该方法在字符串中查找空格,并将其替换为空的字符串。
示例代码:
using System;
namespace Example {
class RemoveWhitespaces {
static void Main(string[] args) {
string String1 = "This is a String.";
String1 = String1.Replace(" ", String.Empty);
Console.WriteLine(String1);
}
}
}
输出:
The old string is: This is a String.
The new string is: ThisisaString.
注意
此方法只能删除单个空格字符
" "
,而不能删除其他空白,例如制表符(\t
)或换行符(\n
)。C# 使用 Where
方法有效地删除字符串中的所有空格
方法 Where
是一个 LINQ
类方法。它用于执行各种有用的操作。我们在这里使用它来删除字符串中的所有空格。
使用此方法删除所有空格的正确语法如下:
String.Concat(OldString.Where(c => !Char.IsWhiteSpace(c)));
在这里,我们使用 Where
在 IsWhiteSpace()
方法的帮助下找到字符串的所有非空白。我们逐字符检查了字符串字符,然后用 String.Concat
方法来连接所有这些字符以再次形成字符串。
示例代码:
using System;
using System.Linq;
namespace Example {
class RemoveAllWhitespaces {
static void Main(string[] args) {
string OldString = "This is a String.";
Console.WriteLine("The old string is: " + OldString);
string NewString = String.Concat(OldString.Where(c => !Char.IsWhiteSpace(c)));
Console.WriteLine("The new string is: " + NewString);
}
}
}
输出:
The old string is: This is a String.
The new string is: ThisisaString.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe